The WebSocket protocol has emerged as a cornerstone technology in modern web applications, facilitating real-time, bi-directional communication over a persistent socket connection. Lets delve into the essential aspects of WebSockets, including their fundamental concepts, the API’s structure, and how developers can effectively implement these protocols within their applications. Understanding WebSockets is crucial for creating responsive and interactive user experiences.
On This Page
Table of Contents
Introduction to WebSockets
What are WebSockets?
A WebSocket is a communication protocol that enables full-duplex communication channels over a single TCP connection. This innovative technology allows for real-time, event-driven interactions between a client and a server, facilitating data exchanges without relying on multiple HTTP connections.
Key Features of WebSockets
- Instantaneous Exchanges: Unlike traditional HTTP, which operates on a request-response model, WebSockets allow instantaneous data exchanges at any moment.
- Standardization: The protocol was standardized in 2011 by the Internet Engineering Task Force (IETF) as RFC 6455.
- Wide Browser Support: Major web browsers, including Google Chrome, Mozilla Firefox, and Safari, fully support WebSockets, making them an ideal choice for developers.
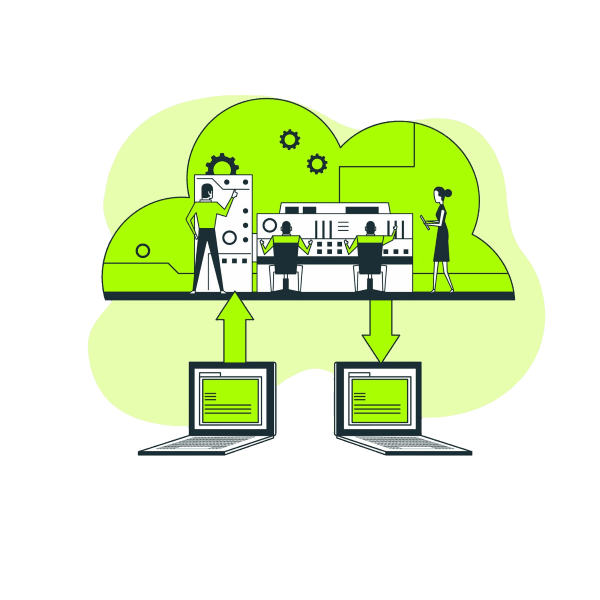
Applications of WebSockets
WebSockets have rapidly gained traction in contemporary web applications that demand immediate updates. Their applications include:
Application | Description |
---|---|
Live Chats | Enable real-time communication between users. |
Online Gaming | Facilitate smooth interactions and instant updates during gameplay. |
Collaborative Platforms | Support simultaneous editing and interactions among users. |
Importance of the WebSocket Protocol
The Websocket protocol is vital for applications necessitating real-time communication. It addresses the constraints associated with traditional HTTP protocols, enabling more efficient data transmission.
With websockets, both the client and server can independently send messages. This capability offers several benefits:
- Reduced latency
- Enhanced overall efficiency
- Persistent single connection
- Lower bandwidth consumption
Websockets are particularly advantageous for:
Application Type | Importance of Real-Time Communication |
---|---|
Instant Messaging | Timely updates for seamless conversations |
Multiplayer Gaming | Real-time interaction among players |
Live Data Feeds | Immediate updates on data changes |
The protocol’s design leads to lower overhead compared to establishing multiple HTTP connections. This reduction translates to improved performance, making websockets a preferred choice for developers building interactive and responsive web applications.
Differences Between WebSockets and HTTP
WebSockets and HTTP differ fundamentally in their communication frameworks, each serving specific purposes based on application requirements.
Here are some essential distinctions between these two protocols:
- State Management: HTTP is a stateless protocol, treating each client request independently, while WebSockets maintain a persistent connection.
- Connection Method: HTTP requires multiple connections for bi-directional exchanges; WebSockets utilize a singular connection for real-time communication.
- Message Initiation: WebSockets allow messages to be sent at any time, in contrast to HTTP, which only responds to client-initiated requests.

These distinction means that, unlike HTTP, which requires multiple connections for bi-directional exchanges, WebSockets utilize a singular connection. Furthermore, WebSockets allow messages to be sent at any time, in contrast to HTTP, which only responds to requests initiated by the client. These inherent differences render WebSockets more suitable for applications demanding real-time updates and interactions.
These distinction means that, unlike HTTP, which requires multiple connections for bi-directional exchanges, WebSockets utilize a singular connection. Furthermore, WebSockets allow messages to be sent at any time, in contrast to HTTP, which only responds to requests initiated by the client. These inherent differences render WebSockets more suitable for applications demanding real-time updates and interactions.
WebSocket API Overview
Understanding the WebSocket API
The WebSocket API provides a standardized interface for creating and managing WebSocket connections within web applications. This enables seamless communication between the client and the server, making it a crucial tool for developers.
Key Features of the WebSocket API
Understanding the key functionalities of the WebSocket API is crucial . Here are some standout features:
- Full-Duplex Communication: This essential feature enables simultaneous message sending and receiving, significantly improving user experience.
- Event-Driven Architecture: The API is event-driven, allowing developers to respond effectively to connection events and incoming messages asynchronously.
- Data Transmission Flexibility: It supports both text and binary data transmission, accommodating various types of information exchange.
- Browser Compatibility: Designed for compatibility across different browsers, ensuring seamless integration into diverse web applications.
Comparing WebSocket with Traditional Methods
Feature | WebSocket API | Traditional HTTP |
---|---|---|
Communication Type | Full-Duplex | Half-Duplex |
Event Handling | Event-Driven | Request-Response |
Data Types Supported | Text and Binary | Text Only |
Compatibility | Cross-Browser | Limited |
With advancements in web technologies, the WebSocket API has become a cornerstone for developers looking to implement efficient real-time communication in their applications. Its numerous benefits make it an attractive choice for modern web development.
WebSocket API Methods and Events
The WebSocket API comprises numerous essential methods and events that facilitate seamless communication between clients and servers. This protocol allows for the establishment of a persistent connection that can handle full-duplex communication, enabling real-time interactions.
- websocket.send(): Utilized for conveying data over the connection.
- websocket.close(): Essential for properly closing the WebSocket connection to prevent resource leaks.
WebSocket API utilizes an event-driven architecture that empowers developers to create responsive applications. The crucial events include:
Event | Description |
---|---|
onopen | Triggered when the connection is established. |
onmessage | Occurs when a message is received from the server. |
onerror | Fires when an error occurs during communication. |
onclose | Indicates that the connection has been closed. |
By leveraging these methods and events, developers can create applications that react efficiently to real-time data changes, thereby enhancing user experience and interactivity.
Implementing WebSockets in Web Applications
Setting Up a WebSocket Server
To set up a WebSocket server, developers must select a programming language and framework that seamlessly supports the WebSocket protocol. Popular choices include:
- Node.js: Utilizes the
ws
library, which provides built-in functionalities for creating a WebSocket server. - Python: Offers frameworks like Flask-SocketIO for efficient WebSocket handling.
- Java: The Spring Framework enables seamless WebSocket integration.
Managing the WebSocket Handshake
The initial phase involves managing the WebSocket handshake, where the server responds to an HTTP request to upgrade the connection. This process is vital for establishing a reliable connection. Once a WebSocket connection is established, the server can:
- Efficiently handle multiple client connections.
- Facilitate bi-directional communication between clients and the server.
Ensuring Reliability and Performance
Furthermore, robust error handling and effective connection management are paramount to ensure reliability and performance, especially during high load conditions that could lead to a WebSocket close. Here is a summary table of these aspects:
Feature | Description |
---|---|
Error Handling | Implement mechanisms to gracefully catch and manage errors. |
Connection Management | Monitor active connections and recycle resources efficiently. |
High Load Handling | Utilize load balancing and scaling techniques for optimum performance. |
By following these guidelines, developers can create a robust and efficient WebSocket server capable of handling real-time data exchange effectively.
Once a WebSocket connection is established, the server can efficiently handle multiple client connections and facilitate bi-directional communication. Furthermore, robust error handling and effective connection management are paramount to ensure reliability and performance, especially during high load conditions that could lead to a websocket close.
Creating a WebSocket Client
Creating a WebSocket client necessitates utilizing the WebSocket API to establish a connection with a WebSocket server. This innovative technology allows for real-time communication between clients and servers.
Developers achieve the connection by instantiating a new WebSocket
object with the server’s URL. Here’s a simple overview of the process:
- Instantiate a new WebSocket object
- Use the server’s URL as a parameter
- Wait for the connection to open
Upon successfully opening the WebSocket connection, the client can send messages using the send()
method and listen for incoming messages through the onmessage
event handler.
It is crucial for the client to effectively manage connection events. These events include:
Event | Description |
---|---|
onopen | Triggered when the connection is successfully opened. |
onclose | Triggered when the connection is closed. |
onerror | Triggered when an error occurs. |
These events help provide feedback to users and maintain the application’s state throughout its lifecycle.
Handling WebSocket Connections
Developers must implement event listeners to manage various states and messages throughout the connection lifecycle. Below, we outline the essential actions to ensure smooth communication:
- Connection Opened: Define clear actions when a WebSocket connection opens.
- Message Received: Establish protocols for handling received messages.
- Connection Closed: Delineate steps to take when the connection closes.
In addition to state management, proactive error handling is essential. This involves:
- Monitoring network interruptions
- Addressing server errors
- Implementing strategies for graceful error recovery
Enhancing User Experience Through Connection State Management is paramount. An application that can:
- Gracefully handle reconnections
- Effectively manage disconnections
Will significantly boost user experience and overall reliability. Below is a comparison table illustrating key differences in connection handling:
Feature | With Proper Handling | Without Proper Handling |
---|---|---|
User Experience | Smooth and continuous | Frequent interruptions |
Error Management | Proactive response | Reactive fixes |
Reliability | High stability | Frequent failures |
Using WebSockets for Interactive Web Applications
Real-Time Data Transmission
Websockets excel in real-time data transmission, enabling instantaneous communication between clients and servers. This capability is vital for applications requiring timely updates, such as:
- Live chat
- Online gaming
- Collaborative tools
With websockets, data can be sent and received without the latency typical of traditional HTTP requests, fostering a seamless user experience. Some of the primary advantages include:
- Reduced latency in data communication
- Persistent connection for uninterrupted updates
- Enhanced interactivity for users
The protocol’s ability to maintain a persistent connection allows applications to push updates to clients the moment they occur, creating more interactive and engaging experiences for users through the use of web sockets.
Examples of WebSockets in Action
WebSockets find extensive application in multiple scenarios, some of which include:
- Live Sports Applications: They deliver real-time scores and updates directly to users, enhancing the viewing experience.
- Collaborative Editing Tools: Users can observe changes made by others instantaneously, significantly improving collaboration efficiency.
- Online Gaming Platforms: Players engage in real-time interactions, creating a more immersive gaming experience.
Comparative Overview of WebSockets :
Platform | Use of WebSockets |
---|---|
Live Sports | Real-time scores and updates |
Collaborative Tools | Instantaneous change updates |
Online Gaming | Real-time player interaction |
These examples underscore the versatility and effectiveness of WebSockets in developing dynamic web applications. By facilitating efficient socket communication, they promise a more engaging user experience across different platforms.
Best Practices for Using WebSockets
When implementing WebSockets, developers must adhere to best practices to achieve optimal performance and security. The following key practices are essential:
- Establish secure connections using the wss:// protocol to encrypt data transmitted between clients and servers.
- Implement robust error handling and reconnection logic to enhance application reliability.
- Utilize message compression to minimize bandwidth usage and improve performance.
- Effectively monitor and manage server resources to handle multiple concurrent WebSocket connections.
One of the most crucial aspects of WebSocket implementation is establishing secure connections. Using the wss:// protocol is essential to:
- Encrypt data, ensuring confidentiality during transmission.
- Protect against man-in-the-middle attacks.
Robust error handling and reconnection logic play a significant role in maintaining reliability. Highlighted advantages include:
Aspect | Importance |
---|---|
Error Handling | Ensures smooth user experience by managing unexpected issues. |
Reconnection Logic | Allows applications to recover from network failures seamlessly. |
Finally, monitoring and managing server resources effectively is crucial for handling multiple concurrent WebSocket connections, particularly in high-traffic situations.
FAQs
Q: What are WebSockets used for in modern web applications?
WebSockets are used for real-time communication between a client and a server, enabling bidirectional data transfer with lower latency compared to traditional HTTP requests. This is particularly useful in applications like chat systems, online gaming, and live data feeds.
Q: How does the websocket connection close code function in a WebSocket protocol?
The websocket connection close code is utilized to indicate the reason for closing a WebSocket connection. It helps both the client and server understand why the connection was terminated, which is essential for debugging and maintaining the application.
Q: What is the significance of the server certificate in establishing a WebSocket connection?
The server certificate plays a crucial role in securing a WebSocket connection, especially when using the secure WebSocket protocol (wss://
). If the server certificate can’t be verified, the client may refuse to establish a connection, ensuring data integrity and security.
Q: Can you explain the process of using WebSocket for a real-time application?
To implement a real-time application using WebSocket, a new WebSocket connection is established from the client to the server. Once connected, the client can send a WebSocket message to the server, and the server can respond, allowing for continuous data exchange without the need to poll the server.
Q: What are some common use cases for WebSockets?
Common use cases for WebSockets include live chat applications, online multiplayer games, stock price updates, live sports scores, and collaborative tools that require real-time updates, all of which benefit from the low latency and persistent connection that WebSockets provide.
Q: How does the WebSocket closing handshake process work?
The WebSocket closing handshake is started when either the client or the server decides to close the connection. A closing frame is sent, which includes a websocket connection close reason. The other party must respond with its own closing frame to complete the handshake, ensuring a graceful termination of the connection.
Q: What are WebSocket extensions, and how do they enhance the protocol?
WebSocket extensions are options that can be negotiated between the client and server to enhance the capabilities of the WebSocket protocol. These extensions can provide additional features such as compression or message fragmentation, which can improve performance and efficiency in data transmission.
Q: How can a developer ensure the scalability of WebSocket connections?
To scale WebSocket connections effectively, developers can implement load balancing strategies, use multiple WebSocket servers, and utilize cluster management tools. This approach allows for distributing the client load across several servers, ensuring that the application can handle an increasing number of simultaneous connections.
Q: What considerations should be made regarding server-side implementation of WebSockets?
When implementing WebSockets on the server side, developers must consider the handling of multiple WebSocket connections simultaneously, managing the websocket lifecycle, ensuring security with server certificates, and efficiently processing incoming and outgoing messages to maintain a responsive user experience.