SQL (Structured Query Language) is the backbone of managing and manipulating data in databases. Whether you’re a beginner or an experienced developer, understanding SQL is essential for retrieving, updating, and organizing data efficiently. From simple queries to complex joins, SQL helps power websites, applications, and data-driven systems.
This cheat sheet is designed to provide a quick reference to the most commonly used SQL commands, functions, and best practices. Whether you need to fetch data, modify records, or optimize queries, this guide will help you write SQL efficiently and effectively. Let’s dive in and explore the essential SQL commands every developer should know!
On This Page
Table of Contents
SQL Cheat Sheet Table : Essential Commands and Syntax
Concept | SQL Command / Syntax | Example / Notes |
---|---|---|
Create Database | CREATE DATABASE database_name; | Creates a new database. |
Drop Database | DROP DATABASE database_name; | Deletes a database permanently. |
Select Database | USE database_name; | Switches to a specific database. |
Create Table | CREATE TABLE table_name ( column1 TYPE, column2 TYPE ); | Defines a new table. |
Drop Table | DROP TABLE table_name; | Deletes a table. |
Alter Table (Add Column) | ALTER TABLE table_name ADD column_name TYPE; | Adds a new column to an existing table. |
Alter Table (Modify Column) | ALTER TABLE table_name MODIFY column_name TYPE; | Modifies an existing column. |
Alter Table (Drop Column) | ALTER TABLE table_name DROP COLUMN column_name; | Removes a column from a table. |
Insert Data | INSERT INTO table_name (column1, column2) VALUES (val1, val2); | Adds new data into a table. |
Update Data | UPDATE table_name SET column1 = value WHERE condition; | Modifies existing records. |
Delete Data | DELETE FROM table_name WHERE condition; | Deletes specific records. |
Select Data | SELECT column1, column2 FROM table_name WHERE condition; | Retrieves specific data. |
Select All Data | SELECT * FROM table_name; | Retrieves all records. |
Where Clause | SELECT * FROM table_name WHERE column = value; | Filters data based on a condition. |
Order By Clause | SELECT * FROM table_name ORDER BY column ASC/DESC; | Sorts query results. |
Group By Clause | SELECT column, COUNT(*) FROM table_name GROUP BY column; | Groups data and performs aggregation. |
Having Clause | SELECT column, COUNT(*) FROM table GROUP BY column HAVING COUNT(*) > 1; | Filters grouped results. |
Joins – Inner Join | SELECT * FROM table1 INNER JOIN table2 ON table1.id = table2.id; | Returns matching rows from both tables. |
Joins – Left Join | SELECT * FROM table1 LEFT JOIN table2 ON table1.id = table2.id; | Returns all rows from the left table, plus matching rows from the right table. |
Joins – Right Join | SELECT * FROM table1 RIGHT JOIN table2 ON table1.id = table2.id; | Returns all rows from the right table, plus matching rows from the left table. |
Joins – Full Outer Join | SELECT * FROM table1 FULL OUTER JOIN table2 ON table1.id = table2.id; | Returns all records from both tables. |
Union | SELECT column1 FROM table1 UNION SELECT column1 FROM table2; | Combines results from multiple queries. |
Union All | SELECT column1 FROM table1 UNION ALL SELECT column1 FROM table2; | Includes duplicates in the result set. |
Subquery | SELECT * FROM table WHERE column = (SELECT column FROM table2 WHERE condition); | Executes a query inside another query. |
Exists Clause | SELECT * FROM table1 WHERE EXISTS (SELECT * FROM table2 WHERE condition); | Returns true if the subquery has results. |
Case Statement | SELECT column, CASE WHEN condition THEN result ELSE other_result END FROM table; | Implements conditional logic in SQL queries. |
Limit Rows | SELECT * FROM table_name LIMIT 10; | Retrieves only the first 10 rows. |
Offset Rows | SELECT * FROM table_name LIMIT 10 OFFSET 5; | Skips the first 5 rows and retrieves the next 10. |
Indexing | CREATE INDEX index_name ON table(column); | Improves query performance. |
Drop Index | DROP INDEX index_name; | Removes an index. |
View Creation | CREATE VIEW view_name AS SELECT column FROM table; | Creates a virtual table. |
Drop View | DROP VIEW view_name; | Deletes a view. |
Stored Procedure | CREATE PROCEDURE proc_name() BEGIN SQL commands; END; | Defines a reusable SQL procedure. |
Call Stored Procedure | CALL proc_name(); | Executes a stored procedure. |
Function | CREATE FUNCTION func_name(params) RETURNS TYPE AS BEGIN RETURN expression; END; | Defines a function. |
Call Function | SELECT func_name(params); | Calls a function. |
Triggers | CREATE TRIGGER trigger_name BEFORE/AFTER INSERT ON table FOR EACH ROW BEGIN SQL; END; | Executes SQL automatically on insert, update, or delete. |
Drop Trigger | DROP TRIGGER trigger_name; | Deletes a trigger. |
Transaction Begin | START TRANSACTION; | Begins a transaction. |
Commit Transaction | COMMIT; | Saves changes. |
Rollback Transaction | ROLLBACK; | Undoes changes. |
Grant Permissions | GRANT SELECT, INSERT ON database.* TO 'user'@'host'; | Assigns privileges. |
Revoke Permissions | REVOKE SELECT, INSERT ON database.* FROM 'user'@'host'; | Removes privileges. |
Show Users | SELECT user FROM mysql.user; | Displays all users. |
Show Tables | SHOW TABLES; | Lists all tables in a database. |
Describe Table | DESC table_name; | Shows table schema details. |
Show Indexes | SHOW INDEX FROM table_name; | Displays index details. |
Show Databases | SHOW DATABASES; | Lists all available databases. |
Wrap UP
SQL is the backbone of database management, allowing developers to store, manipulate, and retrieve data efficiently. Whether you are performing simple queries or complex transactions, this cheat sheet serves as a handy reference to accelerate your SQL development.
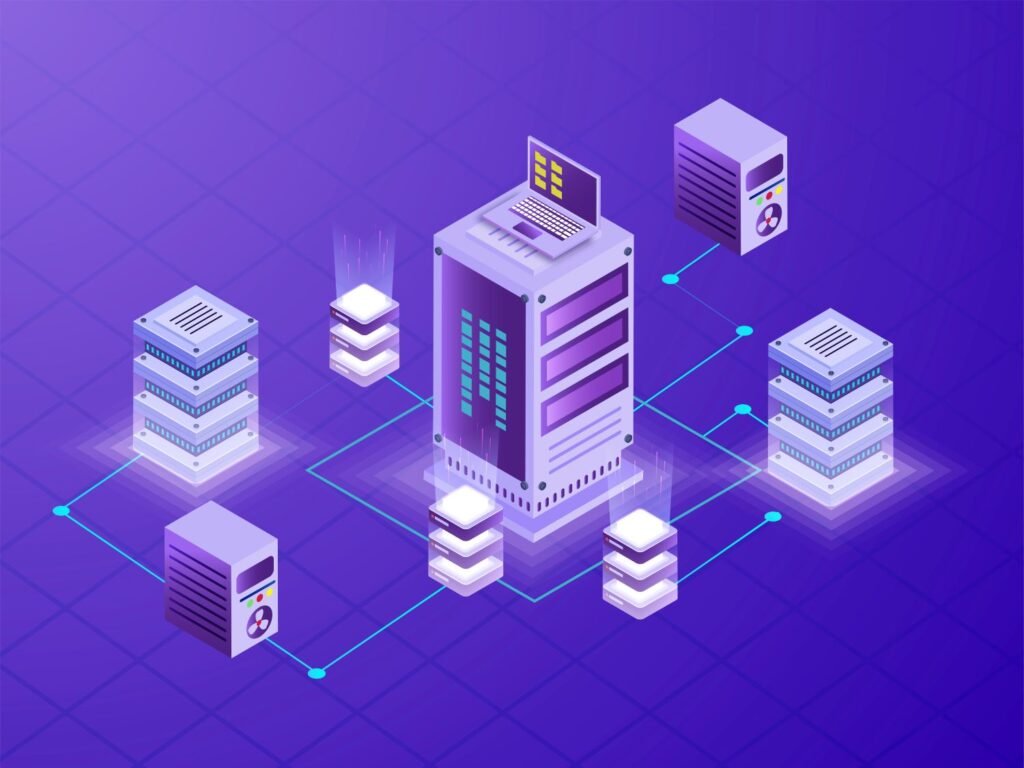
Bookmark this guide for quick access, and keep optimizing your database queries for better performance!
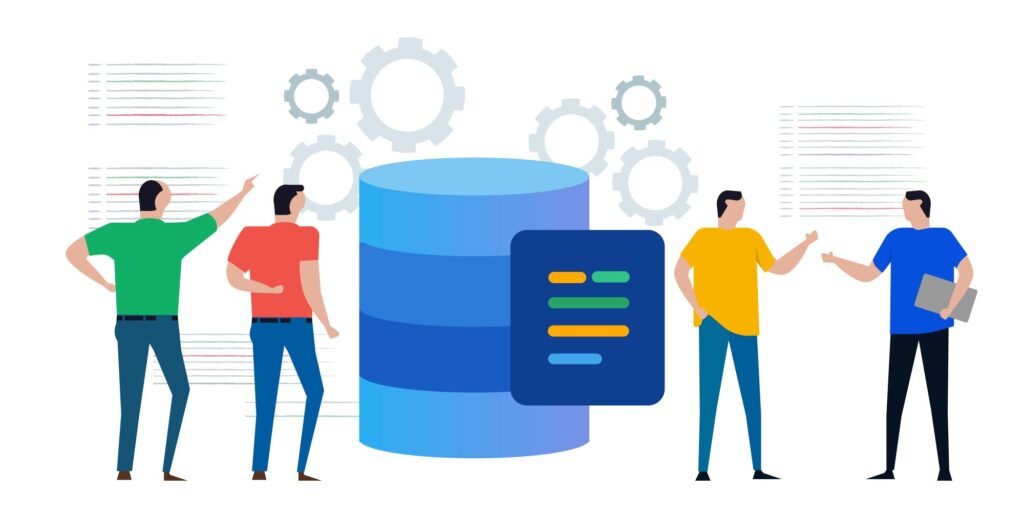
Checkout Other Chearsheets here.
FAQs
What is SQL and why is it important?
SQL (Structured Query Language) is a programming language used to manage and manipulate databases. It allows users to store, retrieve, update, and delete data efficiently. SQL is essential because it helps in organizing large amounts of data and ensures seamless data management for applications.
What are the most commonly used SQL commands?
Some of the most frequently used SQL commands include:SELECT
– Retrieves data from a databaseINSERT
– Adds new recordsUPDATE
– Modifies existing recordsDELETE
– Removes recordsCREATE TABLE
– Creates a new tableDROP TABLE
– Deletes a tableJOIN
– Combines data from multiple tables
What are SQL joins and why are they important?
SQL joins are used to combine records from two or more tables based on a related column. The most common types of joins are:
INNER JOIN – Returns matching records from both tables
LEFT JOIN – Returns all records from the left table and matching records from the right table
RIGHT JOIN – Returns all records from the right table and matching records from the left table
FULL OUTER JOIN – Returns all records when there is a match in either table
Joins are crucial because they allow you to fetch and analyze data from multiple tables without redundancy.
How can I improve SQL query performance?
To optimize SQL queries for better performance, follow these tips:
— Use indexes on frequently searched columns.
— Avoid using SELECT *
; specify only the required columns.
— Use joins efficiently instead of nested subqueries.
— Apply proper WHERE clauses to filter unnecessary data.
— Use LIMIT to restrict large data retrieval.
— Regularly analyze and optimize database structure.
What is indexing in SQL, and how does it help?
Indexing is a way of improving the speed of SQL queries by creating a reference for database records. It allows the database engine to find data faster instead of scanning entire tables. However, excessive indexing can slow down data insertion and updates, so it’s essential to use indexes wisely.
What is the difference between SQL and NoSQL?
SQL databases (like MySQL, PostgreSQL) store data in structured tables and use a predefined schema. NoSQL databases (like MongoDB, Firebase) store data in flexible formats like JSON and are more suited for handling large-scale unstructured data.
What are SQL transactions, and why are they used?
A transaction in SQL is a sequence of operations performed as a single unit. Transactions ensure data integrity using the ACID principles:
Atomicity – All operations succeed or none at all.
Consistency – The database remains in a valid state.
Isolation – Transactions do not interfere with each other.
Durability – Once committed, changes are permanent.
Transactions are used in banking, e-commerce, and applications requiring multiple-step data processing.
What is the difference between DELETE, TRUNCATE, and DROP?
DELETE – Removes specific records while keeping the table structure intact.
TRUNCATE – Removes all records from a table but keeps the structure.
DROP – Deletes the entire table, including its structure.
What are stored procedures, and why are they used?
A stored procedure is a set of SQL commands saved and executed as a function. It helps in:
— Reducing code duplication
— Improving performance
— Enhancing security by restricting direct table accessHt