In today’s fast-paced digital world, users demand lightning-fast website experiences. A few seconds delay can make or break user engagement, affect bounce rates, and even impact search engine rankings. But how can you balance high-quality content with rapid loading times? The answer lies in a powerful web performance technique called lazy loading.
Imagine visiting an online store with high-resolution images of each product. Without lazy loading, the browser would load all images at once, even those not visible on the screen, causing slow loading times and frustrating users. But with lazy loading, images load only when they are about to enter the viewport, making the browsing experience seamless.
Lets explore lazy loading from the ground up—what it is, why it matters, and how to implement it effectively. Along the way, we’ll share examples and best practices to help you master this essential technique.
On This Page
Table of Contents
What is Lazy Loading?
Lazy loading is a design pattern that defers the loading of non-essential resources until they are actually needed. Instead of loading all images, videos, or scripts upfront, lazy loading loads them only when they enter the viewport. This approach optimizes page load speed, reduces bandwidth usage, and improves user experience.
How Lazy Loading Works
Lazy loading leverages the browser’s ability to detect the user’s scroll position. When a user scrolls down the page and a lazily loaded resource approaches the viewport, the browser fetches and displays it.
- Traditional Loading: Loads all resources upfront, increasing initial load time.
- Lazy Loading: Loads only critical resources first; defers non-visible content.
Example Scenario:
Imagine you are reading an online article with ten embedded YouTube videos. Without lazy loading, all videos would be requested simultaneously, increasing load time. With lazy loading, videos load only when they are about to enter the viewport, reducing initial load time.
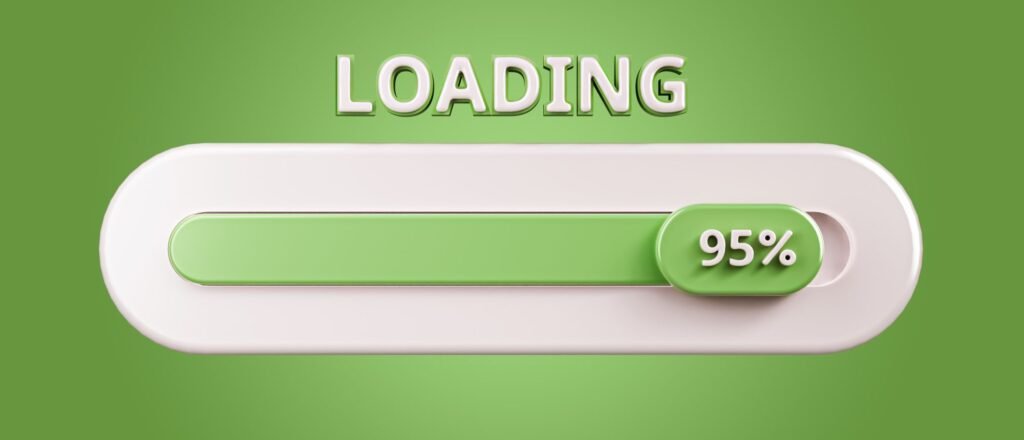
Benefits of Lazy Loading
Implementing lazy loading provides numerous advantages:
- Improved Page Load Speed:
- Critical content loads faster, reducing perceived load time.
- Non-visible resources are loaded later, minimizing initial requests.
- Enhanced User Experience:
- Users interact with the page sooner, leading to better engagement.
- Smooth scrolling experience without lag caused by heavy resources.
- Reduced Bandwidth and Resource Usage:
- Only necessary resources are loaded, conserving user data.
- Especially beneficial for mobile users on limited data plans.
- SEO Advantages:
- Faster loading pages are favored by search engines.
- Improved Core Web Vitals metrics (e.g., Largest Contentful Paint).
Real-Life Example:
When YouTube implemented lazy loading for embedded videos, it improved page load speed by 40%. This change reduced the number of initial requests and delayed loading of the player until the user interacted with the video thumbnail.
Types of Lazy Loading
Different types of content can benefit from lazy loading. Let’s explore them in detail:
Type of Lazy Loading | Description | Example Scenario |
---|---|---|
Image Lazy Loading | Loads images only when they are about to appear on screen. | Online store with product images. |
Video and Iframe Lazy Loading | Defers loading of videos and embedded content until needed. | Blog posts with YouTube embeds. |
JavaScript and CSS Lazy Loading | Loads non-critical scripts and stylesheets on demand. | Loading analytics scripts after page load. |
How to Implement Lazy Loading
There are multiple ways to implement lazy loading depending on the complexity and requirements of your website. Here are the most popular methods:
1. Using Native HTML loading
Attribute
The easiest way to implement lazy loading for images and iframes is by using the native loading
attribute. Supported by modern browsers, it is both lightweight and efficient.
<img src="example.jpg" alt="Example Image" loading="lazy">
<iframe src="https://www.cloudcusp.com/embed/xyz" loading="lazy"></iframe>
- Advantages:
- Minimal code changes.
- Native browser support with optimal performance.
- Limitations:
- Limited to images and iframes.
- Older browsers may not support it.
2. Using JavaScript Libraries and Plugins
For advanced lazy loading scenarios, JavaScript libraries provide more control and compatibility. Popular libraries include:
- LazyLoad.js: Lightweight library supporting images, videos, and iframes.
- lozad.js: High-performance lazy loader using the Intersection Observer API.
Example using lozad.js:
const observer = lozad('.lozad', {
rootMargin: '0px 0px 50px 0px',
threshold: 0.1
});
observer.observe();
<img class="lozad" data-src="example.jpg" alt="Example Image">
3. Using Intersection Observer API
For custom implementations, the Intersection Observer API is a powerful and flexible option. It detects when elements enter the viewport and triggers a callback to load them.
const images = document.querySelectorAll('.lazyload');
const observer = new IntersectionObserver((entries, observer) => {
entries.forEach(entry => {
if (entry.isIntersecting) {
const img = entry.target;
img.src = img.dataset.src;
observer.unobserve(img);
}
});
});
images.forEach(img => observer.observe(img));
- Advantages:
- Full control over lazy loading behavior.
- Better performance with minimal reflows.
- Limitations:
- Requires custom implementation.
- Not supported in older browsers.
Best Practices for Lazy Loading
To maximize lazy loading benefits, consider these best practices:
- Prioritize Above-the-Fold Content:
- Ensure that visible content loads first for a faster first paint.
- Lazy load only below-the-fold images and videos.
- Fallbacks for Browser Compatibility:
- Provide fallback solutions for older browsers.
- Use JavaScript polyfills if necessary.
- Handling SEO and Accessibility:
- Include descriptive
alt
tags for lazy-loaded images. - Use semantic markup to ensure SEO bots can index the content.
- Include descriptive
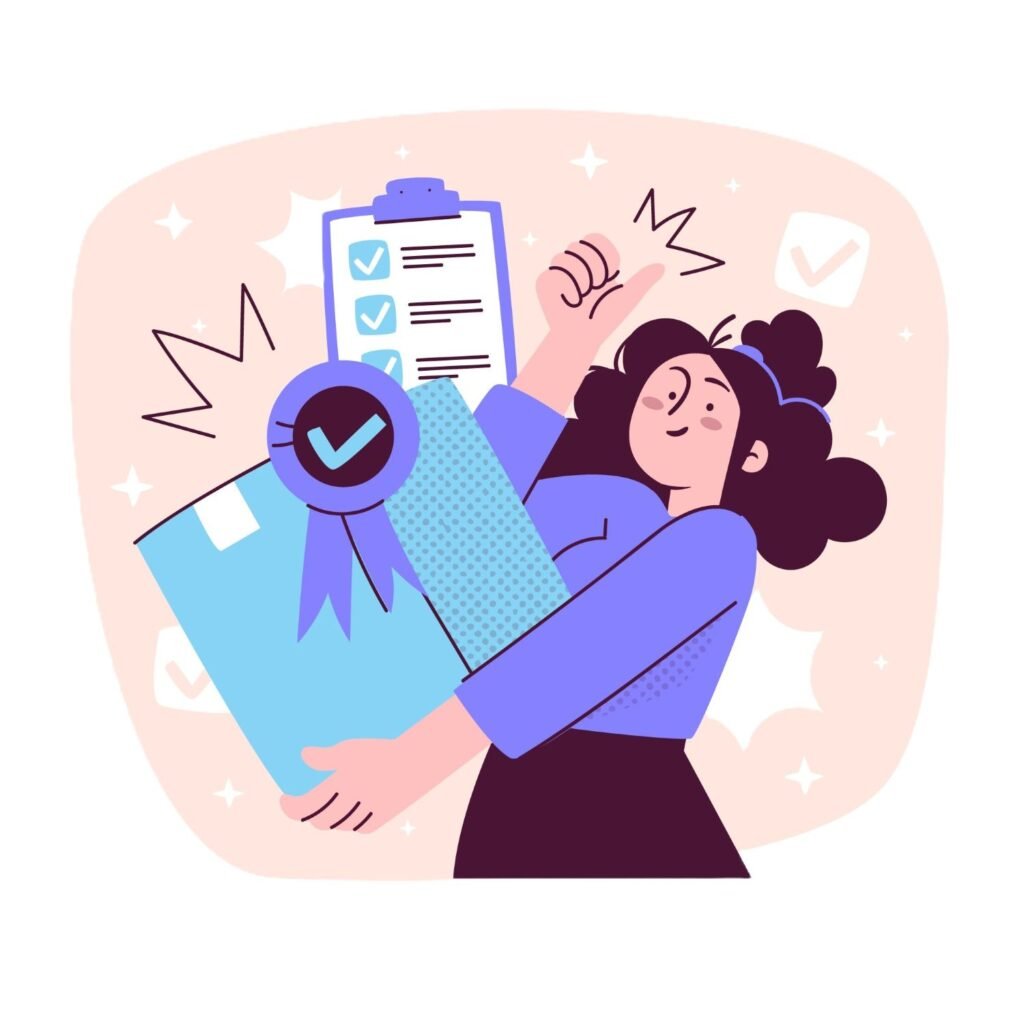
Common Pitfalls and How to Avoid Them
While lazy loading improves performance, incorrect implementation can lead to:
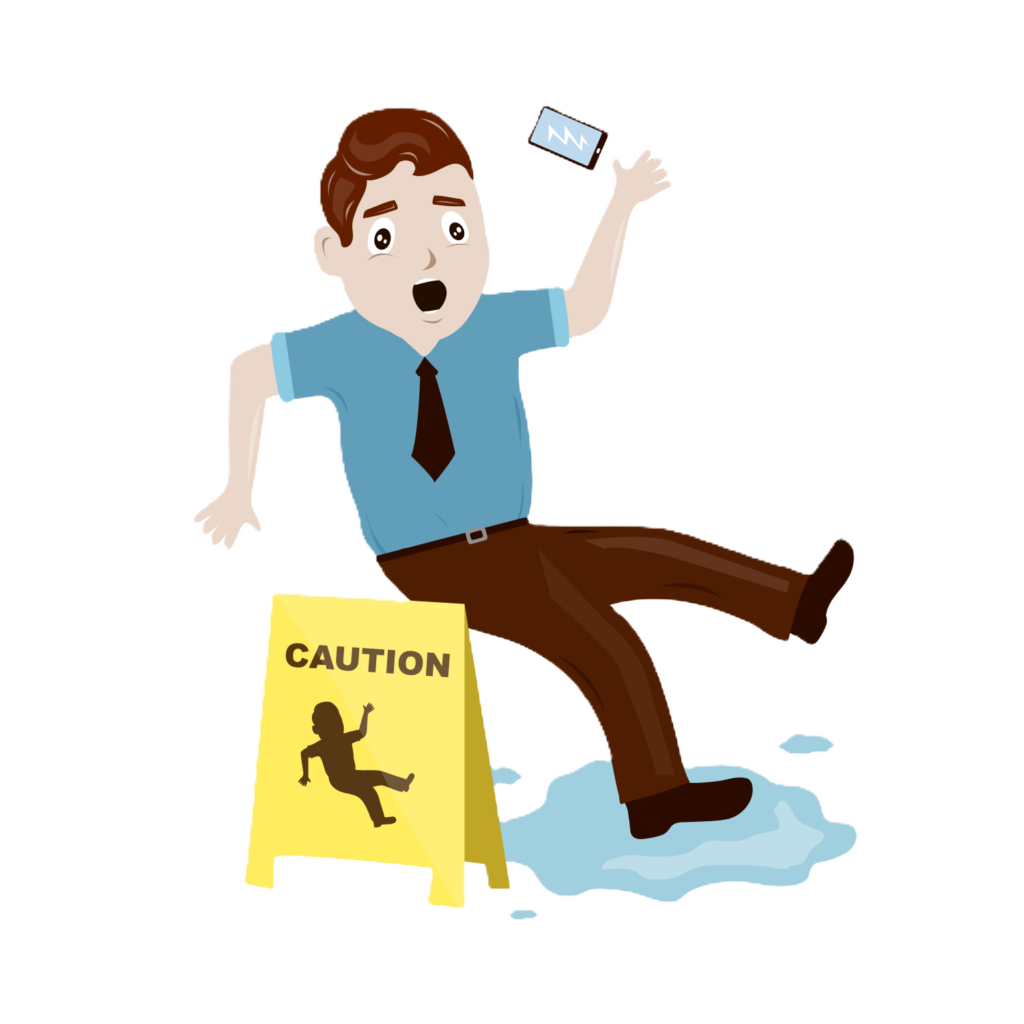
- Delayed Content Rendering:
- Lazy loading above-the-fold content can cause delays.
- Solution: Only lazy load non-essential elements.
- SEO Indexing Issues:
- Search engines may not index lazy-loaded content.
- Solution: Use server-side rendering or hybrid loading.
- Performance Overhead with JavaScript:
- Heavy libraries may counteract performance gains.
- Solution: Opt for lightweight libraries or native solutions.
Real-World Examples and Case Studies
Several companies have witnessed significant performance gains using lazy loading:
- Pinterest: Improved loading time by 40% by lazy loading images.
- Forbes: Reduced page load time by 50% using Intersection Observer for ads.
- Unsplash: Seamlessly loads high-resolution images using lazy loading, enhancing user experience.
WrapUP
Lazy loading is an indispensable technique for optimizing web performance. By strategically deferring non-essential resources, it ensures that users experience fast, smooth interactions without unnecessary waiting times. Whether you choose native attributes, JavaScript libraries, or custom Intersection Observer implementations, lazy loading can significantly enhance your website’s speed and user engagement.
Incorporate lazy loading into your development workflow today and watch your website’s performance and SEO metrics soar. As digital experiences evolve, mastering lazy loading will keep you ahead of the curve, ensuring users return for more.
FAQs
What is lazy loading?
Lazy loading is a web performance technique that defers the loading of non-critical resources (e.g., images, videos, scripts) until they are needed. It enhances page load speed by only loading content when it enters the user’s viewport.
Why should I use lazy loading?
Lazy loading improves page load speed, reduces bandwidth usage, and enhances user experience by loading only visible content first. It also positively impacts SEO as faster websites are favored by search engines.
Which types of content can be lazy-loaded?
Commonly lazy-loaded content includes:
Images (e.g., product photos, gallery images)
Videos and iframes (e.g., YouTube embeds)
Scripts and stylesheets (non-critical JavaScript and CSS)
How do I implement lazy loading for images?
The easiest way is to use the native HTML attribute:
<img src="example.jpg" alt="Example" loading="lazy">
This method is supported by modern browsers and requires no additional JavaScript.
What are the common methods to implement lazy loading?
Native loading
attribute: Lightweight and easy to implement for images and iframes.
JavaScript libraries: Tools like LazyLoad.js or lozad.js for more advanced use cases.
Intersection Observer API: Custom implementation for precise control and better perfor
Does lazy loading affect SEO?
When implemented correctly, lazy loading does not negatively impact SEO. However, ensure that critical content is loaded initially and that all images have descriptive alt
tags for accessibility and indexing.
Is lazy loading supported by all browsers?
Native lazy loading is supported by most modern browsers, including Chrome, Firefox, and Edge. However, older browsers may not support it. Consider using polyfills or JavaScript libraries for broader compatibility.
Can lazy loading cause content to appear late?
Yes, if not implemented carefully. Lazy loading above-the-fold content can cause delays. Always prioritize loading visible content first and defer only non-essential resources.
Does lazy loading improve website performance?
Absolutely! Lazy loading significantly reduces initial page load time, saves bandwidth, and improves scrolling performance, leading to better user engagement and SEO benefits.
Are there any disadvantages of lazy loading?
SEO Challenges: Improper implementation may prevent search engines from indexing content.
Compatibility Issues: Not supported by all browsers, requiring fallbacks.
Delayed User Experience: Content may appear late if network latency is high.
Can I lazy load videos and iframes?
Yes! You can use the native loading="lazy"
attribute for iframes. For videos, use data-src
attributes combined with JavaScript to load them dynamically.
Example for iframe:
<iframe src="https://www.cloudcusp.com/embed/xyz" loading="lazy"></iframe>
Should I lazy load all images on my website?
No, avoid lazy loading images above the fold (e.g., hero banners) as this can delay content visibility. Only lazy load images below the fold to optimize load times effectively.
What are some popular lazy loading libraries?
LazyLoad.js – Lightweight and easy to integrate.
lozad.js – High performance, uses Intersection Observer API.
LazySizes – Flexible with support for responsive images.
How can I test if lazy loading is working?
Use browser developer tools:
Inspect network requests to see when resources are loaded.
Scroll the page and check if images or videos load on demand.
Tools like Lighthouse or GTmetrix can also analyze lazy loading performance.
Can I use lazy loading with a CDN?
Yes, lazy loading is compatible with Content Delivery Networks (CDNs). In fact, CDNs can further enhance performance by caching and delivering lazily loaded resources faster.
How do I debug lazy loading issues?
Check the browser console for JavaScript errors.
Ensure data-src
attributes are correctly set.
Verify Intersection Observer configurations.
Use tools like Lighthouse or WebPageTest for performance audits.
What are the best practices for lazy loading?
Prioritize above-the-fold content to avoid delays.
Use responsive images to optimize for different devices.
Implement SEO-friendly markup with descriptive alt
tags.
Combine lazy loading with CDN for enhanced performance.
Can lazy loading be combined with other performance optimizations?
Yes! Combine lazy loading with:
Image optimization (e.g., WebP format)
Code minification (JavaScript and CSS)
Caching strategies for faster loading
Content Delivery Networks (CDNs) for global resource delivery