A REST API (Representational State Transfer Application Programming Interface) is a way for different software systems to communicate over the internet. Think of it as a bridge that allows various applications to exchange data seamlessly using standard web protocols like HTTP.
On This Page
Table of Contents
How Do REST APIs Work?
REST APIs operate by adhering to a set of principles and utilizing HTTP methods to perform operations:
- GET: Retrieves data from a server.
- POST: Submits new data to a server.
- PUT: Updates existing data on a server.
- DELETE: Removes data from a server.
When a client sends an HTTP request to a server, the server processes the request and sends an appropriate response, often in JSON or XML format.
Key Principles of REST Architecture
REST APIs follow several key principles that ensure smooth and effective communication:
Principle | Description |
---|---|
Statelessness | Each request from a client to a server must contain all the information needed to understand and process the request. |
Uniform Interface | A consistent and standardized approach to interacting with resources, simplifying interactions between the client and server. |
Client-Server Separation | The client and server are separated, allowing for more scalability and flexibility in the development process. |
Cacheability | Responses can be cached to improve performance and reduce server load. |
Layered System | An architecture composed of hierarchical layers that provide a modular approach to API design. |
Examples
Imagine you use a weather application on your phone. When you check the weather, the app sends a GET request to a server’s REST API that returns current weather data for your location:
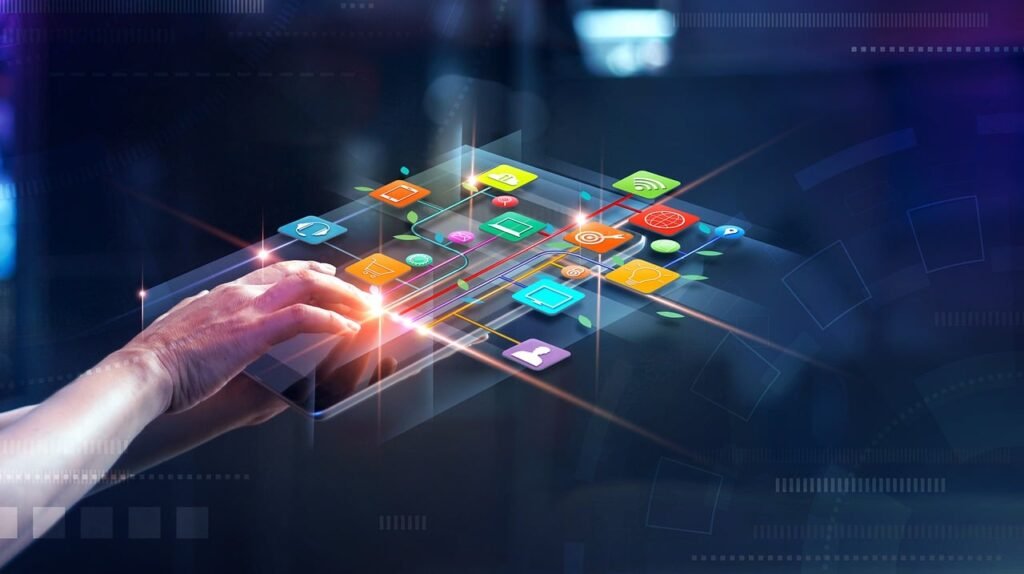
GET /weather?location=NewYork
The server processes this request and sends back a JSON response with the weather details:
{"temperature": "22°C", "condition": "Sunny"}
In this way, REST APIs make it possible for applications to interact with web services efficiently and effectively, enhancing user experiences across various platforms.
Core Components of REST APIs
REST (Representational State Transfer) APIs are widely used protocols in web development, enabling communication between client and server applications. They use standard HTTP methods and are renowned for their simplicity and scalability.
Endpoints and Resources
Endpoints are specific paths mapped to resources that can be manipulated using HTTP methods. For instance, /api/users
could be an endpoint to access user details.
HTTP Methods
HTTP methods are actions performed on the resources:
- GET – Retrieve data from an endpoint. Example:
GET /api/users
to get a list of users. - POST – Create new resources. Example:
POST /api/users
to add a new user. - PUT – Update existing resources. Example:
PUT /api/users/1
to update user with ID 1. - DELETE – Remove resources. Example:
DELETE /api/users/1
to delete user with ID 1.
Status Codes and Responses
Status codes indicate the result of an HTTP request:
Status Code | Meaning |
---|---|
200 | OK – The request was successful. |
201 | Created – A new resource has been created successfully. |
400 | Bad Request – The request could not be understood or was missing required parameters. |
404 | Not Found – The requested resource could not be found. |
500 | Internal Server Error – An error occurred on the server. |
For example, when you request GET /api/users
successfully, the server may return status code 200
with a list of users in the response body.
REST vs. Other API Styles
The most common styles include REST, SOAP, and GraphQL. Choosing the right API style can significantly impact your project .
REST vs. SOAP
REST (Representational State Transfer) and SOAP (Simple Object Access Protocol) are the most well-known API styles. Let’s explore their differences:
Aspect | REST | SOAP |
---|---|---|
Communication | HTTP/HTTPS | HTTP, SMTP, and more |
Data Format | JSON, XML | XML |
Performance | Faster | Slower |
Security | OAuth, HTTPS | WS-Security standard |
📌 Example: Use REST when you need lightweight, stateless communication, ideal for web applications. Use SOAP for higher security needs, such as financial services.
REST vs. GraphQL
Another popular comparison is between REST and GraphQL. Here’s how they differ:
- Data Fetching: REST uses multiple endpoints for different data types, while GraphQL uses a single endpoint to query data.
- Flexibility: GraphQL allows clients to request specific data fields, reducing over-fetching or under-fetching issues.
📌 Example: Use REST for simpler projects or when you need caching. Use GraphQL for complex systems needing precise data fetching, such as social media apps.
When to Use REST APIs
REST APIs are versatile and widely adopted. Use them when:
- Building web applications
- Needing stateless communication
- Requiring easy scalability
💡 Note: REST APIs are developer-friendly, making them a great choice for most standard web services requirements.
RESTful API Design
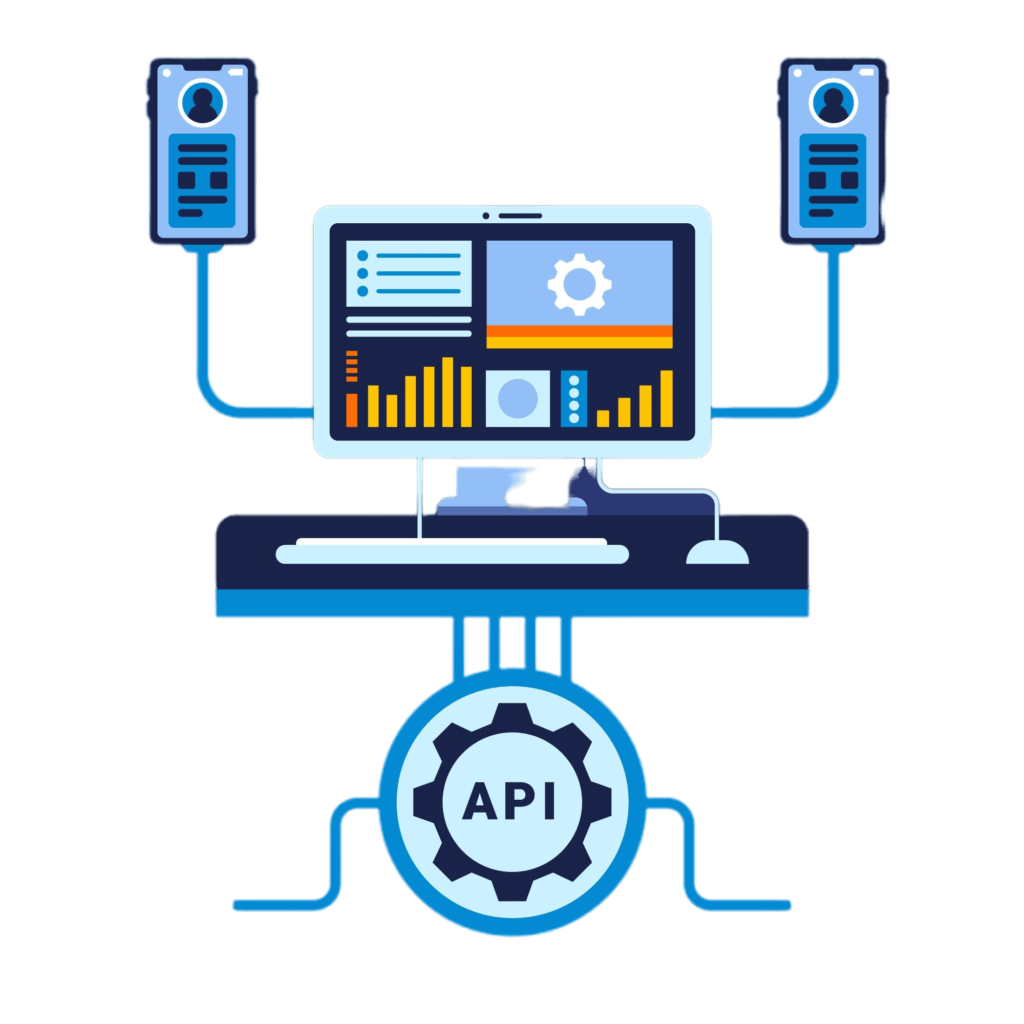
Designing a RESTful API involves creating a system that allows clients and servers to communicate effectively using stateless operations. Here, we will discuss key aspects like designing resource URIs, choosing appropriate HTTP methods, implementing stateless operations, and handling error responses.
Designing Resource URIs
Resource URIs should be intuitive and meaningful. This helps clients understand the resources available. Some best practices include:
- Use nouns (e.g., `/users` instead of `/getUsers`)
- Utilize hierarchy (e.g., `/users/{userId}/posts`)
- Avoid actions in URIs
Example URI: /users/123/orders
where `123` is the user ID, and `/orders` indicates the orders resource of that user.
Choosing Appropriate HTTP Methods
HTTP methods should match the intended operation:
HTTP Method | Operation |
---|---|
GET | Retrieve data |
POST | Create a new resource |
PUT | Update a resource |
DELETE | Delete a resource |
Example: To retrieve a user’s orders, use GET /users/123/orders
.
Implementing Stateless Operations
RESTful APIs are stateless, meaning each request must contain all the information needed for processing. This improves scalability since the server does not need to store session information.
Example: Include authentication tokens in the header of every request instead of relying on server-side sessions.
Handling Error Responses
Proper error handling ensures that clients understand what went wrong and how to fix it. Use appropriate HTTP status codes to indicate errors:
- 400 Bad Request: The request could not be understood by the server due to malformed syntax.
- 401 Unauthorized: The request requires user authentication.
- 404 Not Found: The server has not found anything matching the URI.
- 500 Internal Server Error: The server encountered an unexpected condition.
Example error response:
{ "error": "User not found", "status": 404}
Authentication and Security in REST APIs
REST APIs are a crucial part of modern web services, providing a way for applications to communicate. However, ensuring secure communication is critical. Let’s delve into common authentication methods and best practices to secure REST APIs.
Common Authentication Methods
OAuth: OAuth is a widely used protocol that allows secure authorization. It provides tokens rather than credentials, enabling applications to access resources effectively.
API Keys: API keys are simple codes passed in by the application to authenticate the API call. They work as a unique identifier and a secret token combined into one.
Best Practices for Securing REST APIs
Here are some best practices to secure REST APIs:
- Use HTTPS: Always use HTTPS to encrypt the data sent between the client and server.
- Validate Input: Ensure that all input is validated to prevent injection attacks.
- Implement Rate Limiting: Control the number of requests a user can make to an API in a given time period to prevent abuse.
- Use Strong Authentication: Employ strong and reliable authentication methods like OAuth.
Example: Rate Limiting and Throttling
Consider a popular social media platform that provides its API to developers to create apps. To ensure fair usage and prevent abuse, they implement rate limiting. A typical rate limit may look like this:
Endpoint | Allowed Calls per Minute |
---|---|
/users | 100 |
/posts | 200 |
By setting these limits, the platform ensures stability and availability of its services for all users.
Why Versioning is Important
API versioning is crucial for maintaining seamless interactions between different software systems. Just like software updates, APIs need to evolve without disrupting the services they provide. Imagine if your favorite app suddenly stopped working because the API it relies on changed without notice! Versioning helps avoid such surprises by providing a structured way to handle updates and changes.
Strategies for API Versioning
Several strategies can be employed for API versioning, and choosing the right one depends on your specific needs:
- URI Versioning: Embed the version number in the URI path, e.g.,
/api/v1/resource
. - Query Parameters: Include the version number as a query parameter, e.g.,
/api/resource?version=1
. - Custom Headers: Use HTTP headers to specify the version, e.g.,
Accept: application/vnd.company+json;version=1
. - Content Negotiation: Leverage ‘Accept’ headers to manage different versions based on content types.
Examples
Let’s look at a real-world example. Suppose a company offers a User API for accessing user data. Initially, they have /api/v1/users
. As the API evolves, they introduce new features and make changes, leading to the release of /api/v2/users
. This ensures that existing applications using /api/v1/users
continue to work without disruption.
Version | Endpoint | Description |
---|---|---|
v1 | /api/v1/users | Initial version of the User API. |
v2 | /api/v2/users | Introduced new filtering options and additional fields. |
Managing Deprecation
Deprecation is an essential aspect of API lifecycle management. When an API version becomes outdated, it’s important to communicate this to your users. Consider the following steps for managing API deprecation:
- 🚀 Announce Deprecation: Inform users through official channels about the deprecation timeline.
- 📆 Provide a Transition Period: Allow sufficient time for users to migrate to the newer version.
- 🔧 Maintain Compatibility: Ensure backward compatibility during the transition period to avoid disruptions.
By implementing these strategies, you’ll keep your API services robust and reliable over time.
Common Challenges and Solutions
Creating a REST API is a crucial task for many businesses and developers. Despite its widespread use, several common challenges can hinder the performance and scalability of REST APIs. Lets explore these challenges with their solutions.
Handling Performance Issues
Performance is a key aspect of any API. One common issue is the latency caused by network delays. Here are some solutions:
- Use Caching: Implementing caching mechanisms can significantly reduce latency.
- Optimize Queries: Ensure your database queries are optimized to fetch data quickly.
- Compress Responses: Use compression algorithms to send smaller response payloads.
For example, using Redis for caching frequently accessed data can improve response times dramatically.
Managing Large Data Sets
Working with large data sets can be challenging. It often leads to performance degradation and high memory usage. Here are some solutions:
- Pagination: Breaking down data into smaller chunks makes it manageable.
- Filtering: Allow users to filter the data they need, reducing the data load.
- Lazy Loading: Load data on-demand rather than loading everything at once.
For example, if your API returns a list of users, implementing pagination can help in reducing the load on the server.
Ensuring Scalability
Scalability is essential for handling an increase in user requests. Here are some strategies to ensure scalability:
- Load Balancing: Distribute incoming requests across multiple servers to prevent a single point of failure.
- Microservices Architecture: Break down the monolithic architecture into smaller, manageable services.
- Auto-Scaling: Use cloud providers’ auto-scaling features to adjust resources based on traffic.
For example, using AWS Elastic Load Balancer can help in distributing the traffic evenly across multiple instances, ensuring scalability.
FAQs
How does a REST API work?
A REST API works by defining a set of endpoints (URLs) that represent resources. Clients make HTTP requests to these endpoints using methods such as GET, POST, PUT, or DELETE to interact with the resources. The server responds with the requested data or a status message.
What are the key principles of REST architecture?
The key principles of REST architecture include stateless communication, use of standard HTTP methods, resource-based URIs, and the representation of resources in a format like JSON or XML. REST also emphasizes scalability and simplicity.
How is REST different from SOAP?
REST and SOAP are both web service protocols, but REST is simpler and more lightweight, using standard HTTP methods and data formats like JSON. SOAP (Simple Object Access Protocol) is more complex, requiring XML for messaging and supporting additional features like security and transactions.
How do I handle authentication in REST APIs?
Authentication in REST APIs can be handled using various methods, including API keys, OAuth tokens, and Basic Authentication. The choice of method depends on the security requirements of your application.
What tools can I use to test REST APIs?
Popular tools for testing REST APIs include Postman, Curl, and Insomnia. These tools allow you to send HTTP requests, inspect responses, and automate API testing.
How can I secure my REST API?
Securing your REST API involves implementing authentication (e.g., OAuth, API keys), using HTTPS to encrypt data, validating and sanitizing inputs, and applying rate limiting and throttling to prevent abuse.