Behavior-Driven Development (BDD) is an agile software development process that encourages collaboration between developers, testers, and non-technical or business participants in a software project. BDD extends Test-Driven Development (TDD) by writing tests in a natural language that non-programmers can read. This practice ensures that everyone understands what the software should do.
On This Page
Table of Contents
The Benefits of BDD
Implementing BDD in your development process has several benefits:
- Improved Communication: BDD fosters better communication among team members by using a common language. 📢
- Early Detection of Defects: Identifying issues early in the development cycle reduces the cost and effort required to fix them. 🔍
- Enhanced Documentation: Since BDD scenarios are written in plain language, they serve as excellent documentation for the project. 📝
- Increased Test Coverage: BDD encourages comprehensive testing of all scenarios, leading to more robust software. 🛡️
BDD vs. TDD
Both BDD and TDD are essential methodologies in the software development lifecycle, but they have distinct differences:
Aspect | BDD | TDD |
---|---|---|
Focus | Behavior (User-centric) | Functionality (Code-centric) |
Test Format | Natural language (Gherkin) | Programming language (Unit tests) |
Collaboration | Involves all stakeholders | Mainly developers |
Goal | Ensure software meets user expectations | Ensure code correctness and reliability |
Example | In BDD, the focus is on the behavior. You would start by writing a feature file in Gherkin syntax: This scenario describes the expected behavior in a readable format. The next step would be to implement the step definitions in code to make this scenario pass. | Suppose you need to develop a function that adds two numbers. In TDD, you would start by writing a unit test:
This test will initially fail because the `add` method is not yet implemented. Next, you would write the code to pass the test: |
What is Cucumber?
Cucumber is a popular tool used in Behavior-Driven Development (BDD) to facilitate collaboration between developers, testers, and business stakeholders. It allows writing tests in a human-readable format, making it easier for everyone involved to understand the requirements and the test scenarios.
Key Features of Cucumber
Cucumber offers several features that make it a preferred choice for BDD:
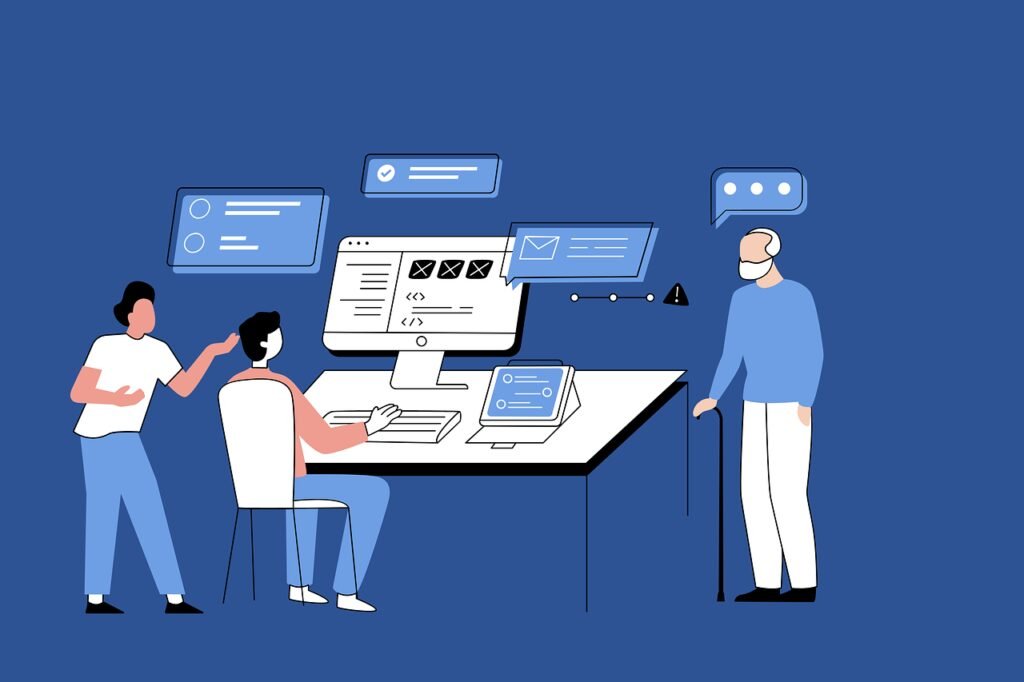
- Readable Syntax: Uses Gherkin language, which is simple and easily understandable by non-programmers.
- Reusable Code: Encourages the reuse of test steps, reducing duplication and maintenance efforts.
- Integrations: Supports integration with various testing frameworks and CI/CD tools.
- Multi-language Support: Compatible with multiple programming languages like Java, Ruby, and JavaScript.
Cucumber vs. Other BDD Tools
While there are several BDD tools available, Cucumber stands out for several reasons:
Feature | Cucumber | Other BDD Tools |
---|---|---|
Syntax | Gherkin | Varies (e.g., SpecFlow, Behat) |
Community Support | Strong, active community | Depends on the tool |
Integration | Wide range of tools | Limited options |
Ease of Use | High | Varies |
Example
Imagine a banking application where you want to ensure that users can transfer money between accounts. A Cucumber scenario for this might look like:
Feature: Money Transfer
Scenario: Transfer money between two accounts
Given the user has an account with a balance of $1000
When the user transfers $200 to another account
Then the user's account balance should be $800
This simple example illustrates how readable and straightforward Cucumber scenarios can be, allowing all stakeholders to easily understand the tests.
Installation and Setup 🚀
Before diving into the installation of Cucumber, it’s crucial to ensure that you have a few prerequisites in place. Firstly, Cucumber requires Java to be installed on your system. If you don’t already have it, you can download it from the official Java SE Development Kit. Additionally, Maven is essential for managing your project’s dependencies. You can install Maven from the official website.
With these prerequisites in place, let’s proceed with the installation of Cucumber. The following commands assume you have a terminal or command-line interface open:
Step-by-Step Installation
1. Verify Java Installation:
java -version
This command should return the Java version installed on your machine. If not, ensure Java is correctly installed and configured.
2. Verify Maven Installation:
mvn -version
Like Java, this command should display the Maven version. If it doesn’t, check your Maven installation.
3. Set Up a New Maven Project:
mvn archetype:generate -DgroupId=com.example -DartifactId=cucumber-example -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
This command initializes a new Maven project, setting up the basic structure needed for Cucumber.
4. Add Cucumber Dependencies: Open the `pom.xml` file in your project and add the following dependencies:
<dependencies>
<dependency>
<groupId>io.cucumber</groupId>
<artifactId>cucumber-java</artifactId>
<version>6.9.1</version>
</dependency>
<dependency>
<groupId>io.cucumber</groupId>
<artifactId>cucumber-junit</artifactId>
<version>6.9.1</version>
</dependency>
</dependencies>
Save the file and run `mvn install` to download the Cucumber dependencies.
Troubleshooting Tips
If you encounter issues during installation, here are some common problems and their solutions:
1. Java Not Recognized: Ensure that your JAVA_HOME environment variable is set correctly.
2. Maven Commands Not Found: Verify that MAVEN_HOME is set and that the `bin` directory is added to your PATH.
3. Dependency Resolution Issues: Double-check the `pom.xml` file for typos or incorrect versions of dependencies.

Configuring Cucumber for Your Project
Integrating Cucumber into your existing projects can significantly enhance your testing framework by promoting behavior-driven development (BDD). The process involves setting up feature files, creating step definitions, and ensuring that all components work seamlessly together.
Creating Feature Files
Feature files are the cornerstone of Cucumber-based testing frameworks. They describe the application’s behavior in plain language, making it accessible to both technical and non-technical stakeholders. Here’s a simple example of a feature file:
Feature: User Login
Scenario: Successful Login
Given the user is on the login page
When the user enters valid credentials
Then the user is redirected to the dashboard
Each line starting with Given, When, and Then represents a step in the scenario. These steps need corresponding step definitions in the code.
Creating Step Definitions
Step definitions are written in the programming language supported by your project, such as Java, JavaScript, or Ruby. Below is an example of step definitions in Java:
import io.cucumber.java.en.Given;
import io.cucumber.java.en.When;
import io.cucumber.java.en.Then;
public class LoginSteps {
@Given("the user is on the login page")
public void userOnLoginPage() {
// Code to navigate to the login page
}
@When("the user enters valid credentials")
public void userEntersValidCredentials() {
// Code to enter username and password
}
@Then("the user is redirected to the dashboard")
public void userRedirectedToDashboard() {
// Code to verify redirection to the dashboard
}
}
Integrating Cucumber with Your Development Environment 🛠️
Integrating Cucumber with your development environment is a crucial step in leveraging its full potential for behavior-driven development (BDD). Popular IDEs like IntelliJ IDEA, Eclipse, and Visual Studio Code offer robust support for Cucumber, facilitating seamless setup and execution of tests. Here, we provide detailed instructions to get you started with each of these environments.
IntelliJ IDEA
First, ensure you have the necessary plugins installed. Navigate to File > Settings > Plugins, and search for “Cucumber for Java“. Install the plugin and restart the IDE. Next, set up a new project or open an existing one. Add Cucumber dependencies in your pom.xml
if you’re using Maven:
<dependency>
<groupId>io.cucumber</groupId>
<artifactId>cucumber-java</artifactId>
<version>6.9.1</version>
</dependency>
To run your tests, create a new Run Configuration. Go to Run > Edit Configurations, add a new JUnit configuration, and specify the main class. Execute the tests and view the results in the Run window.
Eclipse
For Eclipse, start by installing the Cucumber plugin. Go to Help > Eclipse Marketplace, search for “Cucumber Eclipse Plugin,” and install it. Restart Eclipse to complete the installation. Similar to IntelliJ, add Cucumber dependencies to your Maven pom.xml
:
<dependency>
<groupId>io.cucumber</groupId>
<artifactId>cucumber-java</artifactId>
<version>6.9.1</version>
</dependency>
Create a new JUnit test configuration by right-clicking on your project, selecting Run As > JUnit Test. This will execute your Cucumber tests, and results will be displayed in the JUnit view.
Visual Studio Code
Visual Studio Code requires the installation of the Cucumber (Gherkin) Full Support extension. Open the Extensions view by clicking the Extensions icon in the Activity Bar, search for “Cucumber (Gherkin) Full Support,” and install it. Add Cucumber dependencies to your project by editing the package.json
file:
"dependencies": {
"cucumber": "^6.9.1",
...
},
To run Cucumber tests, you can use the integrated terminal or a dedicated task runner extension. Open a terminal, navigate to your project directory, and run:
npx cucumber-js
Test results will be displayed directly in the terminal, showing detailed feedback on each executed scenario.
Understanding the Gherkin Language 🌟

The Gherkin language is an essential component used in Behavior-Driven Development (BDD) to write test cases in Cucumber. It allows for the creation of human-readable descriptions of software behaviors without requiring detailed knowledge of the underlying code. The syntax of Gherkin is designed to be simple and intuitive, ensuring that all team members, including non-technical stakeholders, can understand and contribute to the development process.
At the core of Gherkin are keywords that structure the test scenarios. These keywords include ‘Given’, ‘When’, ‘Then’, ‘And’, and ‘But’. Each serves a distinct purpose in defining the conditions and expected outcomes of a test scenario. Below is a summary of these keywords and their functions:
Keyword | Purpose |
---|---|
Given | Describes the initial context or preconditions. |
When | Specifies the event or action. |
Then | Defines the expected outcome or result. |
And | Connects multiple conditions or actions. |
But | Describes exceptions or contrasting conditions. |
Here are some examples of simple Gherkin statements:
Scenario: User login
Given the user is on the login page
When the user enters valid credentials
Then the user is redirected to the dashboard
Structuring Feature Files 📑
In the realm of Behavior-Driven Development (BDD), well-structured feature files are crucial for maintaining clear and effective test suites.
Each feature file should start with a Feature keyword, followed by a brief description that outlines the primary functionality being tested. This description should be concise yet descriptive enough to provide a clear understanding of the feature’s purpose. For instance:
Feature: User Login
This feature verifies the user login functionality of the application.
Following the feature description, the Background section can be used to define common steps that are prerequisite to all scenarios in the feature. This avoids redundancy and enhances readability. An example of a background step might be:
Background:
Given the user navigates to the login page
The core of the feature file consists of Scenario or Scenario Outline sections. Each scenario represents a specific case or behavior that needs testing. Scenarios should be written in a clear and straightforward manner using the Given-When-Then format. For example:
Scenario: Successful Login
Given the user is on the login page
When the user enters valid credentials
Then the user should be redirected to the dashboard
Using descriptive names for scenarios and steps is essential for clarity. Additionally, leveraging tags can significantly improve the manageability of your test suites. Tags allow you to categorize scenarios, making it easier to run specific groups of tests as needed. For instance:
@SmokeTest
Scenario: Successful Login
Given the user is on the login page
When the user enters valid credentials
Then the user should be redirected to the dashboard
Writing Scenarios and Steps with Examples 💻
Writing effective scenarios and steps in Cucumber requires a structured approach that ensures clarity and reusability. Scenarios in Cucumber are written in Gherkin syntax, which is designed to be human-readable while also being precise enough to be executed by machines. Let’s dive into the process with a practical example in Java.
Consider the following Gherkin scenario:
Feature: User Login
Scenario: Successful login with valid credentials
Given the user is on the login page
When the user enters valid username and password
And clicks the login button
Then the user should be redirected to the dashboard
To implement these steps in Java, we use step definitions. Here are the corresponding step definitions:
import io.cucumber.java.en.Given;
import io.cucumber.java.en.When;
import io.cucumber.java.en.Then;
public class LoginSteps {
@Given("the user is on the login page")
public void userIsOnLoginPage() {
// Code to navigate to login page
}
@When("the user enters valid username and password")
public void userEntersValidCredentials() {
// Code to enter username and password
}
@When("clicks the login button")
public void userClicksLoginButton() {
// Code to click login button
}
@Then("the user should be redirected to the dashboard")
public void userIsRedirectedToDashboard() {
// Code to verify redirection to dashboard
}
}
Key considerations when writing Cucumber scenarios and steps include:
- Reusability: Design steps that can be reused across different scenarios to avoid duplication.
- Data Handling: Use parameterized steps to handle dynamic data inputs. For example:
@When("the user enters {string} and {string}")
public void userEntersCredentials(String username, String password) {
// Code to enter username and password dynamically
}
- Integration: Integrate with other testing frameworks like JUnit or TestNG for robust testing capabilities.
- Edge Cases: Write scenarios that cover edge cases to ensure comprehensive test coverage.
Implementing Step Definitions
Step definitions form the backbone of Behavior-Driven Development (BDD) as they bridge the gap between the Gherkin syntax used in feature files and the underlying code that executes the test cases. In simpler terms, step definitions translate the natural language descriptions of test scenarios into executable code, ensuring that the BDD tests are both human-readable and machine-executable.
To implement step definitions, one needs to write corresponding code in a programming language that matches the phrases used in the Gherkin steps. Here, we will explore examples in three popular programming languages: Java, Python, and JavaScript.
Java Example
In Java, the Cucumber framework is commonly used for BDD. A step definition in Java might look like this:
import io.cucumber.java.en.Given;
import io.cucumber.java.en.When;
import io.cucumber.java.en.Then;
public class LoginSteps {
@Given("the user is on the login page")
public void userIsOnLoginPage() {
// Code to navigate to login page
}
@When("the user enters valid username and password")
public void userEntersValidCredentials() {
// Code to enter username and password
}
@When("clicks the login button")
public void userClicksLoginButton() {
// Code to click login button
}
@Then("the user should be redirected to the dashboard")
public void userIsRedirectedToDashboard() {
// Code to verify redirection to dashboard
}
}
Python Example
In Python, the Behave framework is often used. A step definition in Python might look like this:
from behave import given, when, then
@given('the user is on the login page')
def step_user_on_login_page(context):
# Code to navigate to the login page
@when('the user enters valid credentials')
def step_user_enters_valid_credentials(context):
# Code to enter username and password
@then('the user should be redirected to the dashboard')
def step_user_redirected_to_dashboard(context):
# Code to verify redirection to the dashboard
JavaScript Example
In JavaScript, the Cucumber.js framework is commonly utilized. A step definition in JavaScript might look like this:
const { Given, When, Then } = require('cucumber');
Given('the user is on the login page', function () {
// Code to navigate to the login page
});
When('the user enters valid credentials', function () {
// Code to enter username and password
});
Then('the user should be redirected to the dashboard', function () {
// Code to verify redirection to the dashboard
});
Common Pitfalls to Avoid

- Avoid coupling step definitions too tightly with the UI elements.
- Do not use overly generic step definitions, as they can become ambiguous.
- Ensure that step definitions are independent and do not rely on the execution order of other steps.
- Avoid hardcoding values; use parameterization for flexibility.
Running Tests from the Command Line
To run Cucumber tests from the command line, navigate to your project directory and execute one of the following commands:
mvn test
(for Maven projects)gradle test
(for Gradle projects)
This will initiate the test execution process, and results will be displayed in the terminal. Important steps or errors will be highlighted, helping you to identify any issues quickly. 🛠️
Integrating into CI/CD Pipelines
Integrating Cucumber tests into CI/CD pipelines ensures that tests are run automatically with each code commit. Popular CI/CD tools like Jenkins, Travis CI, and GitHub Actions support this integration. Here’s a sample Jenkins pipeline configuration for running Cucumber tests:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean install'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
}
post {
always {
junit '**/target/cucumber-reports/*.xml'
}
}
}
Generating and Interpreting Test Reports
Cucumber supports various formats for test reports, including HTML, JSON, and XML. Here is an example of generating an HTML report:
cucumber --plugin html:target/cucumber-reports/report.html
Open the generated report in a web browser to review the test results. The report will provide a detailed breakdown of which scenarios passed, failed, or were skipped, allowing you to pinpoint and address any issues swiftly.
Leveraging Data Tables and Examples 📝
Data tables in Cucumber are powerful tools that enhance the readability and maintainability of your test scenarios. These features allow you to handle multiple sets of data efficiently, streamlining your testing process.
It provide a simple way to pass a set of values to a step definition. They are especially useful when you need to test a scenario with various inputs and outputs. The syntax for data tables is straightforward: you define a table in the Gherkin scenario, and Cucumber translates it into a list of maps in your step definition.
Consider the following Gherkin scenario with a data table:
Scenario: User login with multiple credentials
Given the following users exist:
| username | password |
| user1 | pass1 |
| user2 | pass2 |
When the user logs in with username "user1" and password "pass1"
Then the login should be successful
In this scenario, the data table provides multiple sets of credentials. The step definition can then iterate over these credentials, verifying each login attempt.
Examples in Cucumber allow you to define multiple sets of data for a scenario outline. This feature is beneficial when you want to run the same scenario with different combinations of inputs and expected outputs. The syntax for examples is as follows:
Scenario Outline: User login
Given the user has navigated to the login page
When the user logs in with username "<username>" and password "<password>"
Then the login should be "<status>"
Examples:
| username | password | status |
| user1 | pass1 | successful |
| user2 | pass2 | failed |
In this example, the scenario outline runs twice: once with the username “user1” and password “pass1” expecting a successful login, and once with “user2” and “pass2” expecting a failed login. This approach makes your test scenarios more concise and easier to maintain.
To summarize the differences between data tables and examples, consider the following table:
Feature | Use Case | Syntax |
---|---|---|
Data Tables | When you need to pass multiple sets of data to a single step | | column1 | column2 | |
Examples | When you want to run a scenario multiple times with different data sets | Examples: | column1 | column2 | |
Harnessing the Power of Hooks and Tagged Hooks
Hooks in Cucumber are an essential feature that enables you to run specific blocks of code at various points during the execution of your test scenarios. These hooks allow for a higher degree of control and customization, making your test suite more robust and efficient. There are four main types of hooks: Before, After, BeforeStep, and AfterStep.
The Before hook runs before each scenario. Here’s a basic example:
@Before
public void setUp() {
// Code to set up test data
}
Similarly, the After hook executes after each scenario, which is ideal for cleanup actions:
@After
public void tearDown() {
// Code to clean up after tests
}
For more granular control, BeforeStep and AfterStep hooks can be utilized. These run before and after each step within a scenario:
@BeforeStep
public void beforeStep() {
// Code to run before each step
}
@AfterStep
public void afterStep() {
// Code to run after each step
}
Tagged hooks offer even more flexibility by allowing you to execute specific blocks of code based on tags assigned to your scenarios. For instance, you can use tagged hooks to run setup or teardown actions only for scenarios marked with a particular tag:
@Before("@Database")
public void setUpDatabase() {
// Code to set up database for scenarios tagged with @Database
}
@After("@Database")
public void tearDownDatabase() {
// Code to tear down database after scenarios tagged with @Database
}
Hooks are incredibly useful. For example, you can use a Before hook to pre-load test data into a database before each scenario begins. An After hook can then clean up the database to ensure that subsequent tests run in a clean environment.
Creating Custom Formatters and Reports 📊
Custom formatters in Cucumber allow you to define how the test results should be presented. Standard formatters like JSON, HTML, and JUnit are useful, but there are scenarios where a custom formatter is more beneficial. For instance, if your team needs to integrate test results with a bespoke dashboard or specific analytics tools, a custom formatter can bridge the gap effectively.

Creating a custom formatter involves implementing the Formatter
interface provided by Cucumber. Here is a step-by-step method:
1. Create a Class: Start by creating a new class that implements the Formatter
interface.
2. Implement Methods: Override the necessary methods such as startReport
, endReport
, and writeTestResult
.
3. Register Formatter: Register your custom formatter in the cucumber.yml
configuration file or via command line options.
4. Generate Report: Run your Cucumber tests, and your custom formatter will generate the report as specified.
Here’s a simplified example:
public class CustomFormatter implements Formatter {
public void startReport() {
// Initialization code
}
public void endReport() {
// Finalization code
}
public void writeTestResult(Scenario scenario) {
// Custom logic to format the test result
}
}
Once you’ve implemented your custom formatter, you can generate tailored reports that provide better insights into your test results. Options like integrating with CI/CD pipelines or exporting to custom dashboards become feasible, offering a comprehensive view of the test outcomes.
The use of custom reports can significantly improve the digestibility of test results. For instance, a custom HTML report with interactive charts can make it easier for stakeholders to understand the test coverage and pinpoint issues quickly.
FAQs
How does BDD differ from TDD (Test-Driven Development)?
While both BDD and TDD focus on writing tests before code, BDD emphasizes communication and collaboration by using human-readable language to describe the behavior of the system. TDD, on the other hand, is more technical and focuses on writing tests in the form of code.
What is Cucumber in the context of BDD?
Cucumber is a tool that supports BDD by allowing teams to write test cases in plain language, known as Gherkin. These test cases are then automated, bridging the gap between technical and non-technical team members.
What is Gherkin language?
Gherkin is a domain-specific language used in Cucumber to write test cases. It uses simple, structured syntax with keywords like Given, When, Then, And, and But to describe the behavior of the system in plain English.
Are there any advanced features in Cucumber?
Yes, Cucumber offers advanced features such as data tables for handling complex input, hooks for setup and teardown operations, tagged hooks for conditional execution, and custom formatters for generating reports.
Can Cucumber be integrated with Continuous Integration (CI) pipelines?
Yes, Cucumber can be integrated with CI pipelines. By incorporating Cucumber tests into your CI/CD process, you can automate the execution of your test cases and ensure continuous validation of your software.