Imagine you are packing for a trip. You have two options to fit more items into your suitcase: you can either remove unnecessary items (like extra pairs of shoes, heavy jackets, and non-essential accessories) or you can compress everything by vacuum-sealing your clothes to reduce space.
This analogy perfectly illustrates the concept of minification and compression in web development. Both techniques aim to optimize files, improve website speed, and enhance user experience, but they work differently. While minification removes unnecessary characters from code, compression encodes the data in a more efficient format to reduce file size.
On This Page
Table of Contents
What is Minification?
Minification is the process of removing unnecessary characters from source code without changing its functionality. This includes:
- Whitespace
- Comments
- Line breaks
- Redundant code structures
By doing so, the code becomes more compact, which reduces file size and improves page load speed.
Consider a simple JavaScript file before and after minification:
Before Minification:
function greet(name) {
console.log("Hello, " + name + "!"); // Greeting message
}
greet("Alice");
After Minification:
function greet(n){console.log("Hello, "+n+"!")}greet("Alice");
The function remains the same, but unnecessary spaces and comments have been removed, making the file smaller and faster to load.
Tools for Minification
- JavaScript: UglifyJS, Terser
- CSS: CSSNano, CleanCSS
- HTML: HTMLMinifier
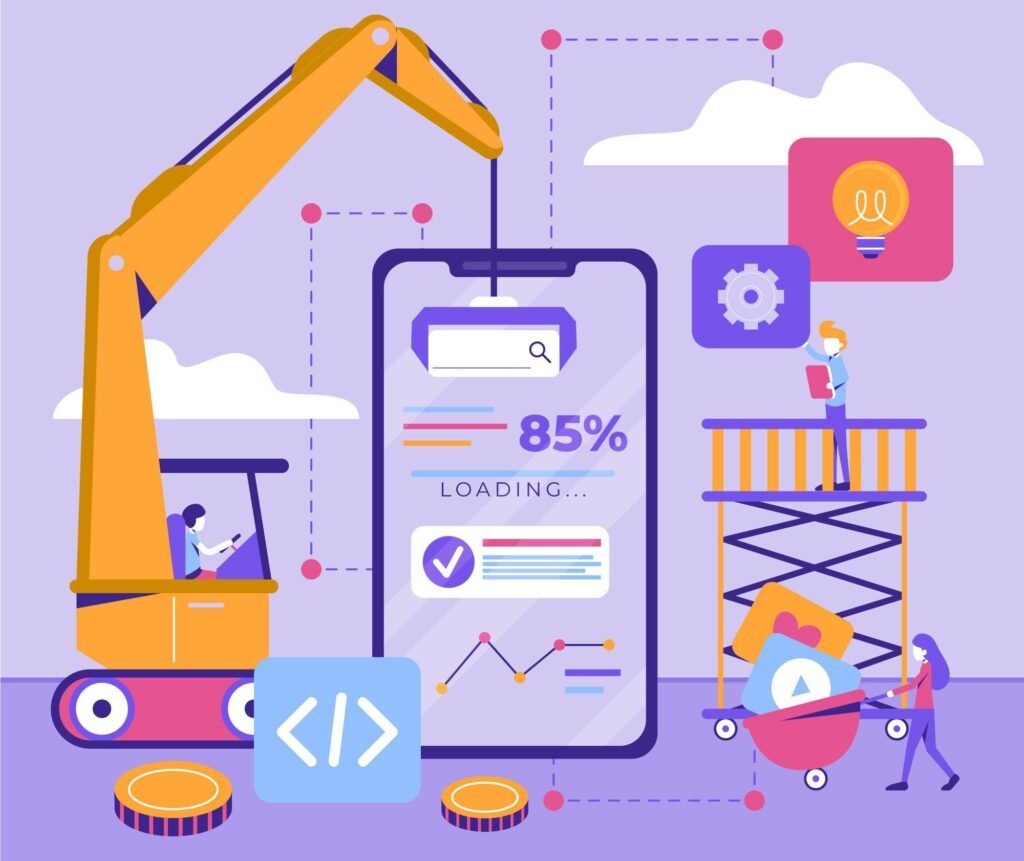
What is Compression?
Compression is the process of reducing the size of files by encoding them in a more efficient format. Unlike minification, compression modifies the file structure, making it more compact but still readable by browsers.
Types of Compression
Compression can be categorized into:
- Lossy compression: Some data is discarded for higher compression (e.g., JPEG, MP3)
- Lossless compression: No data is lost (e.g., Gzip, Brotli)
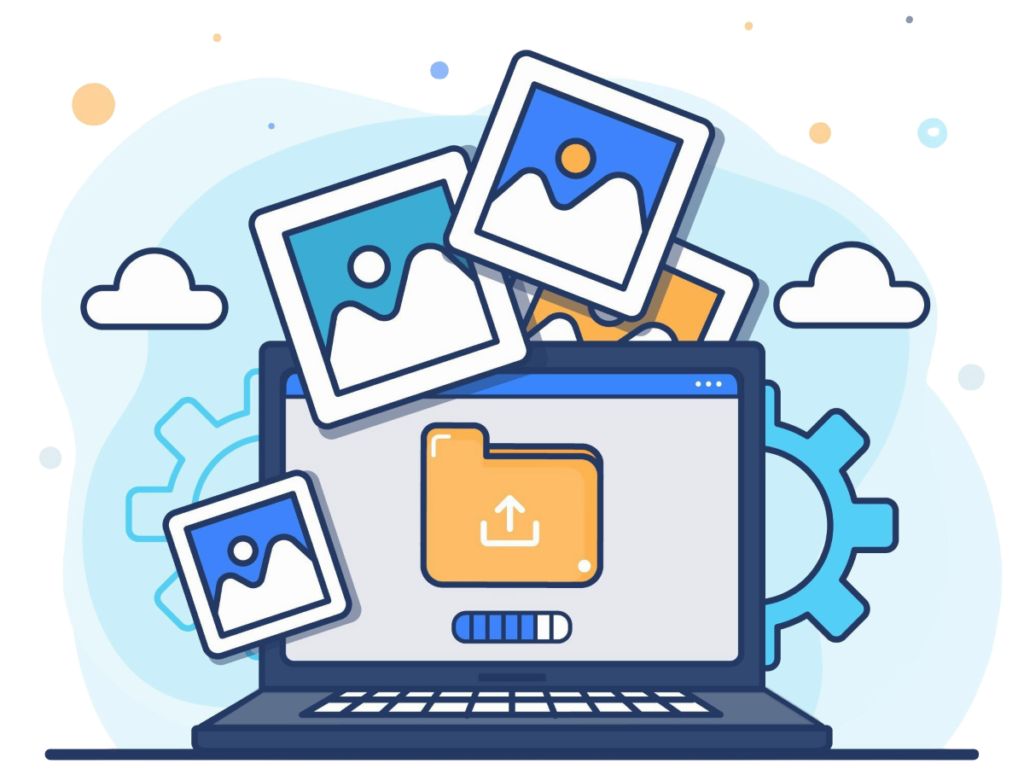
Example of Compression
Consider a CSS file before and after Gzip compression:
Before Compression: (Size: 5KB)
body {
font-family: Arial, sans-serif;
background-color: #f4f4f4;
}
After Compression (Gzip Applied): (Size: 2KB)
The file is encoded efficiently, making it smaller for transfer.
Example of Gzip Compression in a Web Server
You can enable Gzip compression in an Apache web server using the following configuration:
<IfModule mod_deflate.c>
AddOutputFilterByType DEFLATE text/html text/plain text/xml text/css application/javascript
</IfModule>
For Nginx:
gzip on;
gzip_types text/css application/javascript;
This ensures that the server automatically compresses specified file types before sending them to the browser.
Common Compression Tools
Minification vs. Compression: Key Differences
Both minification and compression aim to reduce file size and enhance web performance, but they operate differently. Minification simplifies source code, while compression encodes files more efficiently for transmission. Below is a detailed comparison:
Key Differences Between Minification and Compression
Feature | Minification | Compression |
---|---|---|
Purpose | Removes unnecessary characters | Encodes data in an efficient format |
Effect | Reduces file size by eliminating spaces, comments, and redundant code | Further reduces file size after minification using encoding techniques |
Types | JavaScript, CSS, HTML | Gzip, Brotli, Deflate |
Processing | Done at the source code level | Done at the server level before transmission |
Readability | Still readable and executable | Encoded and requires decoding for use |
Performance Impact | Faster parsing and execution | Reduced bandwidth and faster page load times |
Example Tools | UglifyJS, Terser, CSSNano | Gzip, Brotli, Deflate |
Detailed Breakdown of Differences
- How They Work
- Minification simply removes unnecessary characters without affecting code execution.
- Compression encodes files into a smaller format that needs to be decompressed by the browser.
- Impact on Performance
- Minification improves the efficiency of web files by making them lightweight.
- Compression significantly reduces bandwidth usage, making data transmission faster.
- Implementation
- Minification is applied before files are deployed.
- Compression happens on the server-side before files are sent to the client.
- Compatibility
- Minified files are still readable and editable.
- Compressed files require browsers or servers that support decoding (e.g., Gzip-compatible servers).
By leveraging both minification and compression together, developers can ensure maximum performance improvements, reduced loading times, and an overall smoother user experience.
Feature | Minification | Compression |
---|---|---|
Purpose | Removes unnecessary characters | Encodes data more efficiently |
Effect | Reduces file size | Further reduces file size after minification |
Types | JavaScript, CSS, HTML | Gzip, Brotli, Deflate |
Processing | Done at the source code level | Done at the server level |
Readability | Still readable | Encoded and needs decoding |
When to Use Minification vs Compression?
Choosing between minification and compression depends on the specific needs of your website or application. Each technique serves a unique purpose, and understanding when to use them can significantly improve web performance.
Use Minification When:
- You want to optimize front-end assets like JavaScript, CSS, and HTML files.
- The focus is on removing unnecessary characters (whitespace, comments, extra semicolons, etc.) to reduce file size without changing how the file works.
- You need to maintain human readability while still reducing file size.
- You are working with static files that are frequently updated and donโt require runtime compression.
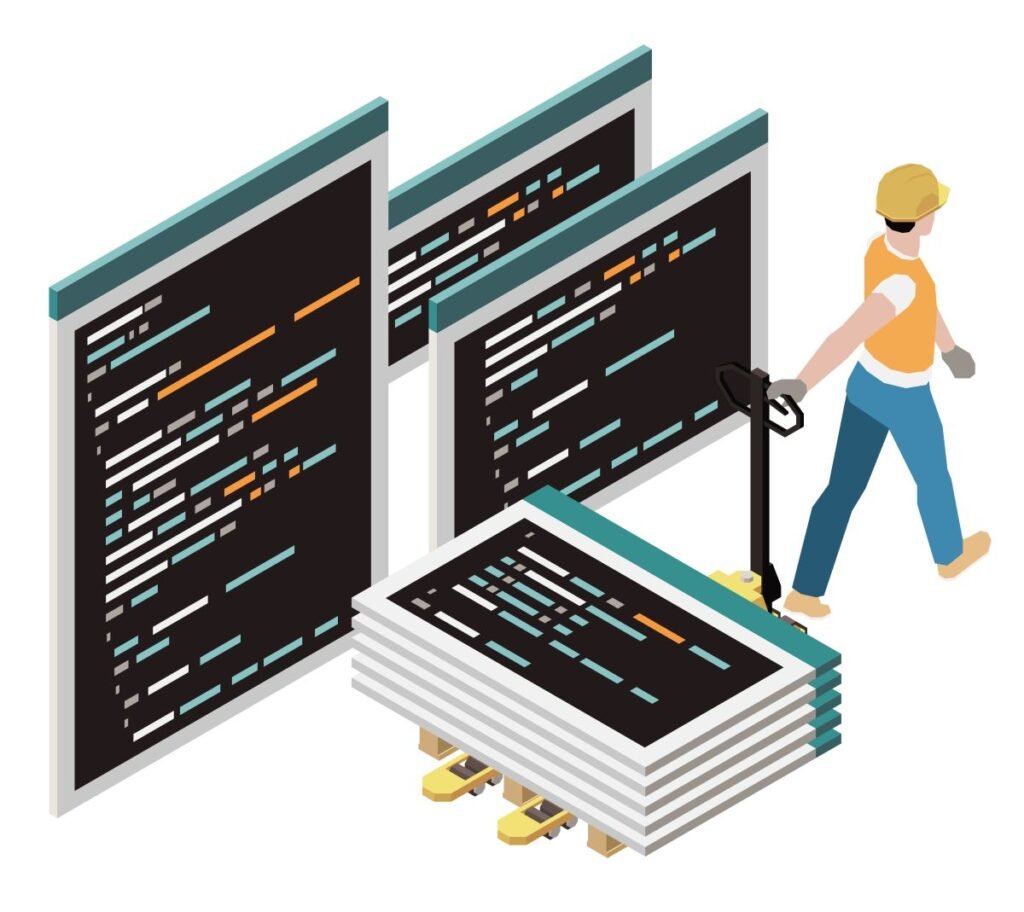
Use Compression When:
- You want to compress a wide range of file types, including HTML, CSS, JavaScript, JSON, XML, fonts, and images.
- The goal is to reduce file transfer size over the network to improve page load times.
- You are serving files over the web and want to minimize bandwidth usage.
- The server supports compression algorithms like Gzip or Brotli, which can automatically compress and decompress files without affecting the original source code.
- You are dealing with large files that need efficient encoding for faster loading and performance.
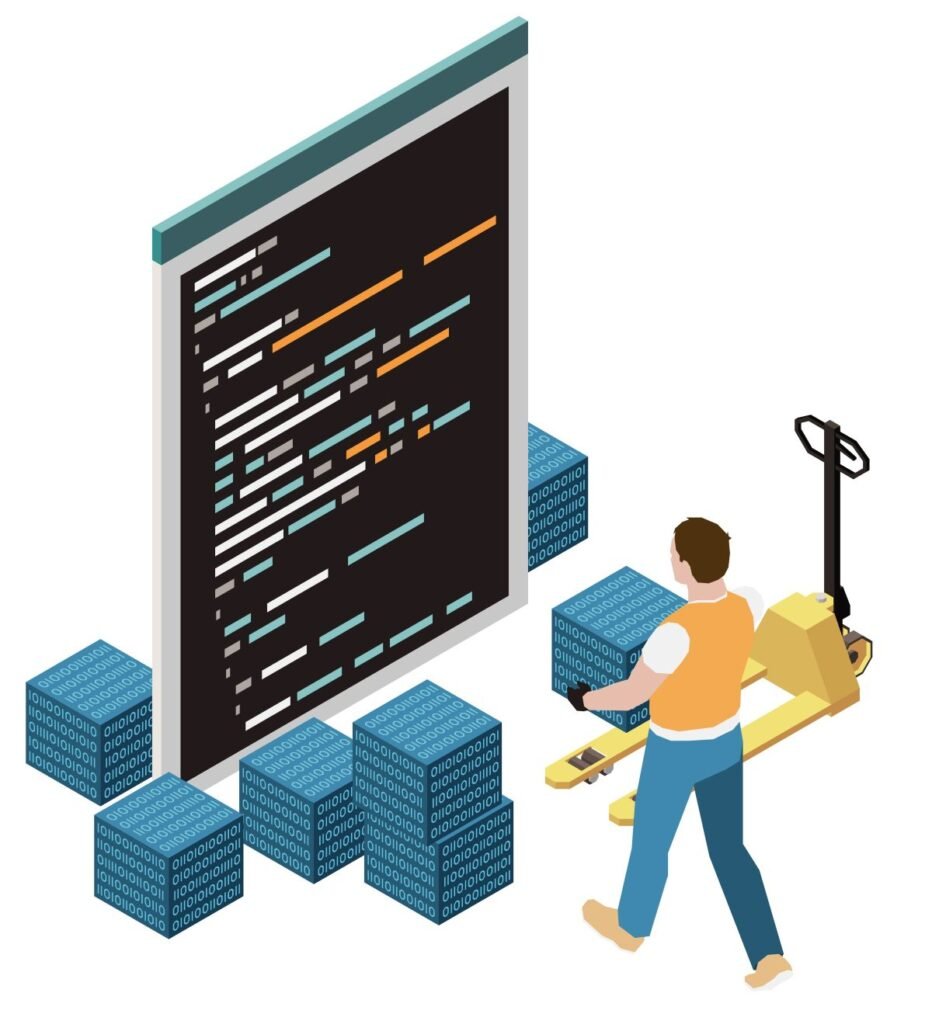
When to Use Both Together?
In most cases, the best approach is to combine both minification and compression for optimal performance. Here’s how:
- First, minify your files using tools like Terser (JavaScript), CSSNano (CSS), or HTMLMinifier (HTML). This reduces the file size at the source code level.
- Next, enable compression (Gzip or Brotli) at the server level to further compress the already minified files.
- Serve the compressed files via a Content Delivery Network (CDN) to ensure faster delivery and reduced latency.
Performance Impact: Minification vs Compression
Technique | Average File Size Reduction | Suitable for |
---|---|---|
Minification | 20-30% | JavaScript, CSS, HTML |
Compression | 50-70% | All file types (text, images, fonts, etc.) |
Both Combined | 40-80% | Best for overall performance |
By implementing both strategies, websites can see 40-60% faster load times, improved SEO rankings, and reduced server load.
Combining Minification and Compression
For maximum performance, both minification and compression should be used together.
Best Practices for Web Performance
- Minify JavaScript, CSS, and HTML files.
- Enable Gzip or Brotli compression on the server.
- Use Content Delivery Networks (CDNs) to serve files faster.
- Optimize caching strategies to store compressed files.
Real-World Performance Insights
Studies show that:
- Minification alone reduces file size by ~20-30%.
- Compression (Gzip) reduces file size by an additional 50-70%.
- Websites using both techniques see a 40-60% improvement in load time.
WrapUP
Both minification and compression are essential for web performance optimization. While minification removes unnecessary code, compression efficiently encodes files to reduce size further. By implementing both, developers can significantly improve website speed, reduce bandwidth usage, and enhance user experience.
Next time you optimize a website, think of it as packing for a trip: Remove what you donโt need (minification), then compress everything tightly (compression). ๐
FAQs
What is minification?
Minification is the process of removing unnecessary characters (e.g., spaces, comments, and line breaks) from source code without changing its functionality to reduce file size.
What is compression?
Compression is the process of encoding files into a more efficient format to reduce their size before being transmitted over the internet.
What is the main difference between minification and compression?
Minification removes redundant characters in the code, while compression re-encodes files in a more efficient format.
Do minification and compression achieve the same result?
No. Minification reduces file size by removing extra characters, whereas compression reduces size by changing the file encoding.
Can minification be reversed?
No, once minified, a file cannot be perfectly restored to its original format without additional tools. However, code beautifiers can improve readability.
Can compression be reversed?
Yes, compressed files can be decompressed automatically by browsers and servers to restore their original content.
Which one improves website speed more: minification or compression?
Compression usually provides a greater reduction in file size compared to minification, but using both together gives the best results.
Should I use both minification and compression?
Yes! Minifying before compressing maximizes file size reduction and improves web performance.
Which files should be minified?
You should minify JavaScript, CSS, and HTML files.
Which files should be compressed?
You should compress all text-based files, including HTML, CSS, JavaScript, JSON, XML, and SVG.
How much does minification reduce file size?
Minification can reduce file size by 20-30%, depending on the amount of whitespace, comments, and redundant code.
How much does compression reduce file size?
Compression (e.g., Gzip, Brotli) can reduce file size by 50-70%, depending on the file type and compression algorithm used.
Which minification tools are best for JavaScript?
Popular JavaScript minification tools include UglifyJS, Terser, and Google Closure Compiler.
Which compression format is better: Gzip or Brotli?
Brotli generally provides better compression ratios than Gzip, but Gzip is more widely supported.
Can minification break my website?
Yes, if done incorrectly. Minifying JavaScript may remove important line breaks or semicolons, causing errors.
Can compression break my website?
No, compression does not alter functionality. However, improper server configuration may prevent files from decompressing properly.
Can images be minified?
No, images cannot be minified but can be compressed using tools like WebP, JPEG, or PNG optimizers.
Does minification affect SEO?
Yes! Minified files load faster, which improves Core Web Vitals and can positively impact SEO rankings.