In the ever-evolving world of software development, speed and quality often seem at odds. Teams are under constant pressure to release features quickly while ensuring their applications remain bug-free and reliable. Enter continuous testing—a practice that validates code changes in real time, keeping the development pipeline flowing smoothly. At the heart of this process lies the integration of Selenium, a widely-used open-source tool for automating browser-based tests, and Jenkins, a powerhouse automation server for continuous integration and continuous deployment (CI/CD). Together, they form a dynamic duo that empowers teams to test efficiently and deliver with confidence.
This article dives deep into the process of integrating Selenium with Jenkins to enable continuous testing. Whether you’re a QA engineer crafting test scripts, a developer streamlining workflows, or a DevOps professional optimizing pipelines, this guide will walk you through every step. From setting up your environment to scaling your tests, we’ll cover it all with practical examples, tips, and troubleshooting advice. By the end, you’ll have the tools to transform your testing strategy. Let’s get started!
On This Page
Table of Contents
1. Understanding Selenium and Jenkins: A Quick Overview
Before we jump into the integration, let’s establish what these tools bring to the table and why combining them is a game-changer.
What is Selenium?
Selenium is an open-source framework designed for automating web browsers. It allows you to simulate user interactions—like clicking buttons, filling forms, or navigating pages—across browsers like Chrome, Firefox, and Edge. Its flagship component, Selenium WebDriver, gives developers precise control over browser automation.
- Example: Imagine testing a login page. With Selenium, you can write a script to enter a username, password, and click “Login,” then verify the welcome message—all automatically.
What is Jenkins?
Jenkins is an open-source automation server that orchestrates CI/CD pipelines. It automates repetitive tasks like building, testing, and deploying code, making it a cornerstone of modern DevOps practices.
- Example: After a developer pushes code to a repository, Jenkins can automatically build the app, run tests, and notify the team of the results.
Why Integrate Them?
Pairing Selenium with Jenkins bridges the gap between automated testing and continuous delivery. Selenium handles the “what” (browser tests), while Jenkins manages the “when” and “how” (scheduling and execution). Together, they ensure every code change is tested seamlessly, reducing manual effort and catching issues early.
2. Prerequisites for Integration
To integrate Selenium with Jenkins, you’ll need a solid foundation. Here’s what to prepare:
Requirement | Details | Example Tool/Versions |
---|---|---|
Java | Required for Selenium and Jenkins (both run on JVM). | JDK 11 or 17 |
Selenium WebDriver | Library for writing and running browser tests. | Selenium 4.x |
Jenkins | The CI/CD server to orchestrate tests. | Jenkins 2.426.x (LTS) |
Browser Drivers | Match these to your browser (e.g., ChromeDriver for Chrome). | ChromeDriver 123.x |
Test Framework | Optional but recommended for structuring tests. | TestNG or JUnit |
IDE | For writing Selenium scripts. | IntelliJ IDEA or Eclipse |
Git | For version control and integrating with Jenkins. | Git 2.43.x |
Steps to Set Up Your Environment
- Install Java: Download and configure the JDK. Verify with
java -version
in your terminal. - Download Jenkins: Get the
.war
file from jenkins.io and run it withjava -jar jenkins.war
. - Install Selenium: Add the Selenium dependency to your project (e.g., via Maven or pip for Python).
- Set Up Browser Drivers: Place drivers (e.g., ChromeDriver) in your system PATH.
- Prepare a Repository: Create a Git repo for your Selenium scripts.
Tip: Keep versions compatible—mismatched drivers or Selenium versions can cause headaches!
3. Writing Selenium Test Scripts for Automation
Your integration starts with solid Selenium scripts. Let’s craft a simple test to build on.
Example: Testing a Login Page
Here’s a Java script using Selenium WebDriver and TestNG:
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.Assert;
import org.testng.annotations.Test;
public class LoginTest {
@Test
public void testLogin() {
// Set ChromeDriver path
System.setProperty("webdriver.chrome.driver", "path/to/chromedriver");
WebDriver driver = new ChromeDriver();
// Open website
driver.get("https://example.com/login");
// Interact with elements
driver.findElement(By.id("username")).sendKeys("testuser");
driver.findElement(By.id("password")).sendKeys("password123");
driver.findElement(By.id("loginBtn")).click();
// Verify result
String welcomeText = driver.findElement(By.id("welcome")).getText();
Assert.assertEquals(welcomeText, "Welcome, Test User!");
// Clean up
driver.quit();
}
}
Best Practices
- Modularize: Break tests into reusable methods (e.g.,
login()
). - Use Waits: Add
WebDriverWait
to handle dynamic pages. - Parameterize: Store credentials in a config file, not hardcoded.
4. Configuring Jenkins for Continuous Testing
With your script ready, let’s set up Jenkins to run it.
Step-by-Step Setup
- Access Jenkins: Open
http://localhost:8080
after starting Jenkins. Complete the initial setup. - Install Plugins:
- Go to Manage Jenkins > Manage Plugins.
- Install Maven Integration (for Java projects) and Git Plugin.
- Create a New Job:
- Click New Item, name it (e.g., “Selenium-Test”), and select Freestyle Project.
Configuring the Job
- Source Code Management: Link your Git repo.
- Build Triggers: Enable “Poll SCM” (e.g.,
H/5 * * * *
for every 5 minutes). - Build Step: Add “Invoke top-level Maven targets” and set
test
as the goal.
5. Integrating Selenium Tests with Jenkins
Now, connect your Selenium script to Jenkins.
Using a Maven Project
- Create a
pom.xml
:
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>selenium-tests</artifactId>
<version>1.0</version>
<dependencies>
<dependency>
<groupId>org.seleniumhq.selenium</groupId>
<artifactId>selenium-java</artifactId>
<version>4.18.1</version>
</dependency>
<dependency>
<groupId>org.testng</groupId>
<artifactId>testng</artifactId>
<version>7.10.1</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.2.5</version>
</plugin>
</plugins>
</build>
</project>
- Push to Git: Commit your script and
pom.xml
to the repo. - Update Jenkins Job: Point the job to your repo and run
mvn test
.
Pipeline Example
For a Jenkins Pipeline:
pipeline {
agent any
stages {
stage('Test') {
steps {
git 'https://github.com/your-repo/selenium-tests.git'
sh 'mvn test'
}
}
}
}
6. Running and Monitoring Tests in Jenkins
Time to execute and track your tests!
Running the Job
- Click Build Now in Jenkins.
- Watch the console output for real-time logs.
Viewing Results
- Add TestNG Plugin: Install it to see detailed test reports.
- Post-Build Action: Add “Publish TestNG Results” and point to
testng-results.xml
.
Example Output: A green build means all tests passed; red indicates failures with stack traces.
Tip: Archive artifacts (e.g., screenshots) by adding a post-build step like “Archive the artifacts” (*.png
).
7. Scaling Your Testing Pipeline
For larger projects, scale up with these techniques:
Parallel Execution
- Add Nodes: Configure Jenkins agents to run tests on multiple machines.
- Example: Split tests across 3 nodes to cut runtime from 15 minutes to 5.
Selenium Grid
- Setup: Run a Grid Hub and Nodes (e.g.,
java -jar selenium-server-4.jar hub
). - Update Script: Use
RemoteWebDriver
to target the Grid.
URL gridUrl = new URL("http://localhost:4444/wd/hub");
WebDriver driver = new RemoteWebDriver(gridUrl, new ChromeOptions());
8. Troubleshooting Common Integration Challenges
Things don’t always go smoothly—here’s how to fix common issues:
Issue | Cause | Solution |
---|---|---|
Driver Mismatch | Browser/driver version conflict | Update driver to match browser |
Test Timeout | Slow page load | Increase WebDriverWait timeout |
Build Failure | Missing dependency | Check pom.xml or PATH settings |
Tip: Use Jenkins logs to pinpoint errors—search for keywords like “exception” or “failed”.
9. Benefits of Selenium-Jenkins Integration
Why bother? Here’s the payoff:
- Faster Feedback: Catch bugs within minutes of a commit.
- Improved Coverage: Automate hundreds of tests effortlessly.
- Team Efficiency: Free QA to focus on exploratory testing.
Real-World Example: A fintech app reduced regression testing from 2 days to 4 hours, boosting release frequency by 50%.
WrapUP
Integrating Selenium with Jenkins for continuous testing is more than a technical upgrade—it’s a mindset shift toward quality-driven development. By automating browser tests within a CI/CD pipeline, you empower your team to deliver robust software faster, with fewer surprises. This guide has walked you through the essentials: from writing scripts to scaling pipelines, all grounded in practical steps and examples. Now it’s your turn—set up your environment, run your first build, and experience the difference. The road to seamless testing starts here. Happy automating!
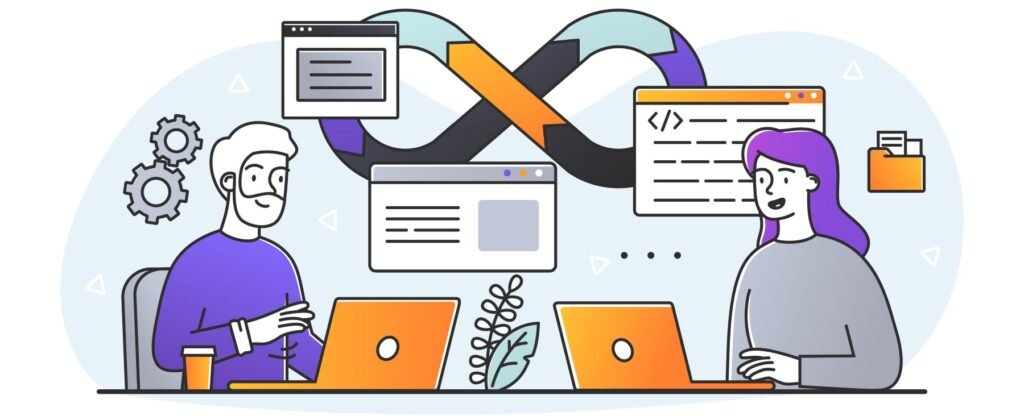
FAQs
What is the easiest way to integrate Selenium with Jenkins?
Answer: The simplest method is to create a Selenium test script (e.g., using Java or Python), push it to a Git repository, and configure a Jenkins freestyle job. Install the Maven Integration or Git Plugin, link your repo, and set the build step to run your tests (e.g., mvn test
). This setup takes less than 30 minutes for beginners.
Why should I use Selenium with Jenkins for testing?
Answer: Combining Selenium with Jenkins automates browser testing within a CI/CD pipeline, catching bugs early, speeding up feedback loops, and reducing manual effort. It ensures your web app works across browsers every time code changes—perfect for agile teams.
How do I fix Selenium tests failing in Jenkins?
Answer: Common fixes include:
Ensuring browser drivers (e.g., ChromeDriver) match your browser version.
Adding explicit waits (e.g., WebDriverWait
) for dynamic elements.
Checking Jenkins logs for dependency errors and updating your pom.xml
.
Run a test build manually to isolate the issue.
Can Jenkins run Selenium tests automatically?
Answer: Yes! Configure a Jenkins job with a build trigger like “Poll SCM” (e.g., H/5 * * * *
for every 5 minutes) or “Build Periodically.” Link it to your Selenium scripts in a Git repo, and Jenkins will execute them on schedule or after code commits.
What tools do I need to integrate Selenium with Jenkins?
Answer: You’ll need:
Java (for both tools).
Selenium WebDriver (for test scripts).
Jenkins (installed locally or on a server).
Browser drivers (e.g., ChromeDriver).
Optional: TestNG/JUnit and Maven/Git.
Download them, set up your PATH, and you’re ready!
How long does it take to set up Selenium with Jenkins?
Answer: For a basic integration, expect 1-2 hours: 30 minutes to install Jenkins and prerequisites, 20 minutes to write a Selenium script, and 30 minutes to configure a Jenkins job. Scaling to advanced setups (e.g., Selenium Grid) may add a few hours.
Does Selenium Grid work with Jenkins for cross-browser testing?
Answer: Absolutely! Set up a Selenium Grid Hub and Nodes, then update your Jenkins job to use RemoteWebDriver
targeting the Grid (e.g., http://localhost:4444/wd/hub
). This runs tests across multiple browsers simultaneously, managed by Jenkins.
How do I view Selenium test results in Jenkins?
Answer: Install the TestNG Results Plugin or use JUnit reports. Add a post-build action in Jenkins to publish results (e.g., point to testng-results.xml
). You’ll see pass/fail stats and detailed logs on the job dashboard.
What are the benefits of continuous testing with Selenium and Jenkins?
Answer: Key perks include:
Faster bug detection (within minutes of commits).
Higher test coverage across browsers.
Less manual testing, freeing up QA time.
Example: A team cut regression testing from 2 days to 4 hours.