In the bustling world of data management, organizations are constantly bombarded with an overwhelming amount of information. Imagine a large retail company that collects data from various sources: sales transactions, customer feedback, inventory levels, and marketing campaigns. Each of these data points is stored in separate tables within a relational database. As the company grows, the need for efficient data retrieval and analysis becomes paramount. This is where SQL views come into play.
SQL views act as virtual tables that simplify complex queries, enhance data security, and improve accessibility. They allow users to present data in a way that is tailored to their specific needs without altering the underlying tables. In this article, we will explore the benefits of SQL views and their real-world applications, all while weaving a narrative that illustrates their importance in today’s data-driven landscape.
On This Page
Table of Contents
What is an SQL View?
To understand the significance of SQL views, let’s first define what they are. An SQL view is essentially a stored query that users can treat as a table. It is created by selecting data from one or more tables and can include filtering, sorting, and joining operations.
Key Characteristics of SQL Views:
- Virtual Table: A view does not store data itself but provides a way to access data from underlying tables.
- Dynamic: Any changes made to the underlying tables are immediately reflected in the view.
- Read-Only or Updatable: Some views can be updated if they meet certain criteria; others are read-only.
Differences Between Views and Tables
Feature | SQL View | Table |
---|---|---|
Data Storage | Does not store data | Physically stores data |
Data Retrieval | Derived from one or more tables | Directly holds data |
Updatability | Can be read-only or updatable | Always updatable |
Performance | May improve performance with caching | Performance depends on indexing |
Benefits of Using SQL Views
Simplification of Complex Queries
Consider a scenario where our retail company needs to analyze sales performance across different regions. The sales data is spread across multiple tables: Sales
, Customers
, and Products
. Writing complex queries to retrieve this information can be cumbersome.

Example:
SELECT
c.Region,
SUM(s.Amount) AS TotalSales
FROM
Sales s
JOIN
Customers c ON s.CustomerID = c.CustomerID
JOIN
Products p ON s.ProductID = p.ProductID
GROUP BY
c.Region;
Instead of running this complex query repeatedly, the company can create a view:
CREATE VIEW RegionalSales AS
SELECT
c.Region,
SUM(s.Amount) AS TotalSales
FROM
Sales s
JOIN
Customers c ON s.CustomerID = c.CustomerID
JOIN
Products p ON s.ProductID = p.ProductID
GROUP BY
c.Region;
Now, retrieving regional sales becomes as simple as:
SELECT * FROM RegionalSales;
Enhanced Data Security
SQL views provide an effective mechanism for enhancing data security. By creating views that expose only specific columns or rows, organizations can restrict access to sensitive information while still allowing users to perform necessary analyses.
Example:
Consider a human resources database containing employee information such as salaries and personal details. Instead of granting direct access to the Employees
table, an HR manager might create a view that excludes sensitive columns:
CREATE VIEW PublicEmployeeInfo AS
SELECT
EmployeeID,
FirstName,
LastName,
Department
FROM
Employees;
This way, users can access essential employee information without compromising sensitive data.
Code Reusability
Creating views allows developers to encapsulate complex logic within a single object. This promotes code reusability, as the same view can be used across different applications or reports without rewriting the underlying SQL logic.
Example:
If our retail company frequently needs to analyze sales by product category, they can create a view that aggregates this information:
CREATE VIEW CategorySales AS
SELECT
p.Category,
SUM(s.Amount) AS TotalSales
FROM
Sales s
JOIN
Products p ON s.ProductID = p.ProductID
GROUP BY
p.Category;
Now, any department needing category sales insights can simply query CategorySales
.
Data Abstraction
SQL views provide a layer of data abstraction, allowing users to interact with simplified representations of complex datasets. This is particularly beneficial for non-technical users who may not be familiar with the intricacies of the underlying database schema.
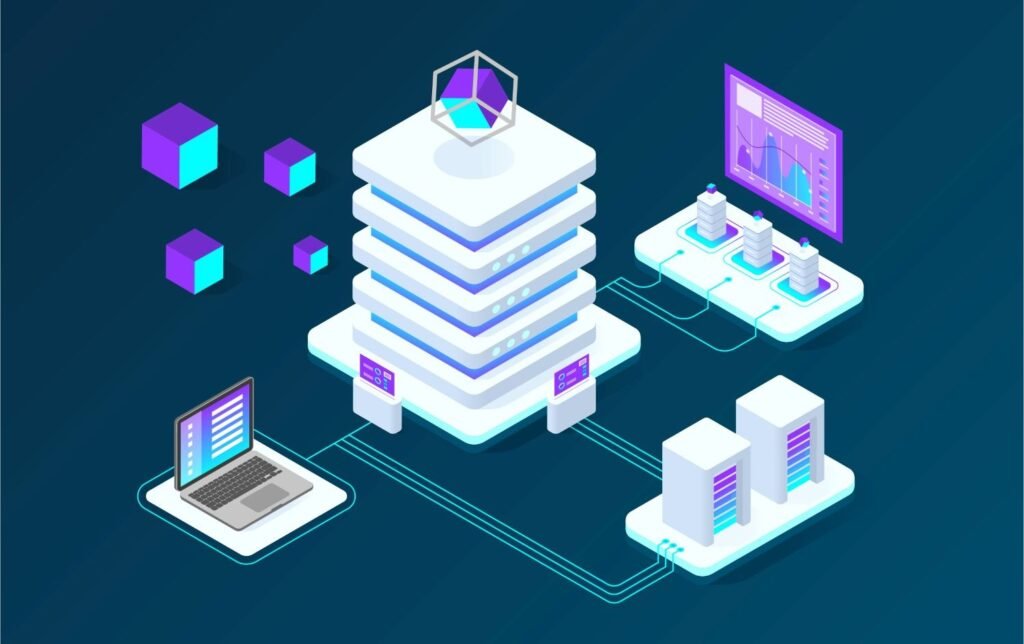
Real-Life Example:
Imagine a marketing team analyzing customer behavior based on purchase history. Instead of exposing them to multiple tables like Customers
, Orders
, and OrderDetails
, an analyst could create a view that consolidates relevant information:
CREATE VIEW CustomerPurchaseHistory AS
SELECT
c.CustomerID,
c.FirstName,
c.LastName,
COUNT(o.OrderID) AS TotalOrders,
SUM(od.Quantity) AS TotalItemsPurchased
FROM
Customers c
LEFT JOIN
Orders o ON c.CustomerID = o.CustomerID
LEFT JOIN
OrderDetails od ON o.OrderID = od.OrderID
GROUP BY
c.CustomerID;
The marketing team can now easily analyze customer purchase behavior without needing to understand the underlying complexity.
Improved Performance with Materialized Views
In scenarios where performance is critical, materialized views can significantly enhance query speed. A materialized view stores the result set of a query physically on disk, allowing for faster retrieval at the cost of additional storage space.
Example:
Suppose our retail company frequently queries total sales by month for reporting purposes. Instead of recalculating this every time, they could create a materialized view:
CREATE MATERIALIZED VIEW MonthlySales AS
SELECT
DATE_TRUNC('month', OrderDate) AS SalesMonth,
SUM(Amount) AS TotalSales
FROM
Sales
GROUP BY
DATE_TRUNC('month', OrderDate);
This materialized view can be refreshed periodically (e.g., daily or weekly), providing quick access to aggregated sales data.
Common Use Cases for SQL Views
Filtering Data for Specific Needs
SQL views are ideal for creating tailored datasets that meet specific requirements without exposing unnecessary information. For instance, if our retail company wants to analyze sales performance only for online orders, they could create a view that filters out in-store transactions:
CREATE VIEW OnlineSales AS
SELECT * FROM Sales WHERE SaleType = 'Online';
Joining Multiple Tables for Comprehensive Insights
As demonstrated earlier, SQL views simplify complex joins across multiple tables. This capability allows analysts to generate comprehensive reports without delving into intricate SQL syntax each time.
Summarized Reporting for Quick Analysis
Views can be designed to provide summarized reports that facilitate quick decision-making. For example, if executives need monthly revenue figures across various product categories, they could rely on a pre-defined view:
CREATE VIEW MonthlyRevenueByCategory AS
SELECT
DATE_TRUNC('month', OrderDate) AS SalesMonth,
p.Category,
SUM(od.Quantity * od.UnitPrice) AS TotalRevenue
FROM
OrderDetails od
JOIN
Products p ON od.ProductID = p.ProductID
JOIN
Orders o ON od.OrderID = o.OrderID
GROUP BY
SalesMonth, p.Category;
Hiding Complexity from End Users
For organizations with non-technical staff who need access to certain datasets, views serve as an excellent way to hide complexity while providing necessary insights. By creating user-friendly views that present only relevant information, businesses empower employees without overwhelming them with technical details.
Ensuring Consistency Across Queries
Using views ensures consistency in how data is accessed across different applications or reports. By centralizing logic within a view, organizations minimize discrepancies that may arise from varying interpretations of complex queries.
Creating and Managing SQL Views
Creating and managing SQL views is straightforward. Here’s how you can do it using basic SQL syntax.
Syntax for Creating Views
To create a view in SQL, use the following syntax:
CREATE VIEW ViewName AS
SELECT Column1, Column2, ...
FROM TableName
WHERE Condition;
Updating and Dropping Views
If changes are needed in an existing view’s definition or if it’s no longer required, you can update or drop it using these commands:
- Updating a View:
CREATE OR REPLACE VIEW ViewName AS
SELECT NewColumn1, NewColumn2,...
FROM NewTableName;
- Dropping a View:
DROP VIEW ViewName;
Best Practices for Using SQL Views
While SQL views offer numerous advantages, adhering to best practices ensures optimal performance and usability.
Considerations for Performance
- Avoid Excessive Complexity: Keep views simple; overly complex views may lead to performance degradation.
- Limit Rows Returned: Use filtering conditions in your views to limit unnecessary data retrieval.
- Use Indexed Tables: Ensure underlying tables have appropriate indexes to enhance performance when accessing views.
When to Use Views vs. Direct Queries
- Use views when you need consistent access patterns across multiple queries.
- Opt for direct queries when performing one-off analyses where performance is less critical.
WrapUP
SQL views are indispensable tools in modern database management systems that empower organizations to navigate complex datasets with ease. By simplifying queries, enhancing security, promoting code reusability, and providing data abstraction, views play a crucial role in optimizing database interactions.
As we’ve explored through real-world examples—from analyzing sales performance at our retail company to ensuring HR confidentiality—understanding how to effectively utilize SQL views will remain vital in today’s ever-evolving data landscape.
In conclusion, embracing SQL views not only streamlines operations but also fosters informed decision-making based on accurate insights—transforming raw data into actionable intelligence for businesses across industries. As organizations continue their journey through digital transformation, mastering these powerful tools will undoubtedly pave the way for success in an increasingly competitive environment.
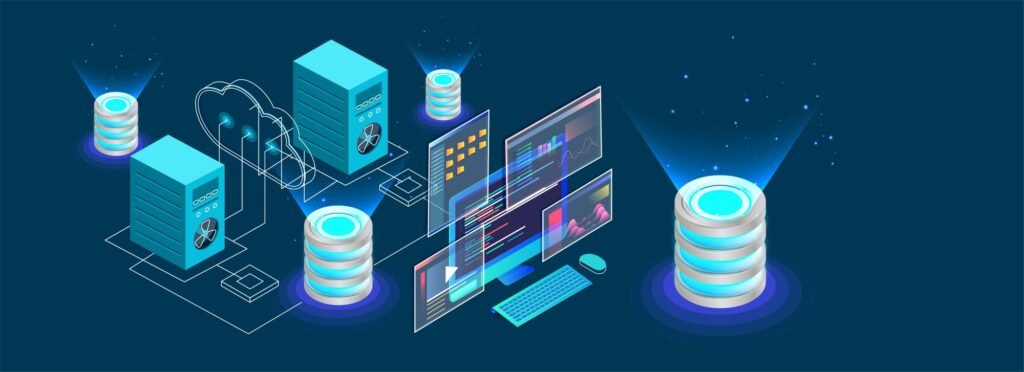
👉 Want to level up your SQL skills? Check out this comprehensive SQL Cheat Sheet for quick commands, syntax, and best practices!
FAQs
What is an SQL view?
An SQL view is like a saved search or a shortcut to a specific set of data from one or more tables. It doesn’t store the data itself but shows you data from the actual tables.
What’s the difference between a view and a table?
A table is where the actual data is stored. A view is just a way to look at that data.
Why use views?
Simpler Queries: Views can make complex queries easier to understand and use.
Security: You can use views to hide sensitive information from certain users[1][2][5]. For example, you might show customer names and addresses but hide their social security numbers.
Customized Data: Views let you present data in a way that makes sense for different users.
Code Reusability: If you often run the same complex query, you can save it as a view and reuse it.
Can we create a view based on another view?
Yes, we can create a view from another view, also known as nested views. However, it’s best to avoid too many layers, as it can make things confusing.
Can we still use a view if the original table is deleted?
No, if we delete the original table, the view will no longer work. It’s like removing the foundation of a house; the house (view) will collapse.
Can we update views?
Yes, views can be updated. However, updating views based on multiple tables can be tricky. Sometimes, we might need special triggers to make sure the underlying tables are updated correctly.
What are materialized/indexed views?
A normal view is like a saved query, but a materialized or indexed view is like saving the query’s results as a table. This can make things faster, especially for complex queries, but it takes up more storage and might not be suitable for frequently updated data.