In today’s interconnected digital landscape, web applications often need to interact with resources hosted on different domains. While this inter-domain interaction is essential for creating rich, interactive experiences, it also introduces significant security risks. Cross-Origin Resource Sharing (CORS) is a critical mechanism that allows web applications to securely request resources from other domains.
On This Page
Table of Contents
What is CORS?
Cross-Origin Resource Sharing (CORS) is a security feature implemented by web browsers to control how resources are requested from different origins. It helps prevent malicious actions by restricting web pages from making requests to a domain different from the one that served the web page. This is essential for protecting sensitive data and maintaining the integrity of web applications.
When a web page makes a request to a server at a different origin (domain, protocol, or port) than its own, it is considered a cross-origin request. Without CORS, these requests would be blocked by the browser’s same-origin policy, which is designed to prevent malicious sites from reading sensitive data from another site. However, CORS allows servers to specify who can access their resources, thereby enabling a controlled and secure sharing of data across different domains. 🔒
CORS in web development
Aspect | Explanation |
---|---|
Purpose | Manage cross-origin requests and enhance web security |
Function | Allows servers to specify permitted domains for resource access |
Importance | Prevents unauthorized access and potential security vulnerabilities |
Common Use Cases | API integrations, third-party services, and secure data sharing |
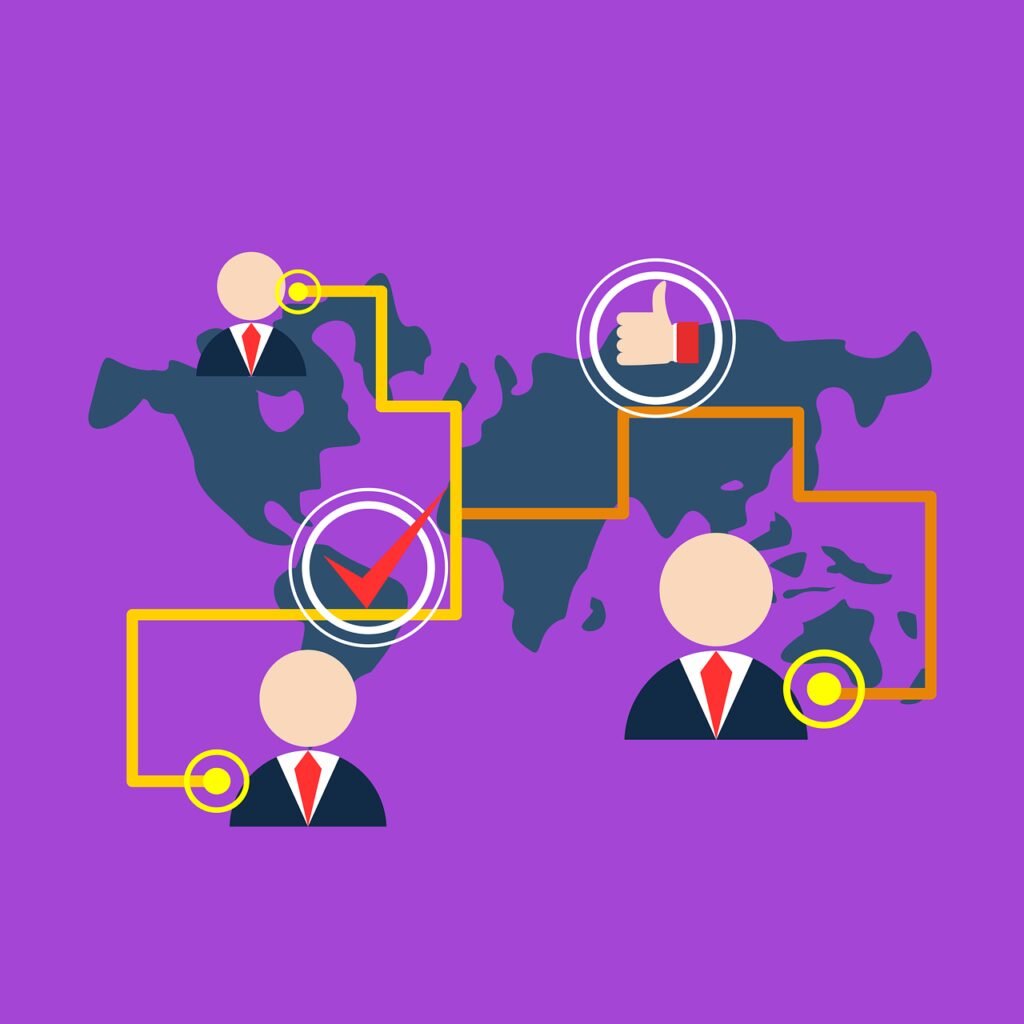
Imagine you have a group of friends who are all hanging out at your house. You only let in people you know and trust. This is similar to how websites operate under the Same-Origin Policy. They only allow interactions with content from their own domain, or ‘house,’ to ensure security. However, what happens if you need to let in a friend from another town?This is where Cross-Origin Resource Sharing (CORS) comes into play. It’s like giving special permission to a trusted friend from another town to join your party.
Key Features of CORS
Here are some key features of CORS:
- Origin Header: Indicates the origin of the request.
- Access-Control-Allow-Origin: Specifies which origins are permitted to access the resource.
- Access-Control-Allow-Methods: Lists the HTTP methods that are allowed when accessing the resource.
- Access-Control-Allow-Headers: Identifies the headers that can be used in the actual request.
CORS vs. Same-Origin Policy
The Same-Origin Policy is like a bouncer at a club. Its job is to make sure that only people from the same club can interact with each other. This means that a script on a webpage can only access data from the same domain it was loaded from. This policy helps maintain security by preventing malicious scripts from accessing sensitive information from different sources. It’s the first line of defense, ensuring that only ‘known’ entities can interact with each other.
Here is a comparison table highlighting the differences between CORS and the Same-Origin Policy:
Feature | CORS | Same-Origin Policy |
---|---|---|
Purpose | Allows controlled cross-origin requests | Blocks cross-origin requests |
Flexibility | More flexible with proper configuration | Strict, limited to the same origin |
Usage | Used for modern web applications | Default browser behavior |
CORS is a powerful feature for enhancing web security and enabling seamless integration of resources across different domains. Understanding its functionality is crucial for developers aiming to build secure and robust web applications. 🌐🔐
How CORS Works
CORS works by using HTTP headers to determine whether a cross-origin request is safe. When a web page makes a request to a different domain, the browser first sends a preflight request to the server. This preflight request checks if the server permits the actual request. If the server allows it, the browser proceeds with the original request. If not, the browser blocks the request, ensuring security.

Imagine you’re hosting a dinner party, and you have a guest list. Cross-Origin Resource Sharing (CORS) is like that guest list for web servers. It determines who is allowed to access resources and who is not. In the digital world, CORS helps manage which web pages can interact with your server’s data.
Another example could be let’s say you want to invite your friend from another town to your party. You need to send them an invitation and get a confirmation. Similarly, when a web page wants to request resources from a different domain, it sends a CORS request. The other domain can then respond with permissions, much like accepting your invitation. If the other domain agrees, it sends back the needed data, allowing the interaction to occur.
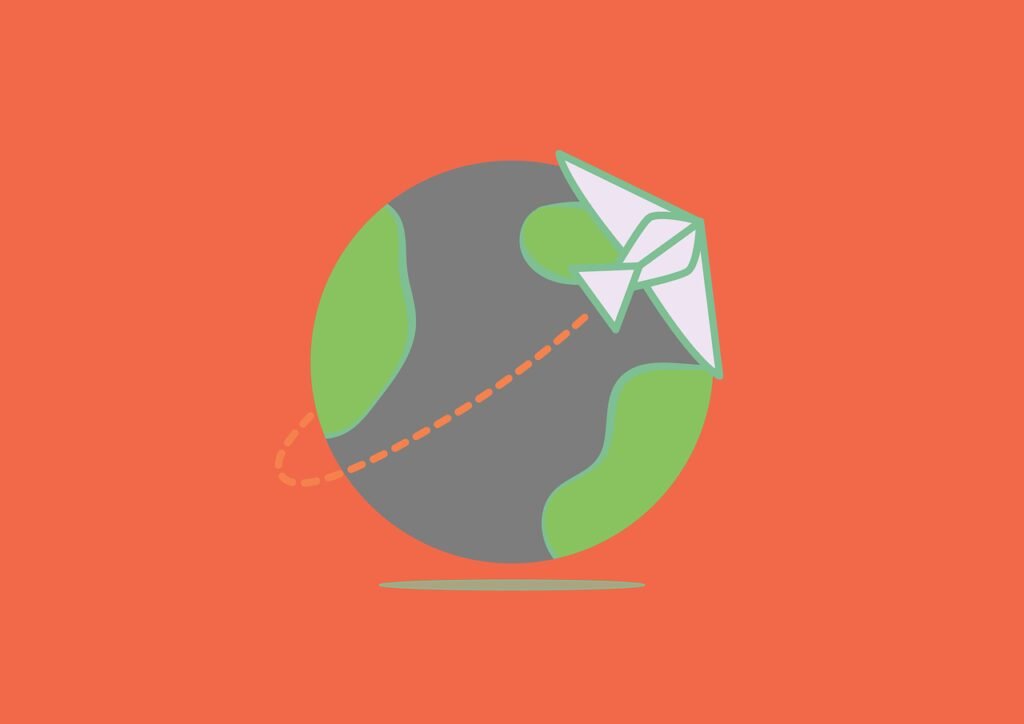
HTTP Headers: The Digital Invitation
Just as you would send invitations to your dinner party, web servers use HTTP headers to communicate permissions. For CORS, these headers include Access-Control-Allow-Origin
, Access-Control-Allow-Methods
, and Access-Control-Allow-Headers
. These headers dictate who can come to the party (which origins are allowed), what they can bring (which methods are allowed), and any special instructions they need to follow.
Simple Requests vs. Preflight Requests
Think of simple requests as guests who come to your party without needing any special permission. They bring a dish, chat, and everything is smooth. These requests use methods like GET, POST, and HEAD and don’t require special headers.
However, some guests might have dietary restrictions or need special accommodations. These are the preflight requests. Before they can join the main event, they send an OPTIONS request to check if they can proceed. This is like calling ahead to see if they can bring their special dish. If the server (host) approves, the main request follows.
Here’s a simple example to illustrate CORS headers:
fetch('https://example.com/data', {
method: 'GET',
headers: {
'Content-Type': 'application/json'
}
}).then(response => response.json()).then(data => console.log(data));
In this example, the browser makes a simple GET request to fetch data. If the server allows it, the data is received and logged. If not, the browser blocks the request, just like a bouncer at a party.
Why CORS Matters

In today’s interconnected world, websites often need to interact with resources from different domains, like fetching data from external APIs or loading images from a content delivery network. CORS acts as a bridge, enabling these interactions while maintaining security. Without CORS, our web experiences would be limited, much like a party confined to just your house without any external friends.
Security implications of CORS
Cross-Origin Resource Sharing (CORS) is a security feature implemented by browsers to manage requests for resources from different origins. Imagine you are at a friend’s house and you want to borrow a book from their shelf. You need their permission to take it, similar to how a web application needs permission to access resources from another domain.
Potential Risks and Vulnerabilities
While CORS is essential for web security, improper configuration can lead to significant vulnerabilities. Here’s a table to illustrate the common risks:
Risk | Description |
---|---|
Data Theft | Attackers can steal sensitive information by exploiting misconfigured CORS policies. |
Malicious Scripts | Hackers can inject malicious scripts into webpages, leading to unauthorized actions. |
Unauthorized Requests | Illegitimate requests can be made to a server, resulting in data breaches or other security issues. |
Common Attacks Exploiting CORS
Several attacks take advantage of CORS vulnerabilities. Here are a few:
Attack | Explanation |
---|---|
Cross-Site Scripting (XSS) | Attackers inject malicious scripts that can compromise user sessions and data. |
Cross-Site Request Forgery (CSRF) | Unauthorized commands are transmitted from a user trusted by the website. |
Man-in-the-Middle (MitM) | Attackers intercept and alter communications between users and servers. |
Importance of Correct Configuration
Proper configuration of CORS is crucial to avoid security pitfalls. Here’s a simple coding example to illustrate the correct setup:
const express = require('express');
const cors = require('cors');
const app = express();
const corsOptions = {
origin: 'https://trusteddomain.com',
methods: 'GET,HEAD,PUT,PATCH,POST,DELETE',
credentials: true,
optionsSuccessStatus: 204
};
app.use(cors(corsOptions));
app.get('/data', (req, res) => {
res.json({ message: 'This is secure data' });
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
In this example, only requests from ‘https://trusteddomain.com’ are allowed, minimizing the risk of unauthorized access.
Just like locking your doors at night, ensuring that your CORS policies are in place protects your data and your users from potential threats.
Setting Up CORS in Apache/Nginx Web Servers
Apache
To enable CORS in Apache, modify the httpd.conf
file:
Header set Access-Control-Allow-Origin "*"
Remember to restart the server after making these changes.
Nginx
For Nginx, add the following to your server block:
add_header 'Access-Control-Allow-Origin' '*';
Restart Nginx to apply changes.
Configuring CORS in Backend Frameworks
Node.js
To implement CORS in a Node.js application, use the cors
middleware:
const express = require('express');
const cors = require('cors');
const app = express();
app.use(cors());
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Django
Use django-cors-headers
to enable CORS in Django:
pip install django-cors-headers
Add it to INSTALLED_APPS
and MIDDLEWARE
in your settings.py
:
INSTALLED_APPS = [
...,
'corsheaders',
]
MIDDLEWARE = [
...,
'corsheaders.middleware.CorsMiddleware',
]
Specify allowed origins:
CORS_ALLOWED_ORIGINS = [
'http://example.com',
]
Flask
Configuring CORS in Flask
1. Install Flask-CORS: Flask-CORS is an extension for Flask that simplifies the process of configuring CORS in your application. Install it using pip:
pip install flask-cors
2. Import and Initialize Flask-CORS: In your Flask application, import the CORS
class from flask_cors
and initialize it with your Flask app instance. This can be done in the main application file (e.g., app.py
).
from flask import Flask
from flask_cors import CORS
app = Flask(__name__)
CORS(app)
By default, this configuration allows CORS for all domains and all routes.
3. Fine-Tune CORS Configuration: For a more granular control, you can specify the origins, methods, and headers that are allowed. This is useful for enhancing security by limiting access to specific domains and methods.
CORS(app, resources={r"/api/*": {"origins": "http://example.com"}})
In this example, only requests from http://example.com
to routes starting with /api/
are allowed.
Custom Configuration for Specific Endpoints: If you need different CORS settings for specific endpoints, you can apply CORS configuration at the blueprint or route level:
from flask_cors import cross_origin
@app.route('/another_api')
@cross_origin(origins='http://another-example.com')
def another_api():
return 'This route is CORS-enabled for another-example.com'
Best Practices for secure CORS Implementations
Cross-Origin Resource Sharing (CORS) is an essential aspect of web security, allowing web applications to interact with resources from different origins securely. However, implementing CORS incorrectly can expose your application to security vulnerabilities.

1. Whitelisting Trusted Domains
One of the fundamental practices in CORS implementation is whitelisting only trusted domains. This ensures that only specified domains can access your resources. Here’s an example of how to whitelist domains in a Node.js environment:
const cors = require('cors');
const allowedDomains = ['https://trusted.com', 'https://another-trusted.com'];
const corsOptions = {
origin: function (origin, callback) {
if (allowedDomains.indexOf(origin) !== -1) {
callback(null, true);
} else {
callback(new Error('Not allowed by CORS'));
}
}
};
app.use(cors(corsOptions));
2. Restricting Methods and Headers
Restricting HTTP methods and headers is another essential practice for securing CORS. Allow only the necessary methods and headers to interact with your resources:
const corsOptions = {
origin: allowedDomains,
methods: ['GET', 'POST'],
allowedHeaders: ['Content-Type', 'Authorization']
};
app.use(cors(corsOptions));
3. Validating Preflight Requests
Preflight requests are used by browsers to determine whether the actual request is safe to send. Validating these requests ensures that only legitimate requests get processed:
const corsOptions = {
origin: allowedDomains,
methods: ['GET', 'POST'],
preflightContinue: false,
optionsSuccessStatus: 204
};
app.use(cors(corsOptions));
4. Monitoring and Logging CORS Activity
Monitoring and logging CORS activity can help you detect and respond to potential security threats. Consider using middleware to log CORS requests:
app.use((req, res, next) => {
console.log(`CORS request from: ${req.headers.origin}`);
next();
});
Identifying and Resolving CORS Errors

Cross-Origin Resource Sharing (CORS) errors are a frequent challenge for developers working with APIs and web applications. These errors arise when a web application attempts to make requests to a different domain than the one that served the web page. Below, we discuss some of the most common CORS errors and how to identify and resolve them.
Common CORS Errors
The two most frequently encountered errors are:
- ‘No ‘Access-Control-Allow-Origin’ header’
- ‘CORS policy: No ‘Access-Control-Allow-Credentials’ header’
Identifying CORS Errors
When an error occurs, the browser will typically log a message in the console. For example:
Access to XMLHttpRequest at 'https://example.com/api' from origin 'http://yourdomain.com' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header is present on the requested resource.
This message indicates that the server did not include the necessary CORS headers in its response. Let’s look at how to resolve these issues.
Resolving CORS Errors
To resolve these errors, developers must ensure that the server includes the appropriate CORS headers in its response. Here are the usual causes and steps to resolve them:
- Missing ‘Access-Control-Allow-Origin’ header: Ensure the server response includes this header. For example: Access-Control-Allow-Origin: *
- Missing ‘Access-Control-Allow-Credentials’ header: If credentials are necessary, include: Access-Control-Allow-Credentials: true
Key HTTP Headers Used in CORS
The following table provides an overview of the essential HTTP headers used in CORS and their purposes:
Header | Purpose |
---|---|
Access-Control-Allow-Origin | Specifies which origins are permitted to access the resource |
Access-Control-Allow-Credentials | Indicates whether credentials are allowed in the request |
Access-Control-Allow-Methods | Lists the HTTP methods that are permitted when accessing the resource |
Access-Control-Allow-Headers | Specifies which headers can be used during the actual request |
🔍 Tip: Use browser developer tools to inspect and debug CORS headers. This can help quickly identify missing or misconfigured headers.
Role of CORS in APIs and Microservices
APIs and microservices often operate across different domains. CORS ensures that these interactions are secure and controlled. Without proper CORS configurations, APIs could become vulnerable to attacks such as cross-site scripting (XSS) and cross-site request forgery (CSRF).
API Type | Common Use Cases | CORS Configuration |
---|---|---|
RESTful APIs | CRUD operations | Access-Control-Allow-Origin |
GraphQL APIs | Querying multiple resources | Preflight requests |
Microservices | Service-to-service communication | Access-Control-Allow-Credentials |
CORS in Single-Page Applications (SPAs)
Single-Page Applications (SPAs) often make asynchronous requests to APIs hosted on different domains. Proper CORS settings are essential to ensure these requests are secure and do not expose the application to vulnerabilities.
- Improves security by defining who can access your resources.
- Prevents unauthorized access and data leakage.
- Facilitates seamless integration with third-party services.
Cross-Origin Resource Sharing is a vital component in the security framework of modern web applications. The principles and practices outlined in this article provide a foundation for securing web applications against CORS-related vulnerabilities. As web technologies evolve, staying informed about best practices and emerging trends in CORS will be essential for maintaining robust security and functionality in your web applications.
FAQs
Why is CORS important for web security?
CORS helps prevent malicious websites from accessing sensitive data from other domains without permission. It enforces a layer of security by controlling which domains can interact with your web application’s resources, thereby mitigating the risk of cross-origin attacks such as Cross-Site Request Forgery (CSRF).
How does the same-origin policy relate to CORS?
The same-origin policy is a security measure that restricts how documents or scripts loaded from one origin can interact with resources from another origin. CORS is an extension to the same-origin policy that allows controlled access to resources on different domains under specified conditions.
What are the key HTTP headers used in CORS?
Key HTTP headers in CORS include:Access-Control-Allow-Origin
: Specifies which origins are allowed to access the resource.Access-Control-Allow-Methods
: Lists the HTTP methods that are allowed when accessing the resource.Access-Control-Allow-Headers
: Indicates which HTTP headers can be used during the request.Access-Control-Allow-Credentials
: Indicates whether the response to the request can be exposed when the credentials flag is true.
What is a preflight request in CORS?
A preflight request is an initial request made by the browser using the OPTIONS method to determine if the actual request is safe to send. This happens when a request uses methods other than GET, POST, or HEAD, or includes non-simple headers. The server must respond with the appropriate CORS headers to allow the actual request to proceed.
How can I configure CORS in my web application?
Configuration depends on the web server or framework you are using. For example:
In Apache, you can use the Header
directive in the .htaccess
file.
In Nginx, you can set headers in the server configuration file.
In Node.js, you can use middleware like cors
.
In Django, you can use the django-cors-headers
library.
What are some best practices for implementing CORS securely?
Best practices include:
Whitelisting only trusted domains.
Restricting the allowed HTTP methods and headers.
Validating and limiting preflight requests.
Regularly monitoring and logging CORS activity to detect and respond to potential misuse.
What common issues might I encounter with CORS, and how can I resolve them?
Common issues include:
Missing or incorrect CORS headers.
Misconfigured server settings.
Browser caching of preflight responses causing unexpected behaviors. You can resolve these issues by ensuring correct header configurations, reviewing server and application logs for errors, and using browser developer tools to debug and test CORS requests.
How does CORS impact APIs and microservices?
CORS is crucial for securing APIs and microservices that are accessed by client-side applications from different domains. Proper CORS configuration ensures that only authorized domains can make requests to your API, protecting it from unauthorized access and potential attacks
Are there any alternatives to using CORS for cross-origin requests?
Alternatives include using JSONP for simple GET requests or server-side proxies that handle cross-origin requests on behalf of the client. However, these methods have their own limitations and security considerations, and CORS remains the preferred method for handling cross-origin requests in modern web development.