John, a software developer, was frustrated. Every time he made a small change to his code, something else broke. Debugging took hours, and last-minute fixes before project deadlines gave him nightmares. One day, a senior developer introduced him to Test-Driven Development (TDD), a technique that seemed counterintuitive at first: “Write tests before writing the actual code? That sounds backward!” However, as John implemented TDD in his workflow, he saw a significant improvement in his code quality and confidence. This is his journey into the world of TDD.
On This Page
Table of Contents
What is Test-Driven Development (TDD)?
TDD is a software development methodology where developers write test cases before writing the actual code. It follows a repetitive cycle of writing a failing test, implementing the minimal code required to pass the test, and then refactoring the code for optimization.
Key Principles of TDD
- Write Tests First: Define the expected functionality before coding.
- Fail First: The initial test should fail because the implementation doesn’t exist yet.
- Incremental Development: Write just enough code to pass the test, then refactor.
- Automation: Tests run automatically to validate new changes.
Why is TDD Important?
- Helps in detecting bugs early.
- Encourages better software design.
- Reduces debugging time.
- Increases developer confidence in making changes.
How Test-Driven Development Works
TDD follows a structured approach called the Red-Green-Refactor cycle:
Phase | Description |
---|---|
Red | Write a test for the new feature. Since the feature is not implemented, the test will fail. |
Green | Write the minimum amount of code needed to make the test pass. |
Refactor | Improve the code structure while ensuring tests still pass. |
Step-by-Step Example of TDD
Imagine John is building a simple function in Python that adds two numbers.
Step 1: Write a Failing Test (Red Phase)
import unittest
from calculator import add
class TestCalculator(unittest.TestCase):
def test_add(self):
self.assertEqual(add(2, 3), 5)
self.assertEqual(add(-1, 1), 0)
- John writes a test expecting
add(2, 3)
to return5
. - Since
add()
isn’t defined yet, running this test will fail.
Step 2: Write Minimal Code to Pass the Test (Green Phase)
def add(a, b):
return a + b
- John implements a simple function that returns
a + b
. - Running the test now passes.
Step 3: Refactor Code (Refactor Phase)
- Since the function is already optimal, no major refactoring is needed.
- However, John ensures code readability and maintainability.
Key Benefits of TDD
Benefit | Explanation |
---|---|
Higher Code Quality | Forces developers to think about the functionality before coding. |
Early Bug Detection | Bugs are caught at the development stage, reducing debugging time. |
Improved Code Design | Encourages writing modular and reusable code. |
Faster Development in the Long Run | Reduces time spent on fixing issues later in the project. |
Increases Developer Confidence | Changes can be made without fear of breaking existing features. |
Challenges and Limitations of TDD
- Initial Learning Curve: Developers new to TDD may find it time-consuming.
- Slower Development in the Beginning: Writing tests first requires more time initially.
- Not Suitable for All Projects: Simple scripts or exploratory coding might not need TDD.
- Requires Discipline: Developers must strictly follow the Red-Green-Refactor cycle.
TDD vs. Traditional Development
Feature | TDD | Traditional Development |
---|---|---|
Testing Approach | Tests are written first | Tests are written after coding |
Error Detection | Early detection | Later in the cycle or post-production |
Code Structure | Encourages modularity | Can become complex and messy |
Development Speed | Slower initially, faster long-term | Faster initially, but debugging takes time |
Best Practices for Effective TDD
- Write Small, Focused Tests: Keep test cases short and focused on one behavior.
- Keep Tests Independent: Each test should work in isolation.
- Use Meaningful Assertions: Ensure the expected output is clearly defined.
- Refactor Code Without Changing Behavior: Maintain functionality while improving structure.
- Automate Tests: Integrate testing into CI/CD pipelines for continuous validation.
TDD in Different Programming Languages
TDD can be implemented in various languages using different testing frameworks.
Language | Testing Framework |
---|---|
Python | unittest , pytest |
Java | JUnit |
JavaScript | Jest , Mocha |
C# | NUnit , xUnit |
Ruby | RSpec |
Example in JavaScript (Using Jest)
Step 1: Write a Failing Test
const add = require('./calculator');
test('adds 2 + 3 to equal 5', () => {
expect(add(2, 3)).toBe(5);
});
Step 2: Implement Function
function add(a, b) {
return a + b;
}
module.exports = add;
Step 3: Run Tests
npm test
If the test passes, the function works as expected.
WrapUP: Why TDD is a Game Changer
John’s initial doubts about TDD vanished as he saw the results. His code was more structured, bug-free, and maintainable. The fear of breaking something was gone because every change was backed by tests. TDD may require discipline, but the long-term benefits outweigh the initial investment. If you’re a developer, adopting TDD could be the key to writing robust and scalable software.
If you haven’t tried Test-Driven Development, give it a shot! Start with a simple function, follow the Red-Green-Refactor cycle, and witness the magic of writing tests first!
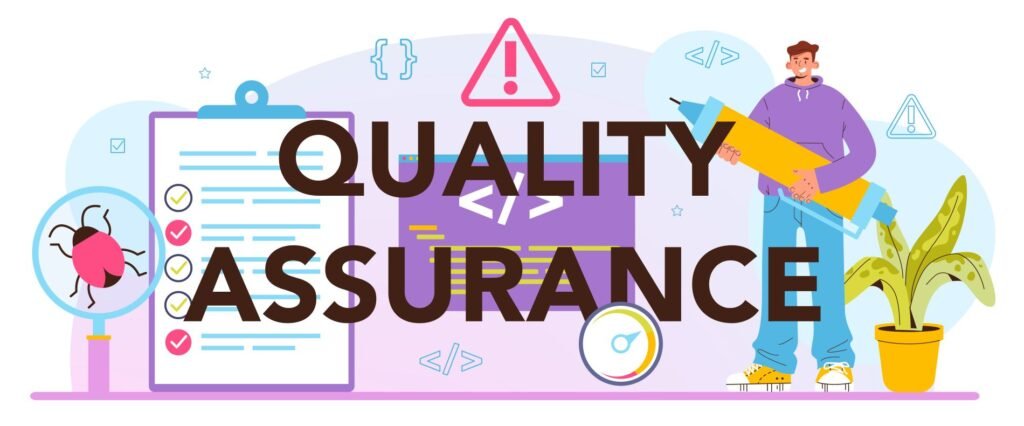
FAQs
What is Test-Driven Development (TDD)?
TDD is a software development approach where tests are written before the actual code. Developers follow a Red-Green-Refactor cycle to ensure the code meets requirements before implementation.
What are the key benefits of TDD?
Improves code quality and design.
Helps catch bugs early in development.
Reduces time spent on debugging and maintenance.
Increases developer confidence in making changes.
What is the Red-Green-Refactor cycle in TDD?
Red: Write a failing test for new functionality.
Green: Write the minimum code required to pass the test.
Refactor: Optimize the code while ensuring tests still pass.
Does TDD slow down development?
Initially, TDD may take more time due to writing tests first, but in the long run, it saves time by reducing debugging and improving maintainability.
Can TDD be used for all types of projects?
TDD is ideal for projects requiring high reliability (e.g., web apps, APIs, large software systems). However, for simple scripts or exploratory coding, TDD might not be necessary.
Which programming languages support TDD?
TDD can be implemented in almost all languages. Some popular testing frameworks include:
Python → unittest
, pytest
Java → JUnit
JavaScript → Jest
, Mocha
C# → NUnit
, xUnit
Can TDD be combined with Agile development?
Yes, TDD aligns well with Agile methodologies, ensuring continuous integration, frequent testing, and delivering small, functional increments.
How do I start learning TDD?
Pick a simple function to test (e.g., an addition function).
Follow the Red-Green-Refactor cycle.
Use a testing framework for your preferred language.
Gradually apply TDD in larger projects.
What are common mistakes developers make when implementing TDD?
Writing too many tests without focusing on core functionality.
Skipping the Refactor phase, leading to unoptimized code.
Writing large, complex tests instead of small, focused ones.
Ignoring test failures and forcing tests to pass incorrectly.
Can I use TDD for frontend development?
Yes! TDD can be applied to frontend development using frameworks like:
React → Jest
, React Testing Library
Angular → Jasmine
, Karma
Vue.js → Vue Test Utils
Is TDD only for professional developers?
No! Both beginners and experienced developers can benefit from TDD. It helps build good coding habits and ensures robust, bug-free applications.