In the world of web browsing, terms like cookies, sessions, and tokens often come up. Understanding these concepts can significantly enhance our online experience. Let us delve into each of these terms with simple analogies to make them more relatable.
On This Page
Table of Contents
Takeaways: Cookies, Sessions, and Tokens
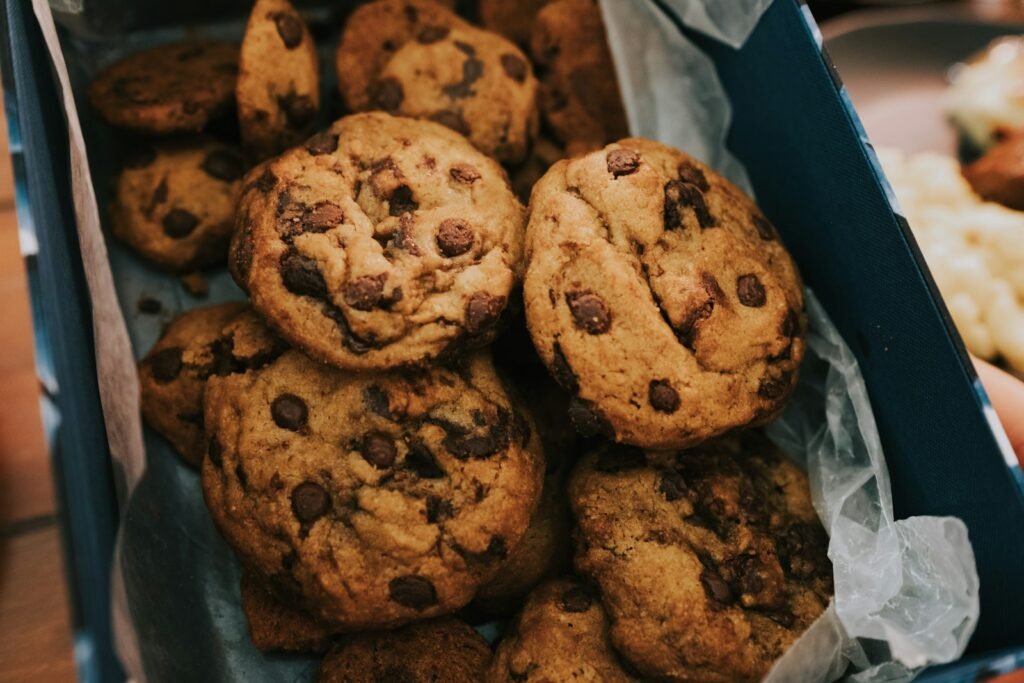
Cookies, in the context of web browsing, can be compared to a note you keep in your pocket. When you visit a website, it may store a small piece of data called a cookie on your device. This cookie might contain details like your login status or site preferences, allowing the website to remember you on your next visit. For instance, if you select a language preference on a website, it will store this choice in a cookie, so you donβt have to select it again.
Sessions, on the other hand, are akin to a visit to a friendβs house. When you enter the house, you start a session, and when you leave, the session ends. Similarly, when you log into a website, a session starts and continues until you log out or become inactive for a certain period. During this session, the website remembers who you are and what you are doing, ensuring a seamless browsing experience.

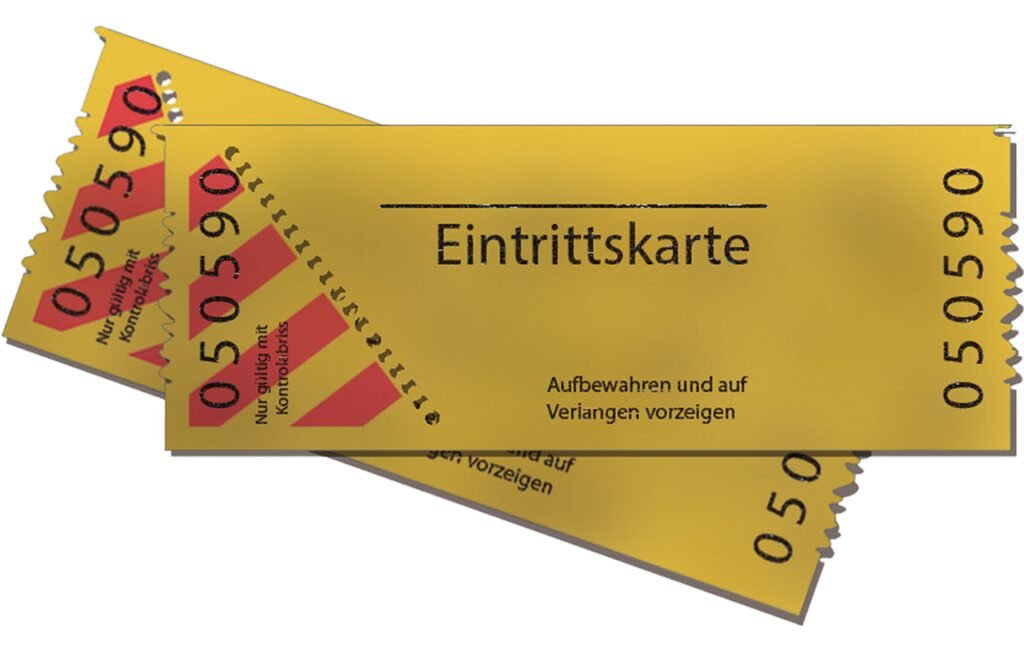
Tokens can be compared to a bus ticket. When you purchase a ticket, it allows you to travel on a bus for a specific duration. Similarly, a token in web browsing is a piece of data that allows you to access certain resources or services for a limited time. Tokens are often used in authentication systems to verify a userβs identity without repeatedly asking for login credentials.
Tokens can be compared to a bus ticket. When you purchase a ticket, it allows you to travel on a bus for a specific duration. Similarly, a token in web browsing is a piece of data that allows you to access certain resources or services for a limited time. Tokens are often used in authentication systems to verify a userβs identity without repeatedly asking for login credentials.
The significance of cookies, sessions, and tokens lies in their ability to enhance user experience and security on the web. They allow websites to remember user preferences, manage user sessions, and authenticate users efficiently. As Tim Berners-Lee, the inventor of the World Wide Web, once said, “Data is a precious thing and will last longer than the systems themselves.” This highlights the importance of managing and understanding how our data is utilized through mechanisms like cookies, sessions, and tokens.
How Cookies, Sessions, and Tokens Work
Cookies πͺ
Cookies are small pieces of data stored by the browser on the user’s device. They help in tracking user information and preferences across different sessions. For instance, when you log into an e-commerce website, a cookie might store your login status so you don’t have to log in again during your next visit.
Imagine you’re at your favorite bakery, and the kind baker remembers your favorite treat every time you return. That’s just like Cookies in web development! When you visit a website, it leaves a small piece of data on your device (in the form of a text file) to remember information about you. This could be anything from your login status πΉ to your preferred language π£οΈ.
Here is a simple example of setting a cookie in JavaScript:
document.cookie = "username=JohnDoe; expires=Fri, 31 Dec 2021 23:59:59 GMT; path=/";
Another example of setting a cookie in Python using the Flask framework:
from flask import Flask, make_response, jsonify
app = Flask(__name__)
@app.route('/set-cookie')
def set_cookie():
resp = make_response(jsonify({'message': 'Cookie set!'}))
resp.set_cookie('mycookie', 'cookie_value') # setting the cookie
return resp
Sessions π§©
Sessions are server-side storage used to track user interactions within a specific timeframe. Unlike cookies, sessions store data on the server, reducing the risk of exposing sensitive information. A practical example is a shopping cart on an online store, where items added to the cart are stored in a session until the purchase is completed.
Another Example could be in the context of our previous example of the Bakery. Imagine you’ve been visiting the bakery daily for a week, and the baker has a notebook where he keeps track of your visits, treats, and preferences. That’s similar to how Sessions work in web development! They provide a way to keep track of user activity over multiple requests.
Here is a basic example of creating a session in PHP:
session_start();
$_SESSION['username'] = 'JohnDoe';
Here’s another simple example of using Sessions with Flask:
from flask import Flask, session, jsonify
app = Flask(__name__)
app.secret_key = 'my_secret_key' # set a secret key for session security
@app.route('/start-session')
def start_session():
session['username'] = 'John_Doe' # setting session variable
return jsonify({'message': 'Session started!'})
@app.route('/get-session')
def get_session():
if 'username' in session:
return jsonify({'username': session['username']}) # retrieve session variable
else:
return jsonify({'message': 'No session found'})
Tokens π
Tokens are used in modern web applications for stateless authentication. They are typically employed in APIs to verify user identity without maintaining a session on the server.
Picture a bouncer at a popular club who checks your ID to verify who you are. Tokens in web development serve a similar purpose, but for digital identities! Instead of storing sensitive information like passwords directly on the server, we create a token that can be used to verify the user without exposing the password.
A common implementation is the JSON Web Token (JWT), which securely transmits information between parties as a JSON object.
Here’s a simple example of using JSON Web Tokens (JWT) with Flask:
from flask import Flask, jsonify, request, abort
from flask_jwt import JWT, jwt_required
from itsdangerous import TimedJSONWebSignatureSerializer as Serializer
app = Flask(__name__)
app.config['SECRET_KEY'] = 'my_secret_key' # set a secret key for JWT
jwt = JWT(app)
@app.route('/login')
def login():
username = request.json.get('username')
password = request.json.get('password')
# perform login logic here
if username and password:
payload = {'id': user_id, 'exp': timestamp + (60 * 60)} # create a payload with user id and expiration time
s = Serializer(app.config['SECRET_KEY'], expires_in=3600) # create a serializer
token = s.dumps(payload) # generate the token
return jsonify({'token': token})
else:
abort(401) # unauthorized
@app.route('/protected')
@jwt_required()
def protected():
return jsonify({'message': 'Access granted!'})
In this example, when a user logs in, they receive a token that can be used to access protected resources for a limited time.
In summary, Cookies, Sessions, and Tokens are essential components of web development that help us create personalized, secure, and user-friendly experiences. They’re like the baker, the notebook, and the bouncer of the digital world, making sure our time online is as pleasant as a warm, freshly baked cookie πͺ!
Pros and Cons of Cookies, Sessions, and Tokens
In the realm of web development and user experience, cookies, sessions, and tokens each come with their own set of benefits and drawbacks. Understanding these pros and cons can help developers make informed choices about which method to implement based on specific needs and requirements.
Pros and Cons of Cookies
Cookies are small pieces of data stored on the user’s browser. They are widely used for managing session state, storing user preferences, and tracking user behavior.
Pros | Cons |
Ease of Use: Simple to implement and manage. π’ | Security Risks: Vulnerable to cross-site scripting (XSS) attacks. π΄ |
Persistence: Can store data for extended periods. π’ | Storage Limitations: Limited to 4KB per cookie. β οΈ |
Accessibility: Can be accessed by both client and server. π’ | Privacy Concerns: Can be used to track user behavior. π΄ |
Pros and Cons of Sessions
Sessions store data on the server side and maintain state across multiple requests from the same user.
Pros | Cons |
Security: Less vulnerable to client-side attacks. π’ | Server Load: Increases server resource usage. β οΈ |
Data Storage: Can store more data compared to cookies. π’ | Scalability Issues: Harder to scale across multiple servers. π΄ |
Automatic Expiry: Sessions typically expire after a set time. π’ | Complexity: More complex to manage compared to cookies. β οΈ |
Pros and Cons of Tokens
Tokens are used for authentication and authorization, often in modern web applications employing RESTful APIs or microservices architecture.
Pros | Cons |
Stateless: Tokens do not require server-side storage. π’ | Token Theft: If intercepted, tokens can be misused. π΄ |
Scalability: Easily scales across distributed systems. π’ | Complexity: Requires more setup and management. β οΈ |
Flexibility: Can be used across different domains and services. π’ | Expiry Management: Tokens must be refreshed periodically. β οΈ |
Quick Comparison Table
To summarize the differences and similarities between cookies, sessions, and tokens, refer to the table below:
Criterion | Cookies πͺ | Sessions π | Tokens π« |
Storage Location | Stored in the user’s browser | Stored on the server | Stored in the client-side application |
Lifespan | Can be set to expire at a specific time or last as long as the browser session | Lasts until the user logs out or the session times out | Typically has a short lifespan and requires renewal |
Security | Vulnerable to cross-site scripting (XSS) attacks | More secure as they are stored server-side | Relies on secure storage and transmission methods |
Use Cases | Commonly used for storing user preferences and tracking | Used for maintaining a logged-in state | Ideal for stateless authentication |
Example | Storing a shopping cart in an e-commerce site | Maintaining a user’s login session on a website | Authenticating API requests in a single-page application |
By understanding these mechanisms, developers can make informed decisions about which method to use based on their specific needs for web application security and state management.
Choosing the Right Solution: Practical Use Cases
Each methodβcookies, sessions, and tokensβhas its unique advantages and ideal use cases.
Cookies are a preferred choice for storing small pieces of data that need to persist between sessions. For example, cookies can effectively store user preferences, such as language settings or theme choices, which enhance the user experience by remembering these preferences on subsequent visits. Since cookies are stored on the client-side, they allow for quick access and do not burden the server with additional state management responsibilities.
Sessions, on the other hand, are optimal for temporary data storage that needs to be tied to a user’s interaction with the server. A classic example is an online shopping cart. When a user adds items to their cart, this information is stored in a session on the server. This approach ensures that the cart data remains intact as the user navigates through different pages, without exposing sensitive data to the client-side. Sessions are also advantageous in scenarios where data integrity and security are paramount, as they reduce the risk of client-side manipulation.
Tokens are increasingly popular in modern web applications, especially for secure API authentication and authorization. Tokens, such as JSON Web Tokens (JWT), are compact, self-contained, and can be easily transmitted through HTTP headers, making them ideal for Single Page Applications (SPAs) and mobile apps. For instance, when a user logs into a web application, the server generates and sends a token that the client can use to authenticate subsequent requests. This stateless approach simplifies server-side management and scales efficiently with the number of users.
FAQS
How are cookies and sessions different? π
Cookies: Stored on your browser and can persist even after you close it.
Sessions: Stored on the server and typically end when you close the browser or log out.
Are cookies safe? π‘οΈ
Cookies are generally safe but can be used for tracking your online activity. Websites should only use them responsibly and with your consent.
How can I clear my cookies? π§Ή
You can clear cookies from your browser settings. Look for options like “Clear browsing data” or “Delete cookies.”
What happens if I disable cookies? π«πͺ
If you disable cookies, some websites might not work correctly. You might have to log in every time or lose your preferences.
What is a session ID? π
A session ID is a unique identifier given to each session. It helps the server know which session belongs to which user.
How do tokens work? π οΈ
Tokens are created when you log in and sent with each request to the server. The server verifies the token to check if you’re allowed to access a resource.
Can tokens expire? β³
Yes, tokens usually have an expiration time for security reasons. After they expire, you need to log in again to get a new token.
What’s the difference between a session token and a JWT? π€
Session Token: Stored on the server, typically used for web sessions.
JWT (JSON Web Token): Encoded and signed token that can be stored on the client side and used across different services.
Why do websites use cookies and sessions? π
Websites use cookies and sessions to enhance user experience, remember login states, track usage, and maintain security.
Can I see what cookies a site is using? π
Yes, you can usually see the cookies a site is using from your browser settings or developer tools.
What is CSRF and how do tokens help? π‘οΈ
CSRF (Cross-Site Request Forgery) is an attack where malicious websites trick your browser into making unauthorized requests. Tokens help prevent this by ensuring that each request is legitimate.
How do I manage my cookies and sessions? π
You can manage cookies and sessions from your browser settings, where you can view, delete, and block them.