REST API testing involves evaluating and validating the performance, reliability, and security of RESTful APIs. REST stands for Representational State Transfer, a design principle that uses HTTP requests to access and utilize data. Testing REST APIs ensures they function correctly and handle different types of requests and inputs effectively.
On This Page
Table of Contents
Importance of REST API Testing
REST APIs are crucial for connecting various software systems and enabling seamless communication between them. Testing these APIs is essential because:
- Ensures reliability: Verifies that APIs perform as expected under different conditions.
- Enhances security: Identifies potential vulnerabilities to protect sensitive data.
- Improves performance: Confirms that APIs handle high loads efficiently.
Types of API Testing
There are several types of API testing, each focusing on different aspects of the API:
Type | Description |
---|---|
Functional Testing | Checks if the API functions meet the required specifications. |
Load Testing | Assesses how the API performs under heavy traffic. |
Security Testing | Identifies vulnerabilities and ensures data protection. |
Integration Testing | Ensures the API integrates well with other systems. |
Benefits of Automated API Testing
Automating API testing offers numerous benefits:
- Efficiency: Automated tests run faster and more frequently than manual tests.
- Consistency: Ensures tests are performed the same way every time.
- Scalability: Easily handle large numbers of test cases.
For example, using tools like Postman or JMeter, we can create, run, and schedule tests to automatically verify API functionality, saving time and reducing human error.
Overview of REST Assured
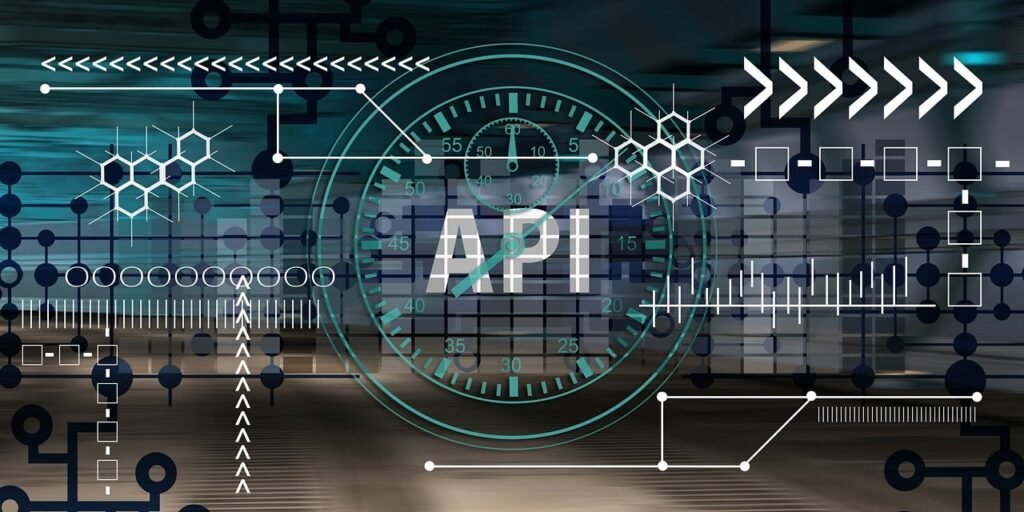
REST Assured is a powerful tool for testing RESTful web services. It is a Java domain-specific language (DSL) that simplifies the process of writing tests for REST APIs. By providing a simple and intuitive interface, REST Assured allows testers and developers to create robust test scripts with minimal effort.
Key Features and Advantages
REST Assured offers a variety of features that make it an excellent choice for API testing:
- Simple Syntax: The syntax is straightforward, making it easy to write and understand test cases.
- Integration with Testing Frameworks: It seamlessly integrates with popular testing frameworks like JUnit and TestNG.
- Supports JSON and XML: REST Assured can handle both JSON and XML formats, making it versatile for different API responses.
- Authentication Handling: It supports various authentication mechanisms, including OAuth, Basic, and Digest authentication.
- Assertions: REST Assured provides robust assertion capabilities to verify response data.
Installation and Setup
Getting started with REST Assured is straightforward. Follow these steps to set up REST Assured in your project:
- Add the REST Assured dependency to your
pom.xml
file if you are using Maven:
<dependency>
<groupId>io.rest-assured</groupId>
<artifactId>rest-assured</artifactId>
<version>4.3.3</version>
</dependency>
- Import REST Assured in your test class:
import static io.restassured.RestAssured.*;
Let’s look at a simple example of how to use REST Assured to test a REST API:
import static io.restassured.RestAssured.*;
import static org.hamcrest.Matchers.*;
public class ApiTest {
@Test
public void testGetUser() {
given()
.when()
.get("https://jsonplaceholder.typicode.com/users/1")
.then()
.statusCode(200)
.body("name", equalTo("Chai Time Tek"));
}
}
In this example, we are sending a GET request to retrieve user information and verifying that the status code is 200 and the user’s name is “Chai Time Tek”.
Adding Rest Assured to Project
Rest Assured is a popular Java library for testing RESTful web services. Follow the steps below to add it to your project:
- Create a new Maven .
- Add the following dependency to your
pom.xml
(for Maven) :
Maven:
<dependency>
<groupId>io.rest-assured</groupId>
<artifactId>rest-assured</artifactId>
<version>4.3.3</version>
</dependency>
Basic Configuration
Once Rest Assured is added to your project, you need to configure it. Here is a basic example of how to set up Rest Assured in your test class:
import io.restassured.RestAssured;
import org.junit.BeforeClass;
public class ApiTest {
@BeforeClass
public static void setup() {
RestAssured.baseURI = "https://api.example.com";
}
}
This configuration sets the base URI for all your API tests to https://api.example.com
. You can now write tests to verify different endpoints.
Understanding RESTful Endpoints
RESTful endpoints are fundamental components in the landscape of modern web services, especially when it comes to API testing. REST, an acronym for Representational State Transfer, is an architectural style that utilizes standard HTTP methods to facilitate communication between a client and a server.
These methods include GET, POST, PUT, DELETE, and PATCH, which correspond to the CRUD (Create, Read, Update, Delete) operations.
At its core, a RESTful endpoint is an URL that identifies resources on a server. For instance, a GET request to the endpoint /users
would retrieve a list of users, while a POST request to the same endpoint would create a new user. By using HTTP methods in combination with these endpoints, RESTful APIs offer a simple, consistent, and scalable approach to web services.
Consider the following examples of RESTful endpoints:
- GET /users: Retrieves a list of users.
- POST /users: Creates a new user.
- PUT /users/{id}: Updates the information of a specific user identified by their ID.
- DELETE /users/{id}: Deletes a specific user identified by their ID.
These endpoints make interaction with web services straightforward, as each URL represents a specific resource or action. When a request is made to a RESTful endpoint, the server processes it and returns the appropriate response, often in JSON or XML format.
Comparing REST with Other API Architectures
To better understand REST, Lets compare it with another popular API architecture: SOAP (Simple Object Access Protocol). Below is a table highlighting some key differences:
Aspect | REST | SOAP |
---|---|---|
Protocol | HTTP | HTTP, SMTP, TCP |
Message Format | JSON, XML | XML |
State | Stateless | Can be Stateless or Stateful |
Ease of Use | Simple, more flexible | More complex, rigid structure |
REST’s simplicity and flexibility make it a preferred choice for many developers, as it allows for more efficient and intuitive API testing.
Creating a Simple GET Request
To create our first GET request using Rest Assured, we’ll first need to set up the Rest Assured library in our preferred Integrated Development Environment (IDE).
In Maven. we need to add the following dependency to our `pom.xml` file:
<dependency>
<groupId>io.rest-assured</groupId>
<artifactId>rest-assured</artifactId>
<version>4.4.0</version>
</dependency>
Once the dependencies are set up, we can start writing our first GET request. Rest Assured makes it simple to interact with APIs and validate responses. Below is a basic example of how to send a GET request to a public API endpoint and handle the response:
import io.restassured.RestAssured;
import io.restassured.response.Response;
public class GetRequestExample {
public static void main(String[] args) {
Response response = RestAssured.get("https://jsonplaceholder.typicode.com/posts/1");
System.out.println("Response Code: " + response.getStatusCode());
System.out.println("Response Body: " + response.getBody().asString());
}
}
In this example, we are sending a GET request to the mock API endpoint `https://jsonplaceholder.typicode.com/posts/1`. The `get` method of Rest Assured sends the request, and the response is stored in the `response` object. We then print the HTTP status code and the response body to the console.
Understanding common HTTP status codes is crucial when working with APIs. A `200 OK` status code indicates that the request was successful and the server returned the requested data. Conversely, a `404 Not Found` status code means that the requested resource could not be found on the server. These status codes help in debugging and handling different scenarios in your test cases.
Validating Responses
When working with APIs, validating responses is a fundamental aspect of ensuring the reliability and accuracy of our applications. With Rest Assured, (a popular Java library used for testing RESTful web services), we can easily validate various aspects of an API response, including status codes, headers, and body content.
One of the first steps in response validation is to check the status code. This indicates whether the request was successful or if it encountered any errors. In Rest Assured, you can verify the status code using the statusCode()
method. For example:
given().when().get("/api/endpoint").then().statusCode(200);
Beyond status codes, headers also play a significant role in response validation. Headers contain metadata about the response, such as content type and encoding. We can validate headers using the header()
method. Here’s an example:
given().when().get("/api/endpoint").then().header("Content-Type", "application/json");
Validating the body content of a response is often more complex but equally important. Rest Assured provides powerful ways to assert that the response body contains specific data or meets certain criteria. For instance, to verify that the response body contains a particular field with a specific value, we can use the body()
method combined with JSONPath syntax:
given().when().get("/api/endpoint").then().body("data.id", equalTo(123));
For more advanced data extraction and validation, JSONPath and XPath are invaluable tools. JSONPath allows you to navigate and extract elements from a JSON document. Similarly, XPath is used for XML documents. Rest Assured integrates seamlessly with these tools, enabling precise and powerful validations:
given().when().get("/api/endpoint").then().body("data.items[0].name", equalTo("ItemName"));
given().when().get("/api/xml-endpoint").then().body(hasXPath("/response/data/item[name='ItemName']"));
Best Practices and Tips for Writing Effective Tests
Writing effective and maintainable API tests with Rest Assured requires a methodical approach. Clear and descriptive naming conventions for test methods make it easier to understand the purpose of each test at a glance. For instance, using names like testUserAuthentication or validateProductDetails significantly enhances readability.
Here are some key takeaways and tips for quick reference:
- Structure test cases for readability with clear naming conventions.
- Adopt a data-driven testing approach for efficiency.
- Handle authentication and authorization appropriately.
- Maintain clean code practices to enhance maintainability.
- Manage dependencies and configurations using build tools and configuration files.
- Implement retry mechanisms and use mocks to handle real-world challenges.
Advanced Techniques: POST, PUT, and DELETE Requests
When working with RESTful APIs, mastering advanced techniques for making POST, PUT, and DELETE requests using REST Assured is essential. These requests are fundamental for creating, updating, and deleting resources, respectively.
POST Requests
POST requests are used to create new resources. In REST Assured, you can set up a POST request by specifying the endpoint, headers, and body. Here’s an example of creating a user profile:
given()
.header("Content-Type", "application/json")
.body("{ \"name\": \"Chai Time Tek\", \"email\": \"sample@cloudcusp.com\" }").when()
.post("/users").then()
.statusCode(201)
.body("id", notNullValue());
PUT Requests
PUT requests are used to update existing resources. The process is similar to POST but usually requires specifying the resource identifier. Here’s an example of updating a user profile:
given()
.header("Content-Type", "application/json")
.body("{ \"name\": \"Chai Time Tek\", \"email\": \"sample@cloudcusp.com\" }").when()
.put("/users/1").then()
.statusCode(200)
.body("name", equalTo("Chai Time Tek"));
DELETE Requests
DELETE requests remove a resource. This request typically needs the resource identifier. Here’s an example of deleting a user profile:
given().when()
.delete("/users/1").then()
.statusCode(204);
Comparison of POST, PUT, and DELETE Requests
The following table summarizes the differences between POST, PUT, and DELETE requests:
Request Type | Operation | Typical Status Codes | Usage |
---|---|---|---|
POST | Create | 201 (Created), 400 (Bad Request) | Used to create a new resource. |
PUT | Update | 200 (OK), 404 (Not Found) | Used to update an existing resource. |
DELETE | Delete | 204 (No Content), 404 (Not Found) | Used to delete a resource. |
These methods are crucial for comprehensive API testing, ensuring the proper creation, updating, and deletion of resources.
FAQs
What are the benefits of using Rest Assured for API testing?
Rest Assured offers a user-friendly syntax for creating and validating API requests. It supports various HTTP methods, response validation, authentication, and integration with test frameworks like JUnit. These features make it a powerful tool for ensuring API reliability.
Can Rest Assured handle different types of HTTP requests?
Yes, Rest Assured can handle various types of HTTP requests, including GET, POST, PUT, and DELETE. You can use it to test and validate different API operations.
How can I test secure APIs with Rest Assured?
Rest Assured supports testing secure APIs by allowing you to configure authentication methods like Basic Authentication, OAuth2, and token-based authentication. This ensures that your tests can handle secure endpoints effectively.
What is data-driven testing in Rest Assured?
Data-driven testing involves using external data sources to run the same test with multiple sets of data. Rest Assured supports data-driven testing by allowing you to parameterize test cases and execute them with different inputs.
What are some best practices for writing tests with Rest Assured?
Best practices include organizing test cases logically, using clear assertions, keeping tests independent, and managing test data effectively. Writing maintainable and reliable tests helps ensure your API remains robust over time.