Docker is a platform designed to make it easier for developers to create, deploy, and run applications by using containers. Containers allow developers to package an application with all of its dependencies into a standardized unit for software development. Think of it as a lightweight, stand-alone, and executable package that includes everything needed to run a piece of software, including code, runtime, system tools, libraries, and settings.
On This Page
Table of Contents
What is Docker?🐳
Docker was introduced in 2013 by a company called dotCloud, which later changed its name to Docker, Inc. The platform quickly gained popularity because it addressed many problems developers faced, such as the ‘it works on my machine’ dilemma. By using containers, Docker ensures that the software will run the same, regardless of the environment.
Key Components of Docker
Docker consists of several key components that work together to create a seamless experience for developers:
- Docker Engine: This is the core of Docker, responsible for creating and managing containers.
- Docker Hub: A cloud-based repository where developers can store and share container images.
- Docker Compose: A tool for defining and running multi-container Docker applications.
Why Use Docker?
Using Docker offers several advantages:
- 🚀 Speed: Containers are lightweight and start quickly.
- 🔄 Consistency: Ensures the application runs the same in different environments.
- 📦 Portability: Containers can run on any platform that supports Docker.
- 🔒 Isolation: Each container runs in its own isolated environment.
Traditionally, setting up the development environment might involve installing various software and configuring them to work together. With Docker, the team can create a container that includes the web server, database, and any other required services. This container can then be shared among team members, ensuring that everyone is working in the same environment, thereby reducing inconsistencies and bugs.
🐳 Containers

Containers have revolutionized the way we develop and deploy applications. In simple terms, a container is a lightweight, standalone, and executable package that includes everything needed to run a piece of software, such as code, runtime, system tools, libraries, and settings. 🛠️
Difference Between Containers and Virtual Machines
Containers and virtual machines (VMs) are often compared, but they have distinct differences. Here’s a quick comparison:
Containers | Virtual Machines |
---|---|
Share the host OS kernel | Include a full OS |
Lightweight and fast | Heavy and slower |
Ideal for microservices | Suitable for running multiple OS |
How Containers Work
Containers leverage OS-level virtualization to run multiple isolated systems on a single host using the host’s OS kernel. This is achieved through:
- Namespaces: Isolate the container’s processes, networking, and file system.
- Control Groups (cGroups): Manage and limit the resource usage of containers.
By sharing the host OS kernel, containers can start up quickly and use fewer resources compared to VMs.
Benefits of Using Containers in Web Development
Containers offer numerous advantages for web development, including:
- Consistency: Ensure the application runs the same in development, testing, and production environments.
- Scalability: Easily scale applications up or down by adding or removing containers.
- Portability: Run your application on any system that supports containerization.
- Efficiency: Improve resource utilization and reduce overhead costs.
For example, developers can use Docker to containerize a web application, ensuring it runs consistently across different environments. Here’s a simple Dockerfile example:
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD ["node", "server.js"]
This Dockerfile sets up a Node.js application, demonstrating how easy it is to containerize and deploy web applications.
Containers are a powerful tool for modern web development, offering consistency, scalability, portability, and efficiency.
Installing Docker on Different Operating Systems 🐳
Depending on your operating system, the installation process for Docker varies. Here’s a simple guide:
Windows
1. Download Docker Desktop for Windows from the official Docker website.
2. Run the installer and follow the on-screen instructions.
3. After installation, launch Docker Desktop and let it initialize.
MacOS
1. Download Docker Desktop for Mac from the official Docker website.
2. Open the downloaded .dmg file and drag Docker to your Applications folder.
3. Launch Docker from Applications, and it will start initializing.
Linux
1. Open a terminal and update your package index:
sudo apt-get update
2. Install Docker:
sudo apt-get install docker-ce docker-ce-cli containerd.io
3. Start the Docker service:
sudo systemctl start docker
Basic Docker Commands
Once Docker is installed, you can start using it with some basic commands. Here are a few to get you started:
docker --version
: Check Docker’s version.docker pull <image_name>
: Pull an image from Docker Hub.docker run <image_name>
: Run a container from an image.docker ps
: List running containers.docker stop <container_id>
: Stop a running container.
Let’s set up a simple web server using Docker. We’ll use the official NGINX image from Docker Hub:
docker run -d -p 8080:80 nginx
This command will:
- Download the NGINX image if it’s not already present.
- Run a new container from the image in detached mode (
-d
). - Map port 8080 on your host to port 80 in the container (
-p 8080:80
).
Open your web browser and navigate to http://localhost:8080, and you should see the NGINX welcome page! 🌐 Happy Dockering! 🚀
Writing a Simple Dockerfile
The Dockerfile is the blueprint for building Docker images. Here’s a basic example to get you started:
# Start with a base image
FROM ubuntu:latest
# Install dependencies
RUN apt-get update && apt-get install -y python3 python3-pip
# Copy application files
COPY . /app
# Set the working directory
WORKDIR /app
# Run the application
CMD ["python3", "app.py"]
In this example, we:
- Start from the latest Ubuntu image
- Install Python and pip
- Copy application files to the container
- Set the working directory
- Run the application
Building and Running a Container
To build your Docker image, navigate to the directory containing your Dockerfile and run:
docker build -t my-first-container .
This command builds the image and tags it as my-first-container
. Once the build is complete, you can run the container with:
docker run -d -p 5000:5000 my-first-container
This command runs the container in detached mode and maps port 5000 of the container to port 5000 on your host machine.
Understanding the Container Lifecycle
Managing Docker containers involves understanding their lifecycle:
Stage | Description |
---|---|
Building | Creating a Docker image from a Dockerfile |
Running | Starting a container from an image |
Stopping | Halting a running container |
Removing | Deleting a container or image |
For example, to stop a running container, use:
docker stop <container_id>
And to remove a container:
docker rm <container_id>
Docker for Web Development
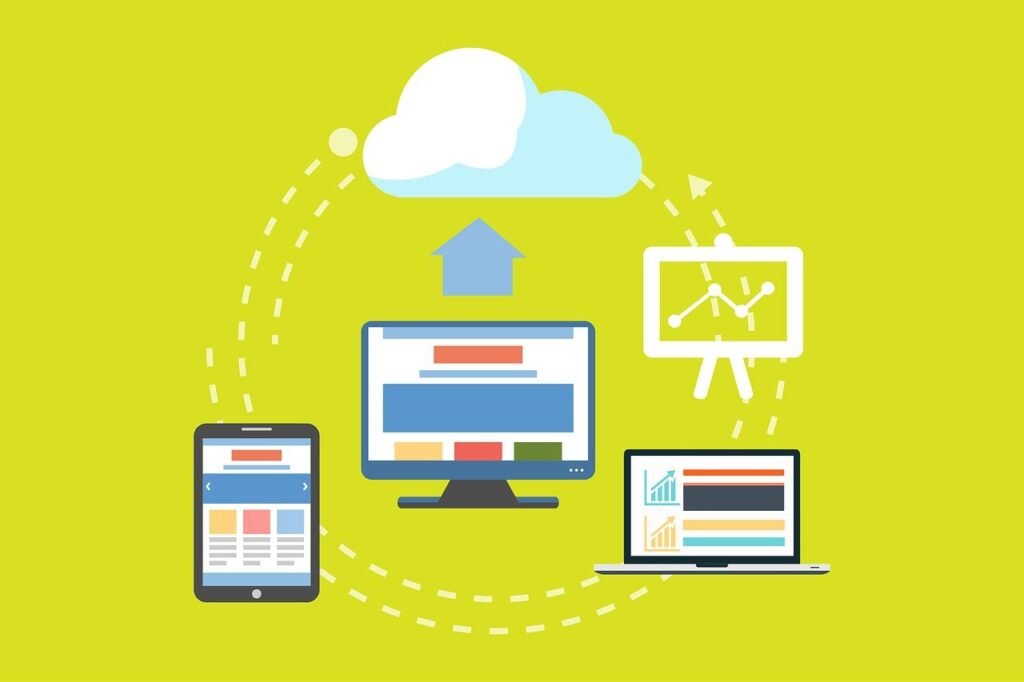
Docker has revolutionized the way we develop, deploy, and manage applications. 🐳 For web developers, Docker offers a streamlined and efficient approach to creating and maintaining development environments.
Advantages of Using Docker in Web Development
Docker provides several benefits that make it an excellent choice for web development:
- Consistency: Docker ensures that your application runs the same way across different environments, reducing the ‘it works on my machine’ problem.
- Isolation: Each Docker container operates in its own isolated environment, preventing dependencies and configurations from interfering with each other.
- Scalability: Docker makes it easy to scale applications up or down by adding or removing containers.
- Portability: Docker containers can run on any system that supports Docker, making it easy to move applications between development, testing, and production environments.
- Efficiency: Docker containers are lightweight and start quickly, allowing for faster development and deployment cycles.
Setting Up a Development Environment with Docker
Getting started with Docker is straightforward. Here’s a simple guide to setting up a Docker-based development environment:
- Install Docker: Download and install Docker from the official website.
- Create a Dockerfile: This file defines your application’s environment. For example:
FROM node:14
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
EXPOSE 3000
CMD ["npm", "start"]
- Build the Docker Image: Run
docker build -t my-web-app .
in your terminal. - Run the Container: Start your container with
docker run -p 3000:3000 my-web-app
.
Containerizing a Simple Web Application
Let’s take a look at a practical example of containerizing a simple web application:
// app.js
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => res.send('Hello, Docker!'));
app.listen(port, () => console.log(`App running on port ${port}`));
With the above code and a corresponding Dockerfile, you can easily create a Docker container that runs your web application.
Docker is a powerful tool for web development, offering consistency, isolation, scalability, portability, and efficiency.
Introduction to Docker Compose
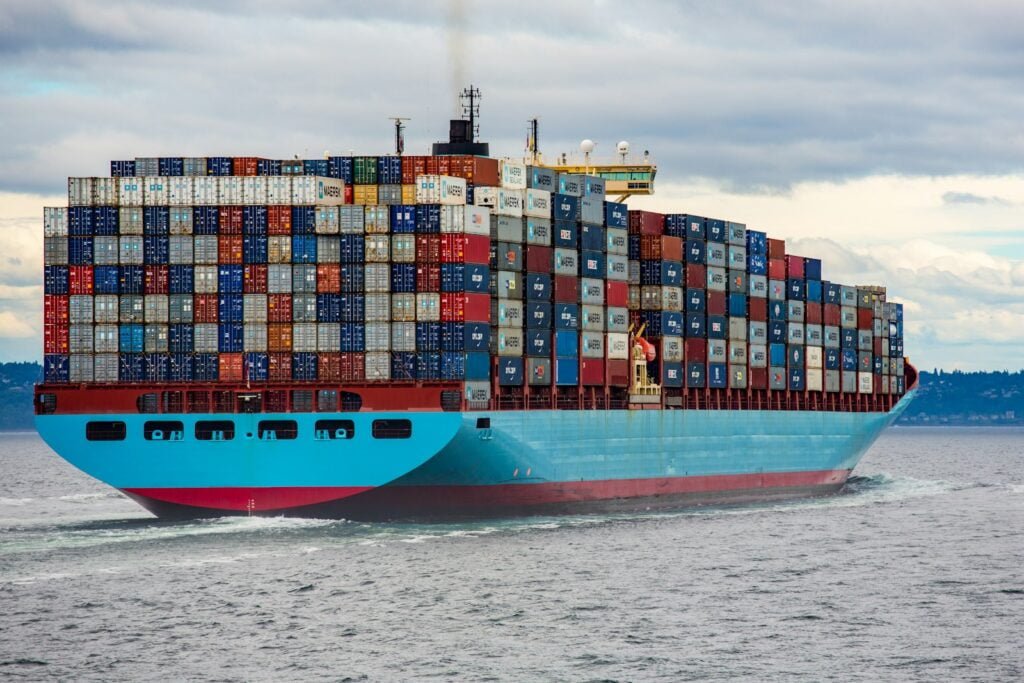
Docker Compose is a powerful tool that simplifies the management of multi-container applications. Whether you’re running a microservices architecture or a complex stack, Compose allows you to define and run multi-container Docker applications with ease. Think of it as an orchestra conductor for your containers 🎶.
Defining Services in a Docker-Compose.yml File
At the heart of Docker Compose is the docker-compose.yml
file. This file is where you define the services that make up your application. Each service corresponds to a Docker container. Here’s a simple example:
version: '3'
services:
web:
image: nginx
ports:
- '80:80'
db:
image: postgres
environment:
POSTGRES_PASSWORD: example
In this example, there are two services: web (using the Nginx image) and db (using the PostgreSQL image). The web service maps port 80 on the host to port 80 in the container, while the db service sets an environment variable for the PostgreSQL password.
Running and Managing Multi-Container Applications
Once your docker-compose.yml
file is ready, you can start your application with a single command:
docker-compose up
This command brings up all the defined services. You can also use -d
to run them in detached mode:
docker-compose up -d
To stop the services, simply run:
docker-compose down
Managing multi-container applications has never been easier. With Docker Compose, you can:
- Scale services up or down
- View logs with
docker-compose logs
- Execute commands inside running services
Example: A Web Application
Imagine you’re developing a web application that consists of a front-end, a back-end, and a database. With Docker Compose, you can define all these services in a docker-compose.yml
file:
version: '3'
services:
frontend:
image: react-app
ports:
- '3000:3000'
backend:
image: express-api
ports:
- '5000:5000'
db:
image: mongo
ports:
- '27017:27017'
Running docker-compose up
will start all three services, enabling seamless interaction between the front-end, back-end, and database. This setup is ideal for development and testing environments, ensuring consistency and reproducibility across different stages of your project 🚀.
Docker Volumes 📦
Docker volumes are an essential tool for managing data in containerized applications. They allow you to persist data generated by the container, ensuring that it isn’t lost when the container stops or is removed. 📦
Using Volumes to Persist Data
Persisting data is crucial for applications that require data to be available even after a container is terminated. Docker volumes provide a way to store this data outside the container’s filesystem. Let’s look at a simple example:
docker run -d -v my_volume:/app/data my_image
In this command, -v my_volume:/app/data
creates a volume named my_volume
and mounts it to the /app/data
directory inside the container.
Sharing Data Between Containers
One of the powerful features of Docker volumes is the ability to share data between multiple containers. This can be particularly useful for microservices architectures where different services need access to the same data.
Consider the following example where two containers share the same volume:
docker run -d -v shared_volume:/shared/data container1
docker run -d -v shared_volume:/shared/data container2
Both container1
and container2
can read and write to the shared_volume
. This setup ensures data consistency and simplifies data management across services. 🔄
Imagine a web application with a backend API and a frontend service. Both services need access to a common configuration file:
docker volume create config_volume
docker run -d -v config_volume:/config backend_service
docker run -d -v config_volume:/config frontend_service
By using a shared volume, both the backend and frontend services can access and update the configuration file, ensuring they are always in sync. 📝
Benefits of Using Docker Volumes
- Data persistence across container restarts and removals
- Easy data sharing between containers
- Improved data management and consistency
- Isolation of data from the container filesystem
Docker volumes are a powerful feature that offers numerous benefits for managing data in containerized applications. By understanding how to create and use volumes, you can ensure data persistence, facilitate data sharing, and streamline your data management processes. 🚀
Docker Networking 🖧
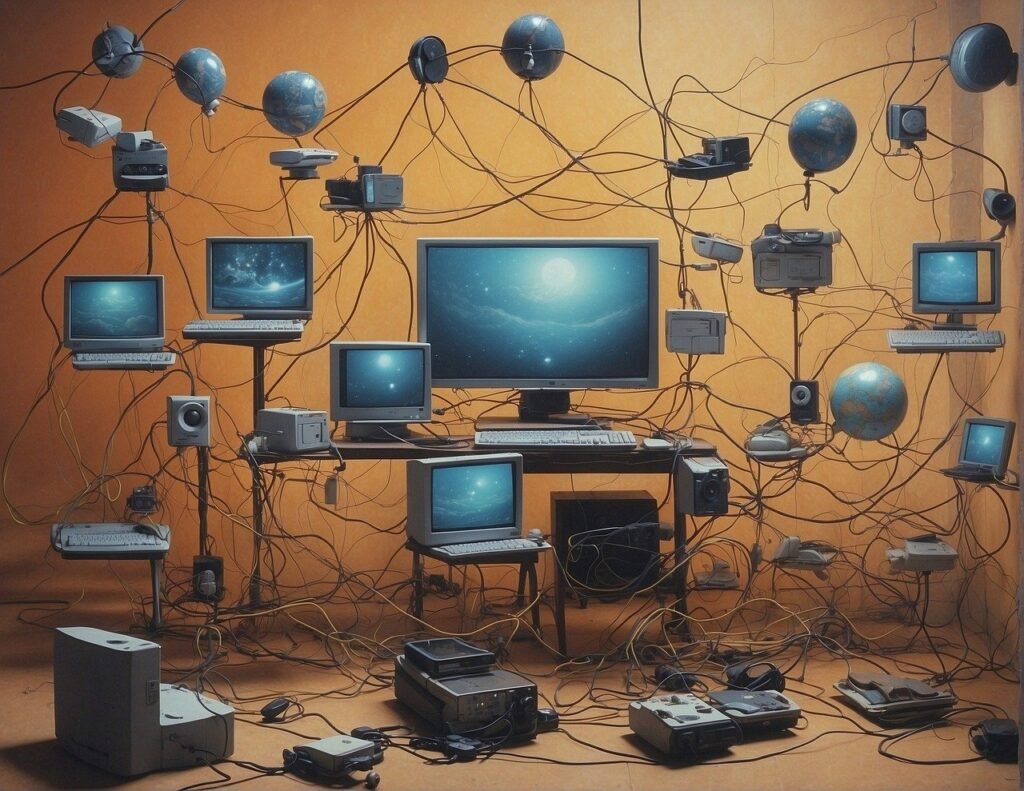
Docker networking is a critical component for ensuring seamless communication between containers. Essentially, it allows containers to interact with each other and with external networks, much like how devices connect and communicate over the internet. 🖧
Setting Up a Network for Container Communication
When you set up a network in Docker, you are essentially creating a virtual network that containers can join. This network facilitates communication between containers and isolates them from external networks. Here are some common Docker network drivers:
- Bridge: The default driver. Good for standalone containers.
- Host: Removes network isolation between the container and the Docker host.
- Overlay: Useful for multi-host networking, often used in Docker Swarm.
- None: Disables all networking for a container.
Exposing and Linking Containers
Exposing and linking containers is crucial for enabling communication and making services available to the outside world. Here’s how you can do it:
Exposing: To expose a container’s port to the host machine, you use the -p
flag. For example:
docker run -d -p 8080:80 my-web-app
This command maps port 8080 on the host to port 80 in the container.
Linking: Linking allows one container to communicate with another. For example:
docker run -d --name db-container my-database
docker run -d --name app-container --link db-container:db my-application
In this example, app-container
can communicate with db-container
using the alias db
.
While developing a web application with a frontend, a backend, and a database. You can set up the following network structure:
Container | Role | Exposed Ports |
---|---|---|
frontend | Handles user interface | 3000 |
backend | Processes business logic | 4000 |
database | Stores data | None |
By setting up appropriate networking and links, your frontend can communicate with the backend, and the backend can query the database, creating a cohesive application environment.
Best Practices for Using Docker in Web Development
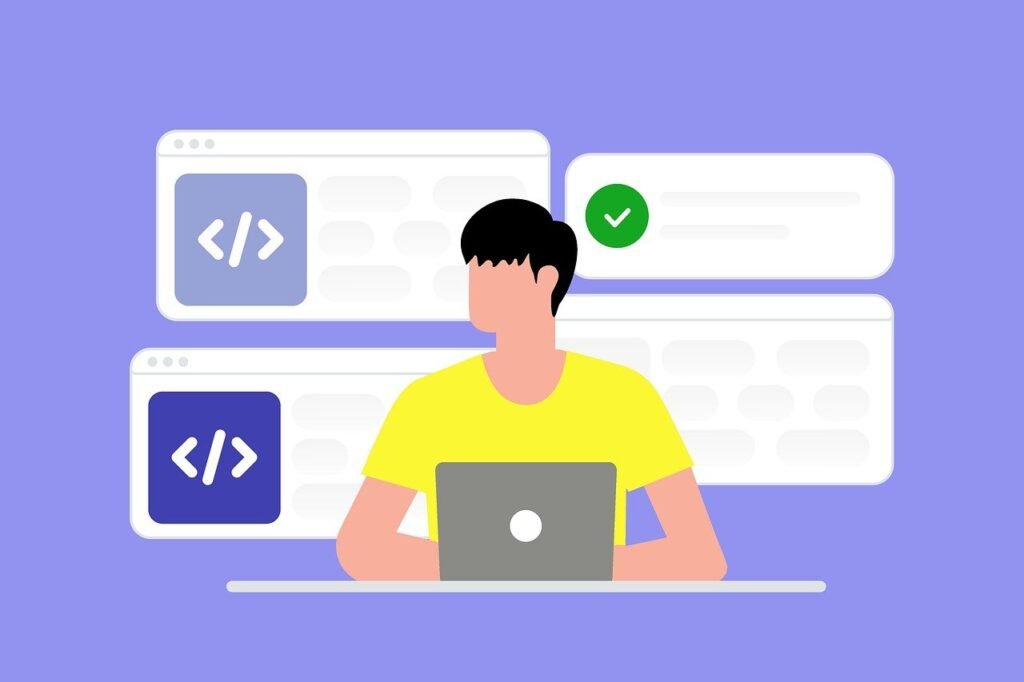
Docker has revolutionized the way developers build, ship, and run applications. By providing a consistent environment for development, Docker ensures that applications run seamlessly across different systems.
Writing Efficient Dockerfiles 📝
An efficient Dockerfile is crucial for building optimized Docker images. Here are some tips:
- Use Multi-Stage Builds: Multi-stage builds help in reducing the final image size by allowing you to copy only the necessary artifacts from the build stage.
- Leverage Official Base Images: Start with lightweight, official base images such as
alpine
to keep your image size small. - Minimize Layers: Combine related commands using
RUN
statements to reduce the number of layers in your image.
Example Dockerfile snippet:
FROM node:14-alpine AS build
WORKDIR /app
COPY package.json .
RUN npm install
COPY . .
RUN npm run build
FROM nginx:alpine
COPY --from=build /app/build /usr/share/nginx/html
Managing Dependencies 📦
Dependencies can bloat your Docker image if not managed properly. Here are some best practices:
- Use a Dependency Manager: Tools like
npm
oryarn
help in managing dependencies efficiently. - Ignore Unnecessary Files: Use
.dockerignore
to exclude files and directories that are not needed in the image. - Pin Versions: Always pin dependency versions to avoid unexpected issues due to updates.
Example .dockerignore
file:
node_modules*.logdist
Security Considerations 🔒
Security should be a top priority when working with Docker. Here are some recommendations:
- Use Non-Root Users: Run your containers as a non-root user to limit the damage in case of a security breach.
- Regularly Update Images: Keep your base images and dependencies up to date to include the latest security patches.
- Scan Images for Vulnerabilities: Use tools like
Clair
orTrivy
to scan your Docker images for known vulnerabilities.
Example of creating a non-root user in Dockerfile:
FROM node:14-alpine
RUN addgroup -S appgroup && adduser -S appuser -G appgroup
USER appuser
WORKDIR /app
COPY . .
RUN npm install
CMD ["npm", "start"]
Docker Debugging Issues
Debugging issues in Dockerized applications can be quite challenging. 🐳 One common problem is the discrepancy between the local development environment and the Docker container. This often leads to ‘works on my machine’ scenarios.
To tackle this, ensure that the Docker container mimics the production environment as closely as possible. Use Docker Compose to manage multi-container applications and create reproducible environments.
Handling Configuration and Environment Variables
Managing configuration and environment variables is crucial for application portability. 🌍 Incorrect handling can lead to security vulnerabilities or misconfigurations.
Use the .env
file to store environment variables. This file should not be included in the version control system to avoid exposing sensitive data. Here is an example:
DATABASE_URL=postgres://user:password@localhost:5432/dbname
SECRET_KEY=your_secret_key
Optimizing Docker Performance
Performance optimization in Docker is essential to ensure efficient resource usage and faster application response times. 🏎️ Common issues include high memory usage and slow container startup times.
To optimize performance:
- Use minimal base images, such as Alpine Linux, to reduce the container size.
- Leverage caching by structuring Dockerfile commands to minimize layer changes.
- Monitor container resource usage using tools like Docker Stats or cAdvisor.
Implementing these strategies can significantly improve the performance of your Dockerized applications.
Consider a Node.js application experiencing slow startup times. Here’s a step-by-step solution:
- Use a lightweight base image:
FROM node:14-alpine
- Leverage multi-stage builds to reduce the final image size.
- Cache dependencies to avoid repetitive installations:
COPY package*.json ./
followed byRUN npm install
By following these steps, the application’s startup time can be significantly reduced, leading to better performance and user experience.
Continuous Integration and Deployment(CI/CD)
Continuous Integration (CI) and Continuous Deployment (CD) are essential practices in modern software development. They ensure that code changes are automatically tested, integrated, and deployed, reducing manual efforts and minimizing errors. Docker, a popular containerization tool, plays a crucial role in enhancing CI/CD pipelines. Let’s explore how Docker can streamline these processes.
Docker simplifies the CI/CD workflow by providing a consistent environment for development, testing, and deployment.
Imagine a software development team working on a web application. They use Docker to containerize their application and integrate it with a CI/CD pipeline. Here’s a simplified overview of their workflow:
- Developers push code changes to a version control system (e.g., Git).
- A CI server (e.g., Jenkins) detects the changes and triggers a build process.
- A Docker image is built and tested within a Docker container.
- If tests pass, the Docker image is pushed to a container registry (e.g., Docker Hub).
- A CD tool (e.g., Kubernetes) pulls the Docker image from the registry and deploys it to a production environment.
Wrap UP
Docker has revolutionized the way we develop and deploy web applications, offering unparalleled consistency and efficiency. By containerizing your applications, you can ensure that your development environment mirrors production, reducing “works on my machine” issues and streamlining your workflow.
As you delve deeper into Docker, you’ll uncover a plethora of tools and best practices that can further enhance your development process.
FAQs
How does a Docker container differ from a virtual machine?
Containers share the host system’s kernel and resources more efficiently than virtual machines, which include a full operating system. This makes containers lightweight, faster to start, and more resource-efficient compared to virtual machines.
What is Docker Compose and how does it help in managing multi-container applications?
Docker Compose is a tool for defining and running multi-container Docker applications. With a docker-compose.yml
file, you can define services, networks, and volumes, and manage the entire application stack with simple commands.
How do I handle networking between Docker containers?
Docker provides networking features to connect containers. By default, Docker creates a bridge network that allows containers to communicate. You can create custom networks and configure containers to use them, ensuring secure and isolated communication between containers.
How can I integrate Docker with my CI/CD pipeline?
Docker can be integrated with CI/CD tools like Jenkins, GitLab CI, and GitHub Actions. By containerizing your application, you can automate builds, run tests in isolated environments, and deploy containers to production, ensuring a streamlined and consistent deployment process.