Imagine you’re working on a web app that tracks customer orders for a growing e-commerce business. As users interact with your app, you need to manage customer data, process orders, and generate insightful reports. What powers all of this behind the scenes? SQL — the language that speaks to databases. From startups to enterprise-level companies, SQL is everywhere.
In this article, we’ll explore 10 essential SQL queries that every developer should know. Whether you’re retrieving customer orders, calculating totals, or joining tables, mastering these queries will elevate your database management skills. We’ll provide real-life scenarios, code examples, and tips for writing efficient SQL code.
On This Page
Table of Contents
Retrieving All Data from a Table (SELECT Query)
Think of this query as the starting point for any data exploration. When you want to see all the data in a table, you use a SELECT
query.
Scenario:
You run a customer management system and want to see all customer records stored in the customers
table.
SQL Example:
SELECT * FROM customers;
Explanation:
SELECT
tells the database to retrieve data.*
represents all columns.FROM customers
specifies the table name.
Output Table Example:
customer_id | name | join_date | |
---|---|---|---|
1 | Jane Doe | jane@example.com | 2023-01-01 |
2 | John Smith | john@example.com | 2023-02-15 |
Tip: Avoid using SELECT *
in production systems. Instead, specify only the columns you need (e.g., SELECT name, email FROM customers;
).
Filtering Results Using WHERE Clauses
Filtering is crucial when you need only specific records.
Scenario:
You want to find customers who joined after January 1, 2023.
SQL Example:
SELECT * FROM customers
WHERE join_date > '2023-01-01';
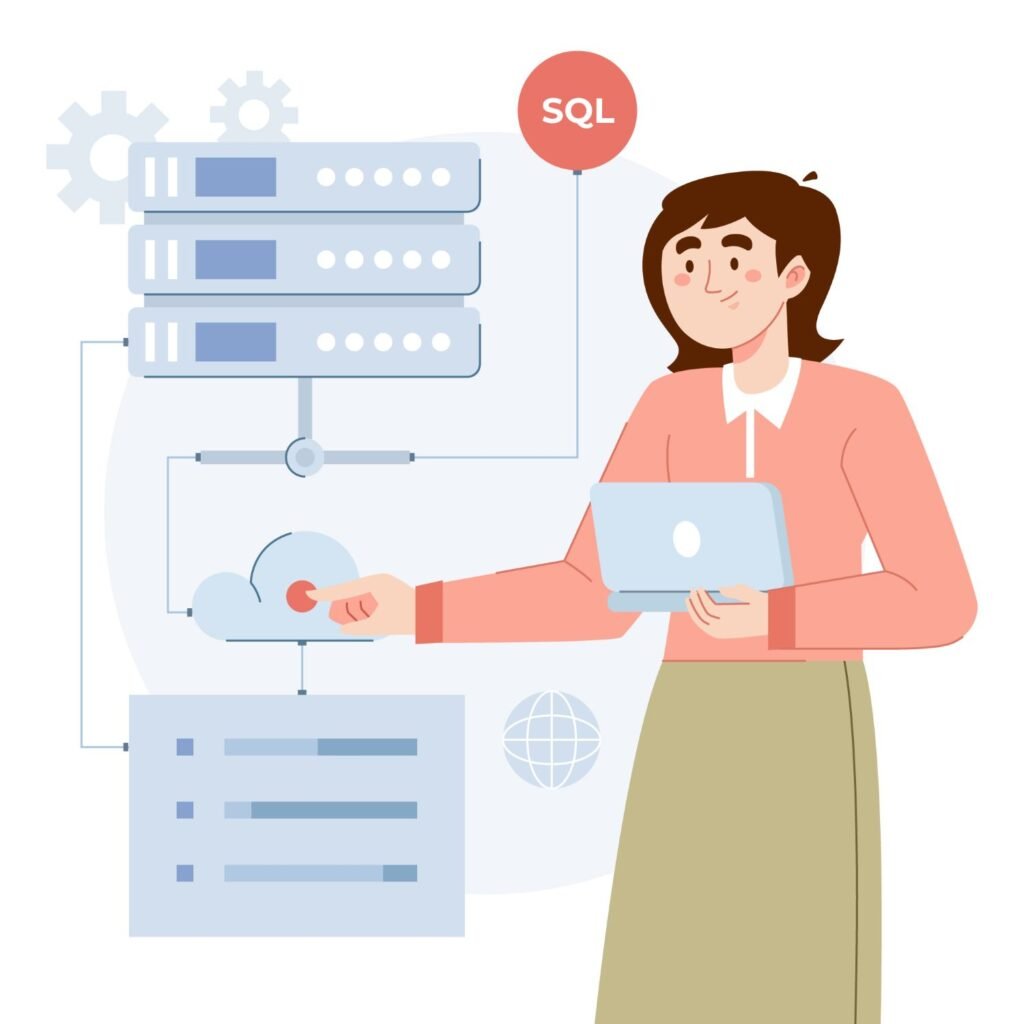
Explanation:
WHERE
specifies a condition.join_date > '2023-01-01'
filters out customers who joined after January 1, 2023.
Output Table Example:
customer_id | name | join_date | |
---|---|---|---|
2 | John Smith | john@example.com | 2023-02-15 |
Tip: Combine multiple conditions using AND
, OR
, or NOT
for complex filtering.
Sorting Data with ORDER BY
Sorting helps you organize results in a meaningful way.
Scenario:
You want to see customers sorted by their join_date
, with the most recent first.
SQL Example:
SELECT * FROM customers
ORDER BY join_date DESC;
Explanation:
ORDER BY
specifies the sorting column.DESC
means descending order (newest to oldest).
Output Table Example:
customer_id | name | join_date | |
---|---|---|---|
2 | John Smith | john@example.com | 2023-02-15 |
1 | Jane Doe | jane@example.com | 2023-01-01 |
Tip: Use ASC
(ascending order) to sort from oldest to newest.
Limiting Results with LIMIT and OFFSET
Sometimes, you only need a small sample of data.
Scenario:
You want to display the first two customers from the customers
table.
SQL Example:
SELECT * FROM customers
LIMIT 2;
Explanation:
LIMIT 2
retrieves only the first two rows.
Tip: Use OFFSET
to skip a certain number of rows. For example:
SELECT * FROM customers
LIMIT 2 OFFSET 1;
This retrieves the second and third rows.
Grouping and Aggregating Data (GROUP BY, HAVING)
Aggregation allows you to calculate totals, averages, and more.
Scenario:
You want to count the number of customers who joined on each date.
SQL Example:
SELECT join_date, COUNT(*) AS customer_count
FROM customers
GROUP BY join_date;
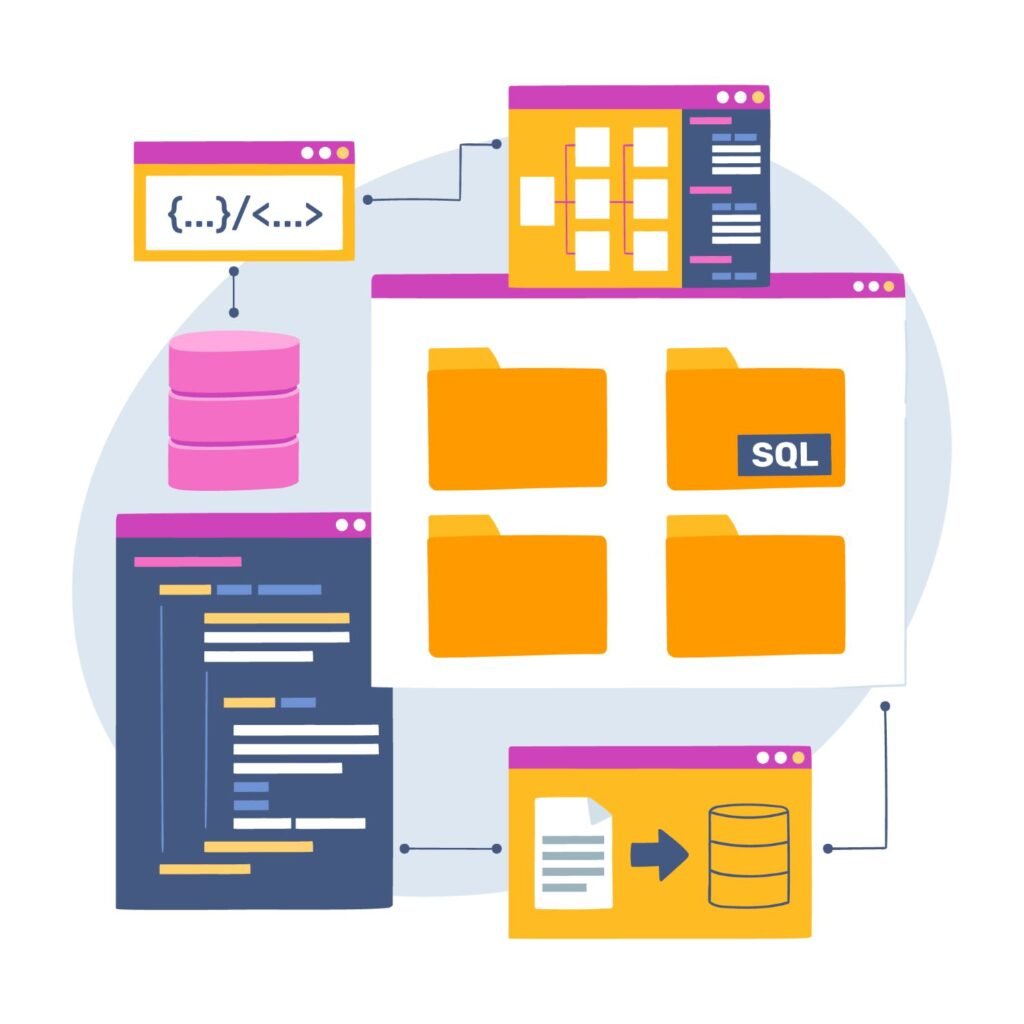
Explanation:
GROUP BY
groups rows with the samejoin_date
.COUNT(*)
counts the number of rows in each group.
Output Table Example:
join_date | customer_count |
---|---|
2023-01-01 | 1 |
2023-02-15 | 1 |
Tip: Use HAVING
to filter grouped results:
SELECT join_date, COUNT(*) AS customer_count
FROM customers
GROUP BY join_date
HAVING COUNT(*) > 1;
Joining Tables (INNER JOIN, LEFT JOIN, RIGHT JOIN, FULL OUTER JOIN)
Joins allow you to combine data from multiple tables.
Scenario:
You have two tables: customers
and orders
. You want to see all orders along with customer names.
SQL Example (INNER JOIN):
SELECT customers.name, orders.order_id, orders.order_date
FROM customers
INNER JOIN orders ON customers.customer_id = orders.customer_id;
Explanation:
INNER JOIN
retrieves rows with matchingcustomer_id
in both tables.
Output Table Example:
name | order_id | order_date |
---|---|---|
Jane Doe | 101 | 2023-03-01 |
John Smith | 102 | 2023-03-02 |
Tip: Use LEFT JOIN
to include all customers, even those without orders.
Inserting New Records (INSERT INTO)
Adding data is a common task.
Scenario:
You want to add a new customer to the customers
table.
SQL Example:
INSERT INTO customers (name, email, join_date)
VALUES ('Alice Johnson', 'alice@example.com', '2023-03-10');
Tip: You can insert multiple rows at once:
INSERT INTO customers (name, email, join_date)
VALUES
('Bob Lee', 'bob@example.com', '2023-03-11'),
('Charlie Kim', 'charlie@example.com', '2023-03-12');
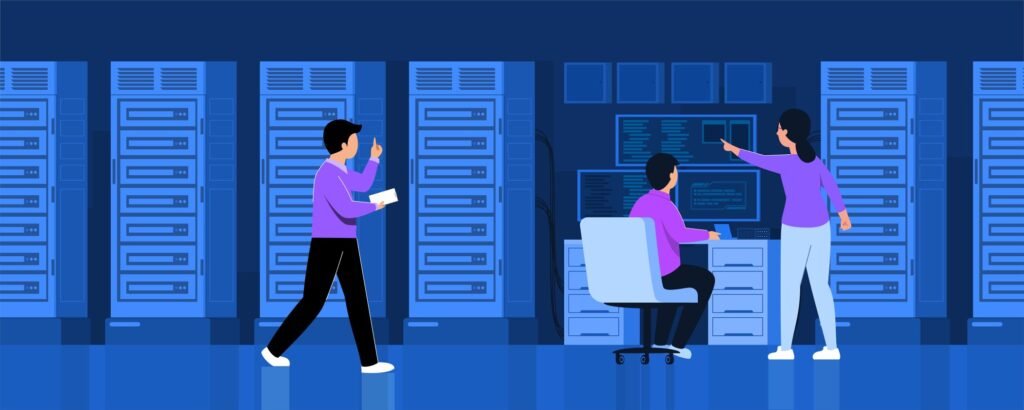
Updating Existing Data (UPDATE Query)
Updating records is essential for maintaining accurate data.
Scenario:
You want to update Jane Doe’s email.
SQL Example:
UPDATE customers
SET email = 'jane.doe@newdomain.com'
WHERE name = 'Jane Doe';
Tip: Always include a WHERE
clause to avoid updating all rows by mistake.
Deleting Records (DELETE Query)
Deleting records should be done with caution.
Scenario:
You want to delete a customer who requested account removal.
SQL Example:
DELETE FROM customers
WHERE name = 'Bob Lee';
Tip: Use LIMIT
to delete only a few rows if unsure.
Subqueries and Nested Queries
Subqueries allow you to nest one query inside another.
Scenario:
You want to find customers with orders placed after March 1, 2023.
SQL Example:
SELECT * FROM customers
WHERE customer_id IN (
SELECT customer_id FROM orders
WHERE order_date > '2023-03-01'
);
Tip: Use subqueries to simplify complex conditions.
WrapUP
Mastering these top 10 SQL queries will make you more efficient at managing databases and retrieving meaningful insights. Whether you’re working on web apps or data analysis, these queries are your building blocks. Keep practicing, and you’ll be well on your way to becoming a SQL pro.
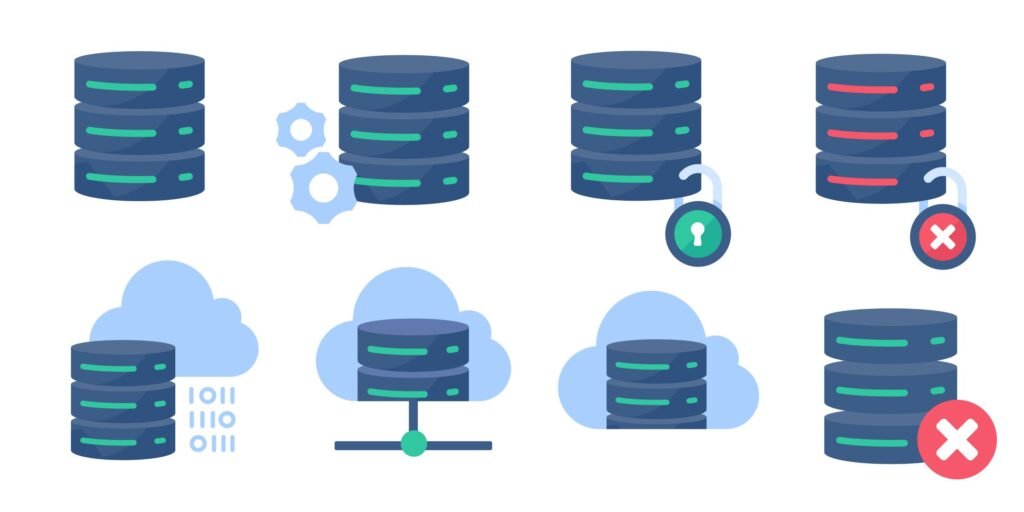
Additional Resources:
FAQs
What is SQL, and why is it important?
SQL is a language used to interact with relational databases, making it essential for managing and querying data efficiently.
What is the difference between SELECT *
and selecting specific columns?
SELECT *
retrieves all columns, while specifying columns retrieves only the data you need, improving performance and readability.
How can I filter NULL values in SQL?
Use IS NULL
or IS NOT NULL
in your WHERE
clause to handle NULL values.
What is the purpose of ORDER BY
?
ORDER BY
sorts query results in ascending (ASC
) or descending (DESC
) order.
How do I sort by multiple columns?
List multiple columns in ORDER BY
, separated by commas. Example: ORDER BY last_name, first_name
.
What is the difference between INNER JOIN and LEFT JOIN?
INNER JOIN
returns only matching rows, while LEFT JOIN
returns all rows from the left table and matching rows from the right.
How can I safely update records in SQL?
Always use a WHERE
clause to limit affected rows, and back up your data before making updates.
What are SQL aggregate functions?
Aggregate functions like SUM
, AVG
, COUNT
, MIN
, and MAX
calculate values across multiple rows.
What is the difference between HAVING
and WHERE
?
WHERE
filters rows before grouping, while HAVING
filters grouped results.
How can I limit the number of rows returned by a query?
Use LIMIT
to restrict the number of rows. Example: LIMIT 10
.
What is a subquery, and how is it used?
A subquery is a query nested inside another query, often used for complex filtering.
How do I insert multiple rows at once in SQL?
Use a single INSERT
statement with multiple VALUES
clauses. Example: INSERT INTO table (col1, col2) VALUES (1, 'a'), (2, 'b');
What happens if I run DELETE
without a WHERE
clause?
All rows in the table will be deleted. Always use a WHERE
clause unless you intend to clear the table.
What is the difference between TRUNCATE
and DELETE
?
TRUNCATE
removes all rows without logging individual row deletions, making it faster but less flexible than DELETE
.
How do I join more than two tables?
Use multiple JOIN
clauses. Example: FROM table1 INNER JOIN table2 ON condition1 INNER JOIN table3 ON condition2
.
What are SQL transactions?
Transactions allow multiple SQL operations to be executed as a single unit, ensuring data consistency.
What is the use of DISTINCT
in SQL?
DISTINCT
removes duplicate rows from the result set. Example: SELECT DISTINCT column_name FROM table;
.
How do I handle case sensitivity in SQL queries?
SQL is generally case-insensitive, but behavior can vary by database. Use LOWER
or UPPER
functions for consistency.
Can I combine multiple conditions in a WHERE
clause?
Yes, use AND
, OR
, and parentheses for complex conditions.
How do I prevent SQL injection?
Use parameterized queries or prepared statements to ensure that user input is treated as data, not executable code.