Postman is a powerful collaboration platform for API development. It has become an indispensable tool for developers and QA engineers due to its user-friendly interface and robust features. Primarily, Postman is used for API testing, which involves sending various types of HTTP requests to a web server and analyzing the responses. This process allows developers to ensure that their APIs are functioning as expected.
On This Page
Table of Contents
Key Features of Postman
One of the key features of Postman is the ability to create and manage API requests. These requests can be saved in collections, which are essentially organized groups of requests. This makes it easier for developers to keep their work structured and share it with team members. Additionally, Postman supports environments, enabling users to define different sets of variables (like API keys and URLs) for various stages of development, such as local development, staging, and production.
To further illustrate Postman’s advantages, here’s a comparison table with other popular API tools:
Feature | Postman | Insomnia | Swagger |
---|---|---|---|
API Request Management | โ | โ | โ |
Collections | โ | โ | โ |
Environments | โ | โ | โ |
Collaboration Tools | โ | โ | โ |
Installing Postman
To begin using Postman, the first step is to install the application on your computer. Postman is compatible with various platforms, including Windows, macOS, and Linux. Below, we provide detailed, step-by-step instructions for each platform to ensure a smooth installation process.
Windows | macOS | Linux |
---|---|---|
1. Visit the Postman Downloads page. | 1. Navigate to the Postman Downloads page. | 1. Go to the Postman Downloads page. |
2. Click on the Windows download button. | 2. Click on the macOS download button. | 2. Download the appropriate Linux version (Snap or tar.gz). |
3. Once the download is complete, open the installer and follow the on-screen instructions. | 3. Open the downloaded file. | 3. For Snap, open the terminal and run: sudo snap install postman |
4. After installation, Postman will automatically launch. | 4. Drag the Postman app to your Applications folder. | 4. For tar.gz, extract the file and move it to the desired location. |
5. Launch Postman from your Applications folder. | 5. Launch Postman by running the executable. |
Navigating the Postman Interface
Getting familier with the Postman interface is crucial for optimizing your workflow. The Postman interface is designed to be intuitive and user-friendly, making it accessible for beginners and efficient for experienced users. Here, we will navigate through the primary components of the Postman interface: the workspace, collections, request builder, and response viewer.
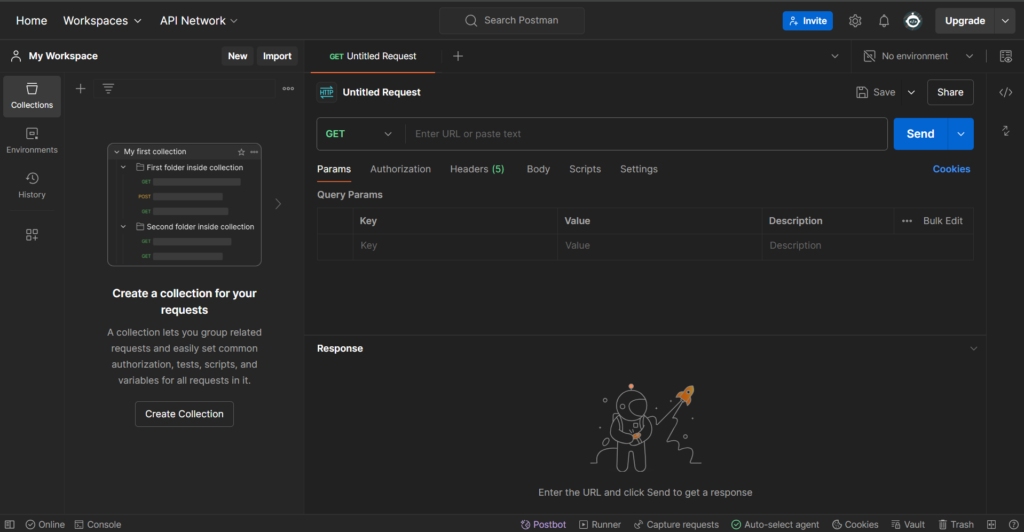
Workspace ๐ฅ๏ธ
The workspace is the central hub of Postman, where all your API testing activities happen. It allows you to manage your API requests, collections, environments, and more, all in one place. You can create multiple workspaces for different projects, ensuring your work remains organized and manageable.
Collections ๐
Collections are a powerful feature in Postman that allow you to group related API requests. This is especially useful for organizing tests and scripts for different parts of an API. For example, a QA engineer might create a collection for user authentication tests and another for product management tests. Collections can be further subdivided into folders for even more granularity.
Request Builder ๐ ๏ธ
The request builder is where you construct your API requests. It comprises several key areas:
- HTTP Method: Choose the type of request (GET, POST, PUT, DELETE, etc.).
- URL: Enter the endpoint you want to test.
- Headers: Add any necessary headers required for your request.
- Body: Include data payloads for POST or PUT requests.
Tips for efficient use:
- Utilize environment variables to manage different settings for various environments (e.g., development, staging, production).
- Save frequently used requests to collections for quick access.
Response Viewer ๐
Once a request is sent, the response viewer displays the serverโs response. It provides valuable information, including status codes, response time, and the actual response body. This tool is essential for debugging and validating API behavior. You can also view response headers and cookies.
Creating Your First API Request
To get started with Postman, letโs create a simple GET request to a public API. For this example, we’ll use a weather service API to retrieve current weather data. Follow these steps to create your first API request:
Step-by-Step Instructions
1. Open Postman: Launch the Postman application on your computer.
2. Create a new request: Click on the โNewโ button in the top-left corner, then select โRequestโ from the dropdown menu.
3. Name your request: Enter a name for your request, such as โWeather Data Request,โ and click โSave to Collection.โ
4. Set the HTTP method: In the request builder, select โGETโ from the dropdown menu next to the URL field.
5. Enter the URL: Type in the URL of the weather API. For example, you can use: https://api.openweathermap.org/data/2.5/weather?q=London&appid=your_api_key
. This URL includes the endpoint, query parameters (city name and API key), and necessary credentials.
6. Configure the parameters: In the โParamsโ tab, you can see the parameters parsed from the URL. Make sure the values are correct.
7. Set headers (if needed): For many public APIs, headers might not be necessary. But if required, click the โHeadersโ tab and add key-value pairs for any needed headers.
8. Send the request: Click the โSendโ button to dispatch the GET request to the API endpoint.
9. Interpret the response: The response from the API will appear in the lower part of the Postman interface. You can view it in different formats like JSON, HTML, or raw text. The status code (e.g., 200 OK) indicates the result of your request.
Example 2: Fetching User Data from a Social Media API
Imagine you want to fetch user data from a social media API. The process is similar:
1. Set the URL: https://api.socialmedia.com/users?username=johndoe&apikey=your_api_key
2. Check the parameters: Ensure โusernameโ and โapikeyโ are correctly set.
3. Send the request: Click โSendโ and review the response to check the userโs data.
Example 3: Inserting New User in a mock API
let’s go through the process of sending a POST request to create a new user in a mock API. Begin by opening Postman and setting the request type to POST. In the URL field, enter the endpoint URL for the mock API, for example, https://mockapi.io/users
.
Next, you need to configure the request headers. Headers provide additional context about the request. For a POST request that includes JSON data, you’ll typically need to set the Content-Type
header to application/json
.
Once the headers are set, move on to the request body. In Postman, select the “Body” tab and choose the “raw” option. Ensure the format is set to JSON and then input the data you wish to send. For example, to create a new user, your JSON might look like this:
{
"name": "John Doe",
"email": "john.doe@example.com",
"password": "securepassword"
}
With the URL, headers, and body configured, you are ready to execute the request. Click the “Send” button. Postman will process the request and display the response from the server in the lower section of the interface.
The response will typically include a status code, such as 201 for a successful creation, and a body containing the details of the newly created user or an error message if something went wrong. By carefully examining the response, you can verify whether the request was successful and troubleshoot any issues that arise.
If you encounter issues, consider these tips:
- Verify the URL and parameters are correct.
- Check your API key and other credentials.
- Review the API documentation for required headers or additional parameters.
- Use the Postman console for more detailed error messages.
Below is a summary of the most common HTTP methods and their typical applications:
HTTP Method | Use Case |
---|---|
GET | Retrieve data from a server |
POST | Create new resources |
PUT | Update existing resources |
DELETE | Remove resources |
Writing Tests in Postman
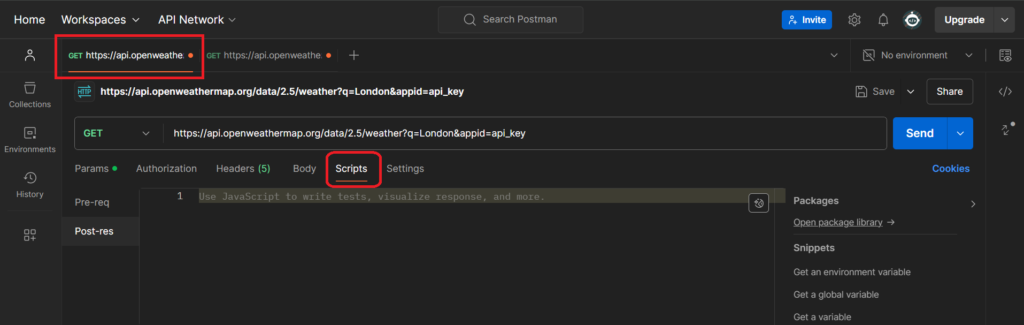
To get started with writing tests in Postman, navigate to the “Scripts” tab of your request. Here, you can write JavaScript code that will run after the request is executed. This feature allows you to create various assertions to check different aspects of the API response.
Here are some common assertions you might use:
1. Checking Status Codes
Ensuring that the response status code is as expected is a fundamental test. For example, if you expect a successful response, you can check for a status code of 200:
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
2. Response Times
Monitoring response times helps in identifying performance issues. You can set a threshold for acceptable response times:
pm.test("Response time is less than 500ms", function () {
pm.expect(pm.response.responseTime).to.be.below(500);
});
3. Validating Response Body
Often, you need to verify specific data within the response body. For instance, you can check if a particular field contains the expected value:
pm.test("Response body contains userId", function () {
var jsonData = pm.response.json();
pm.expect(jsonData.userId).to.eql(1);
});
Using Environment Variables and Collections
When conducting API testing with Postman, leveraging environment variables and collections can significantly optimize efficiency and organization. Environment variables in Postman are placeholders for information that can vary between environments, such as API keys, URLs, or tokens. By using environment variables, we can seamlessly switch settings between different environments like development, staging, and production without having to manually update each request.
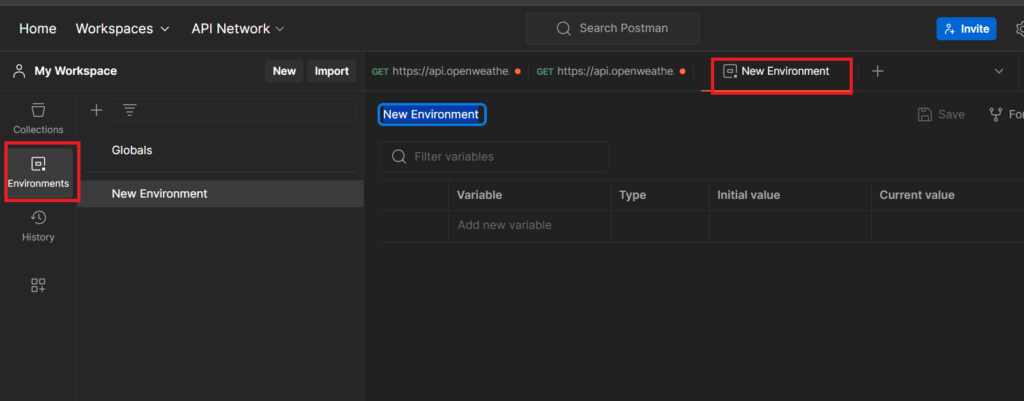
To create an environment variable, navigate to the ‘Environments‘ tab and click ‘Add‘. Define the variable name and its corresponding value. For instance, you might have a variable named baseURL
with the value https://api.dev.yourapp.com
for your development environment. This can later be updated to https://api.prod.yourapp.com
for your production environment.
Here is a step-by-step guide to illustrate the process:
- Create Environment Variables: Navigate to ‘Environments’ > ‘Add’, define variable names and values.
- Use Variables in Requests: Incorporate variables in your URLs, headers, or request bodies using double curly braces, e.g.,
{{baseURL}}/users
. - Create Collections: Go to ‘Collections’ > ‘New Collection’, name it and add related requests.
- Organize Requests: Group related requests under a collection and use folders for further categorization.
Consider a scenario where you are testing an API across multiple environments. By using collections and environment variables, you can streamline your workflow. For instance, you can create a collection named ‘User Management’ with requests for creating, updating, and deleting users. Use environment variables to manage different settings for development, staging, and production environments.
Advanced Testing in Postman
Imagine you’re working on an e-commerce platform where the accuracy of product information is critical. With data-driven testing, you can ensure your API returns the correct product details under various conditions by systematically feeding different data sets into your tests. Similarly, pre-request and test scripts allow you to set up and validate complex scenarios, such as user authentication flows or payment processing, with ease.
Furthermore, integrating Postman with CI/CD pipelines empowers teams to incorporate API testing into their development workflows seamlessly. This integration ensures that any changes to the codebase are automatically validated against predefined test cases, significantly reducing the risk of introducing bugs into production environments.
Data-Driven Testing ๐
Data-driven testing is an essential methodology in software quality assurance that involves running test cases with multiple sets of data to ensure comprehensive test coverage. This approach allows Testing team to validate the behavior of an application under various conditions, thereby identifying edge cases and potential defects that could be missed with static testing methods.
Here’s a step-by-step guide on how to set up data files in Postman, utilize variables, and iterate through data sets:
Setting Up Data Files in Postman
1. Prepare your data file: Create a JSON or CSV file containing the data sets you wish to test. For instance, if you’re testing multiple user login credentials, your CSV file might look like this:
username,password
user1,pass1
user2,pass2
user3,pass3
Using Variables
2. Import the data file: In Postman, open your collection and go to the “Runner” tab. Click “Select File” and upload your JSON or CSV file.
3. Set up variables: In your request, replace static values with variables that correspond to the column headers in your data file. For example, replace the username and password fields with {{username}}
and {{password}}
.
Iterating Through Data Sets
4. Configure the iteration: In the Runner, specify the number of iterations. Postman will automatically iterate through the rows of your data file, running the collection with each set of data.
5. Execute the tests: Click “Start Test” to run your collection with the data sets. Postman will iterate through each row of your file, substituting the variables and executing the test cases accordingly.
Key Steps Summary
Step | Description |
---|---|
1 | Prepare your data file (CSV/JSON) |
2 | Import the data file into Postman Runner |
3 | Set up variables in your request |
4 | Configure the iteration count |
5 | Execute the tests |
Benefits of Data-Driven Testing
โข Comprehensive test coverage
โข Efficient identification of edge cases
โข Reduced manual testing effort
โข Enhanced test reliability and accuracy
โข Facilitation of automated regression testing
Enhancing Tests with Pre-request and Test Scripts ๐ ๏ธ
Postman provides robust testing capabilities through the use of pre-request and test scripts. These scripts, written in JavaScript, empower users to automate and enhance their API testing workflows.

Pre-request Scripts: These scripts run before the actual API request is sent, making them ideal for tasks like setting up environment variables, fetching authentication tokens, or manipulating request parameters. For example, you can use a pre-request script to dynamically generate a timestamp to include in the request:
pm.environment.set("currentTimestamp", new Date().toISOString());
Another common use case is fetching an authentication token from an external service before making an API request:
pm.sendRequest({
url: 'https://auth-service.com/token',
method: 'POST',
header: {
'Content-Type': 'application/json'
},
body: {
mode: 'raw',
raw: JSON.stringify({ username: 'user', password: 'pass' })
}
}, function (err, res) {
pm.environment.set("authToken", res.json().token);
});
Test Scripts: These scripts execute after receiving a response from the API, allowing users to validate response data, check headers, and assert conditions. For instance, you can verify that the status code of a response is 200 OK:
pm.test("Status code is 200", function () {
pm.response.to.have.status(200);
});
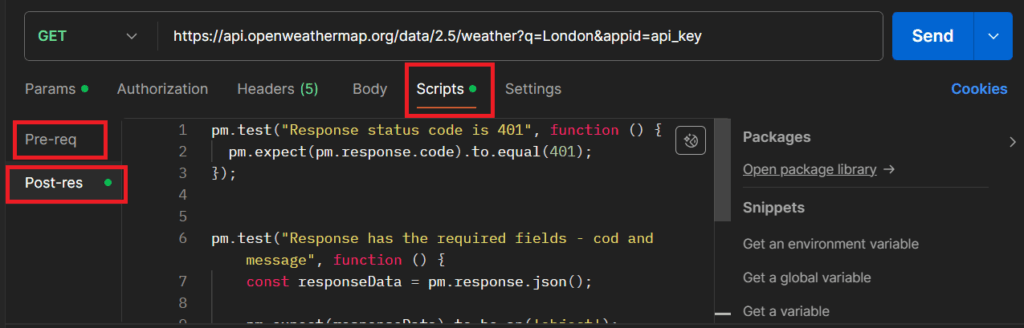
Additionally, you can check for specific headers or validate the structure and content of the response body. For example, verifying that the response contains a valid JSON object:
pm.test("Response is JSON", function () {
pm.response.to.be.json;
});
Consider a scenario where you are verifying API responses for a weather application. A pre-request script could be used to set the geographic coordinates dynamically, while a test script could assert that the temperature value in the response falls within an expected range:
// Pre-request script to set coordinates
pm.environment.set("latitude", "40.7128");
pm.environment.set("longitude", "-74.0060");
// Test script to validate temperature
pm.test("Temperature is within range", function () {
var temperature = pm.response.json().main.temp;
pm.expect(temperature).to.be.within(273.15, 313.15); // Kelvin
});
By leveraging the power of pre-request and post-res /test scripts, you can significantly enhance the efficiency and reliability of your Postman tests, ensuring robust and accurate API validation.
Integrating Postman with CI/CD Pipelines ๐
Integrating Postman with Continuous Integration/Continuous Deployment (CI/CD) pipelines provides numerous benefits, including automated testing and enhanced code quality. This integration allows developers to validate APIs continuously throughout the development lifecycle, ensuring that any issues are detected and addressed promptly.
To begin, Postman collections can be set up in a CI/CD pipeline using popular tools like Jenkins, Travis CI, or GitHub Actions. Here’s a step-by-step guide for integrating Postman with these tools:
Jenkins
- Install the Postman plugin.
- Create a new Jenkins job and configure it to run your Postman collection.
- Set up the build triggers to initiate the job automatically.
- Monitor the build results to ensure your API tests pass successfully.
Travis CI
- Add the Postman Newman dependency to your project.
- Configure the `.travis.yml` file to run the Postman collection.
- Push your changes to GitHub, and Travis CI will automatically trigger the build.
- Review the build logs to verify the test outcomes.
GitHub Actions
- Create a new GitHub Actions workflow file.
- Define the steps to install Newman and run your Postman collection.
- Commit the workflow file to your repository, and GitHub Actions will execute it on each push.
- Check the Actions tab for the test results.
Comparison of CI/CD Tools
Tool | Integration Ease | Build Trigger Support | Community Support |
---|---|---|---|
Jenkins | High | Comprehensive | Strong |
Travis CI | Moderate | Basic | Good |
GitHub Actions | High | Comprehensive | Growing |
For an example, consider an e-commerce platform that needs to ensure its API endpoints are functioning correctly. By integrating Postman collections into a CI/CD pipeline, the team can automate testing for various functionalities such as product listings, user authentication, and order processing. This setup promises that any changes to the codebase do not introduce new bugs or regressions.
Advantages of CI/CD Integration
- Automated and continuous testing
- Early detection of issues
- Improved code quality
- Reduced manual testing efforts
- Streamlined development workflows
FAQs
What is Postman?
Postman is a popular API development tool that allows developers to design, test, and document APIs. It provides a user-friendly interface for sending requests, writing tests, and organizing API collections.
Can I use variables in Postman tests?
Yes, Postman supports environment and global variables. You can use these variables to store values and reuse them across multiple requests and tests.
What are some best practices for automating API tests?
Some best practices for automating API tests include:
Organize your tests into collections and folders.
Use environment variables to manage different test environments.
Write clear and concise test scripts.
Implement data-driven testing for comprehensive coverage.
Regularly update and maintain your tests to reflect API changes.
How can I integrate Postman tests with my CI/CD pipeline?
To integrate Postman tests with your CI/CD pipeline, you can add Newman commands to your build scripts. Most CI/CD tools, such as Jenkins, Travis CI, and GitLab CI, support running Newman as part of the build process.