In today’s web development, APIs are essential for letting different software systems communicate with each other. Two popular ways to design APIs are GraphQL and REST. Both methods help with sharing data, but they have different approaches and ways of doing things.
On This Page
Table of Contents
What is REST API?
REST (Representational State Transfer) API is a web service communication standard that allows different software systems to communicate over the internet. 🌐 It’s built on HTTP, making it easy to understand and implement. In simple terms, REST APIs enable the sharing of data and functionalities between different systems effortlessly.

Key Principles of REST
REST adheres to several key principles that make it an efficient method for web service architectures:
- Stateless Operations: Each request from a client to a server must contain all the information the server needs to fulfill that request. The server does not store the state of the client between requests.
- Client-Server Architecture: The client and server operate independently, allowing each to evolve separately.
- Cacheable Responses: Responses from the server can be cached by the client to improve performance.
- Uniform Interface: REST APIs follow a uniform set of rules and conventions, making them easy to use and understand.
RESTful Methods
REST APIs primarily use four HTTP methods:
Method | Description |
---|---|
GET | Retrieve data from the server |
POST | Create new data on the server |
PUT | Update existing data on the server |
DELETE | Delete data from the server |
Use Cases and Examples
REST APIs are used in a myriad of real-life applications. For instance:
- Social Media Integration: Allowing apps to fetch and post data on social media platforms like Twitter and Facebook.
- eCommerce Systems: Facilitating communication between the frontend and backend systems of an online store.
- Weather Services: Enabling apps to access weather data from global weather services.
Here’s an example:
fetch('https://api.example.com/data', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
}).then(response => response.json())
.then(data => console.log(data));
In summary, REST APIs are an indispensable tool for modern web services, providing efficient and flexible ways to connect different systems.
What is GraphQL?
GraphQL is a query language for your API, and a server-side runtime for executing queries by using a type system you define for your data. It was developed by Facebook in 2012 and released as open-source in 2015. Unlike REST APIs, where multiple endpoints are needed, GraphQL allows you to fetch all the necessary data in a single request.
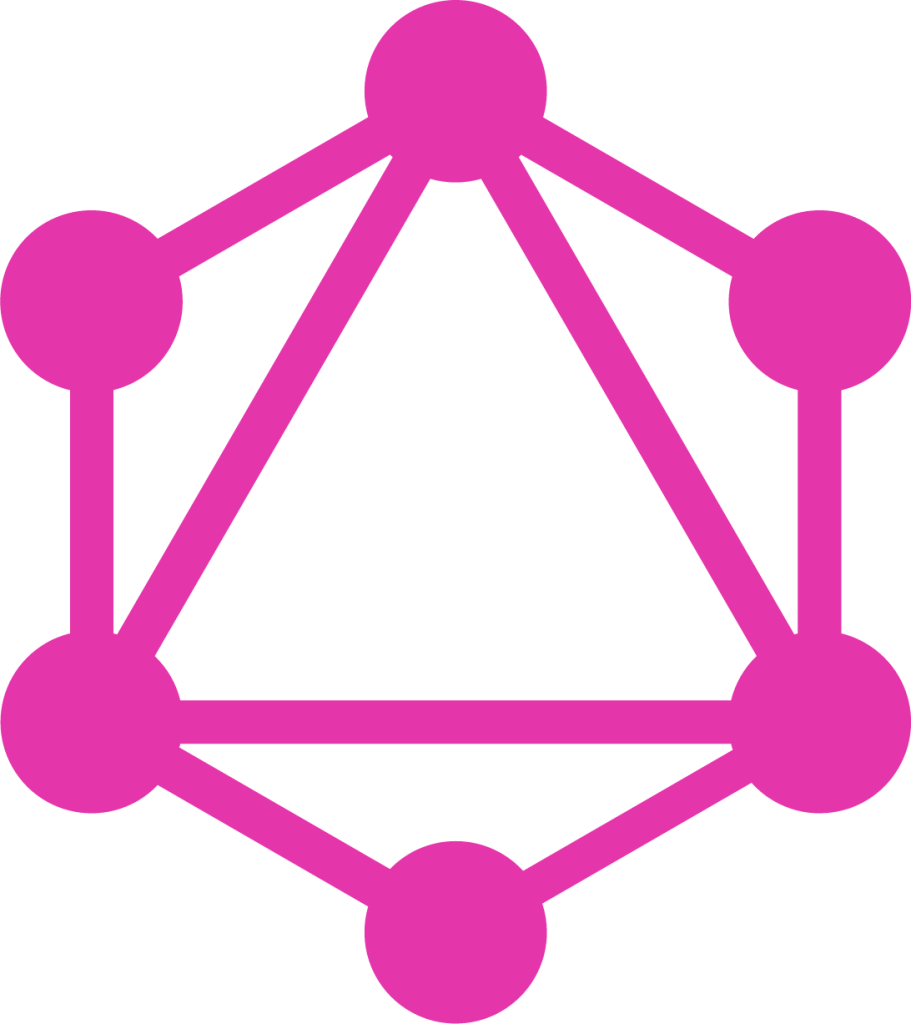
Core Concepts of GraphQL
Schema: The skeleton of your API. It defines types and relationships between them.
Types: Represents what kind of data you can query from the API.
Queries: Requests to read or fetch data.
Mutations: Requests to modify or write data.
Resolvers: Functions that handle the logic of fetching the requested data.
Query and Mutation Operations
In GraphQL, you perform operations with queries and mutations:
Query Example:
{
user(id: "1") {
name
age
}
}
This query requests the name and age of a user with the ID of 1.
Mutation Example:
mutation {
addUser(name: "Jane", age: 30) {
id
name
}
}
This mutation adds a new user named Jane, aged 30, and returns the new user’s ID and name.
Schema and Type System
The schema is the most crucial part of a GraphQL API and it uses the following type system:
- Scalars: Basic data types such as
Int
,Float
,String
, etc. - Object Types: Represents composite data types.
- Input Types: Used in mutations to pass data.
Use Cases and Examples
GraphQL is versatile and can be used in various scenarios, such as:
- Mobile Apps: Efficient data fetching, reducing over-fetching and under-fetching.
- Web Development: Flexibility to query exactly what you need.
- Microservices: Efficiently stitch multiple services into a single API.
In a nutshell, GraphQL is a powerful tool for modern APIs, empowering developers to build more efficient, flexible, and team-friendly APIs.
Key Differences Between GraphQL and REST
In the world of APIs, two dominant paradigms exist: GraphQL and REST. Both have their place, but understanding their key differences is crucial for developers choosing which to implement.
One significant difference between GraphQL and REST is how they handle data fetching.
Data Fetching and Over-fetching
Here’s a table focused on how REST and GraphQL handle data fetching and over-fetching:
Aspect | REST API | GraphQL |
---|---|---|
Data Fetching | Fixed Endpoints: Each endpoint returns a fixed set of data. | Flexible Queries: Clients specify exactly what data they need. |
Over-fetching | Possible: Clients may receive more data than needed because endpoints return a predefined structure. | Minimized: Clients request only the specific fields required. |
Example Scenario | Fetching User Info: To get user info and posts, you might need to make separate requests to /users/{id} and /posts?userId={id} . | Fetching User Info: A single query can fetch user info and posts in one go: graphql query { user(id: "1") { name posts { title } } } |
Code Example (REST) | Fetching User and Posts:GET /users/1 | GraphQL Query:graphql query { user(id: "1") { name posts { title } } } |
Pros | Simplicity: Easy to implement with predefined endpoints. | Efficiency: Reduces the number of requests and minimizes data over-fetching. |
Cons | Over-fetching/Under-fetching: May need multiple requests or get unnecessary data. | Complexity: Queries can become complex, requiring more careful design. |
Flexibility and Efficiency
Here’s a simplified table focusing on the flexibility and efficiency of REST vs. GraphQL:
Aspect | REST API | GraphQL |
---|---|---|
Endpoints | Fixed Endpoints: Each endpoint returns specific data. | Flexible Queries: Clients request exactly what they need. |
Request Efficiency | Less Efficient: May need multiple requests for different data. | More Efficient: Single request can get all needed data. |
Example Scenario | REST: To get user info and their posts, you might need to call /users/{id} and /posts?userId={id} separately. | GraphQL: One query can fetch user info and their posts together. |
Code Example (REST) | Fetching User Info:GET /users/1 | GraphQL Query:query { user(id: "1") { name posts { title } } } |
Pros | Simple: Easy to use with clear, separate endpoints. | Customizable: Get only the data you ask for in one request. |
Cons | Data Over-fetching: May get more data than needed. | Complex Queries: Can be more complex to set up and manage. |
Versioning
Here’s a simplified table comparing REST and GraphQL approaches to versioning:
Aspect | REST API | GraphQL |
---|---|---|
Versioning Approach | URL Versioning: Different versions in URL paths. | No Versioning: Use a single endpoint. |
Example | REST: /api/v1/users or /api/v2/users | GraphQL: /graphql (always the latest) |
Pros | Clear Versions: Different versions can be maintained separately. | Simplicity: No need to manage multiple versions. |
Cons | Maintenance: Requires managing and updating multiple versions. | Backward Compatibility: Changes may affect all clients using the same endpoint. |
Error Handling and Responses
Here’s a simple table comparing REST API and GraphQL error handling:
Aspect | REST API | GraphQL |
---|---|---|
Error Handling | HTTP Status Codes: Uses standard status codes (e.g., 404, 500). | Error Fields: Errors are included in the response body. |
Example Error | REST: 404 Not Found, 500 Internal Server Error | GraphQL: { "errors": [{ "message": "User not found" }] } |
Error Location | HTTP Headers: Errors are indicated by status codes in headers. | Response Body: Errors are part of the JSON response. |
Pros | Standardized: Easy to understand with common HTTP status codes. | Detailed: Can provide detailed error messages within the response. |
Cons | Limited Detail: Status codes alone may not give enough context. | Complexity: Errors are mixed with regular response data, which can be harder to manage. |
Performance Considerations
Here’s a straightforward table comparing REST and GraphQL performance considerations:
Aspect | REST API | GraphQL |
---|---|---|
Number of Requests | Multiple Requests: Often needs several calls for different data. | Single Request: Can fetch all needed data in one call. |
Data Fetching | Over-fetching: May receive more data than needed. | Precise Fetching: Only fetches the data requested. |
Server Load | Higher Load: More requests can increase server load. | Lower Load: Fewer requests can reduce server load. |
Complex Queries | Simple Queries: Easier to handle but can result in multiple requests. | Complex Queries: Can be more resource-intensive due to complex queries. |
Example Scenario | REST: Fetching user details and their posts may need separate calls. | GraphQL: One query can get user details and posts together. |
When to Use REST vs. GraphQL
In the world of APIs, REST and GraphQL are two dominant paradigms. Let’s explore when to use each by considering various factors, including project requirements, scalability, maintenance, and team expertise.
Project Requirements
Choosing between REST and GraphQL often starts with understanding project requirements:
- REST: Suitable for simpler applications with well-defined endpoints.
- GraphQL: Ideal for complex applications where clients demand specific data.
For example, an e-commerce site that needs specific product information might benefit from GraphQL’s flexibility.
Scalability and Maintenance
Scalability and maintainability are crucial for long-term success:
- REST: Easier to cache and scale at the network level.
- GraphQL: Efficient data fetching but requires careful query management to prevent server overload.
An application with high traffic might leverage REST’s scalability advantages. Conversely, if the app is data-driven with frequent changes in data requirements, GraphQL offers flexibility.
Team Expertise and Resources
The team’s expertise and resource availability are pivotal:
- REST: Generally well-understood with extensive documentation and tooling.
- GraphQL: Requires in-depth knowledge of the query language and server setup.
Teams familiar with REST can quickly adopt it to speed up the development process, while a team with diverse tech skills might harness GraphQL for its precise data-fetching capabilities.
The appropriate choice largely depends on project specifics, scalability needs, and team capabilities. Evaluate these factors carefully to select the most suitable API approach for your project.
Tools and Libraries
APIs are the backbone of modern web development, facilitating seamless data exchange between applications. REST (Representational State Transfer) and GraphQL are two popular API styles. Let’s explore the tools and libraries that support each, emphasizing their practical usage.
Popular REST Tools and Libraries
REST APIs are known for their simplicity and scalability. Some popular tools and libraries that support REST include:
- Express.js: A minimal and flexible Node.js web application framework, helping in building robust RESTful APIs.
- Retrofit: A type-safe HTTP client for Android and Java.
- Flask: A lightweight WSGI web application framework for Python, ideal for quick development of RESTful APIs.
Example of a basic REST API in Express.js:
const express = require('express');
const app = express();
app.get('/api/data', (req, res) => {
res.json({
message: 'Hello, World!'
});
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
GraphQL Tools and Libraries
GraphQL APIs offer more flexibility by allowing clients to specify the shape and amount of data they need. Key tools and libraries for GraphQL development include:
- Apollo Server: A community-driven, easy-to-use GraphQL server for JavaScript.
- GraphQL.js: The JavaScript reference implementation of GraphQL.
- Prisma: A next-generation ORM that allows you to access your database through a type-safe GraphQL API.
Example of a basic GraphQL schema in Apollo Server:
const { ApolloServer, gql } = require('apollo-server');
const typeDefs = gql`
type Query {
hello: String
}
`;
const resolvers = {
Query: {
hello: () => 'Hello, World!'
}
};
const server = new ApolloServer({
typeDefs,
resolvers
});
server.listen().then(({ url }) => {
console.log(`🚀 Server ready at ${url}`);
});
Choosing between REST and GraphQL
When choosing between REST and GraphQL, consider the following factors:
- Data Fetching: GraphQL allows fetching specific data in a single request, whereas REST may require multiple endpoints.
- Development and Maintenance: REST is straightforward and easier to implement initially. GraphQL requires a deeper understanding but offers more flexibility long-term.
- Performance: REST might be more performant for simple requests, but GraphQL shines in complex queries and efficient data retrieval.
Example: Facebook initially developed GraphQL to optimize their mobile development. On the other hand, companies like Twitter still rely on REST APIs for simplicity and wide adoption.
WrapUP
Both REST and GraphQL have their merits and best-use scenarios. While REST excels in simplicity and ease of implementation, GraphQL offers flexibility and efficiency. Choose based on your project’s specific needs and scalability requirements.
FAQs
What is the main difference between GraphQL and REST APIs?
GraphQL is a query language for APIs that allows clients to request only the specific data they need, using a single endpoint. REST APIs use a set of predefined endpoints to handle different operations, and clients typically receive more data than required.
How does data fetching differ between GraphQL and REST?
GraphQL allows clients to request exactly the data they need in a single query, reducing over-fetching and under-fetching. REST may require multiple requests to different endpoints to retrieve related data, which can lead to over-fetching or under-fetching issues.
Is GraphQL more complex to implement than REST?
GraphQL can be more complex to implement due to its schema definition, query language, and resolver functions. However, it provides greater flexibility and efficiency. REST is generally simpler but may require more endpoints and handling of various data retrieval scenarios.
What are some common use cases for GraphQL?
GraphQL is often used in scenarios requiring complex data relationships, dynamic querying, and efficient data retrieval, such as in modern web and mobile applications with evolving data needs.
What are some common use cases for REST APIs?
REST is commonly used for traditional web services, CRUD operations, and applications with well-defined endpoints and data structures. It is suitable for simpler use cases where the flexibility of GraphQL may not be necessary.