Python has become the dominant language for AI and machine learning due to its extensive ecosystem of libraries and frameworks. Whether you are working on deep learning, natural language processing, or reinforcement learning, Python offers a vast range of tools to help you develop powerful AI models efficiently.
In this page, we will explore some of the best AI and machine learning libraries in Python and how to use them.
On This Page
Table of Contents
Machine Learning Libraries
Scikit-Learn: The Classic ML Library
Scikit-Learn is one of the most popular and easy-to-use machine learning libraries for classical ML algorithms.
Key Features:
- Implements regression, classification, clustering, and dimensionality reduction
- Built-in datasets for testing models
- Strong integration with NumPy and SciPy
Example: Logistic Regression with Scikit-Learn
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.datasets import load_iris
# Load dataset
iris = load_iris()
X_train, X_test, y_train, y_test = train_test_split(iris.data, iris.target, test_size=0.2)
# Train model
model = LogisticRegression()
model.fit(X_train, y_train)
# Predict
predictions = model.predict(X_test)
print(predictions)
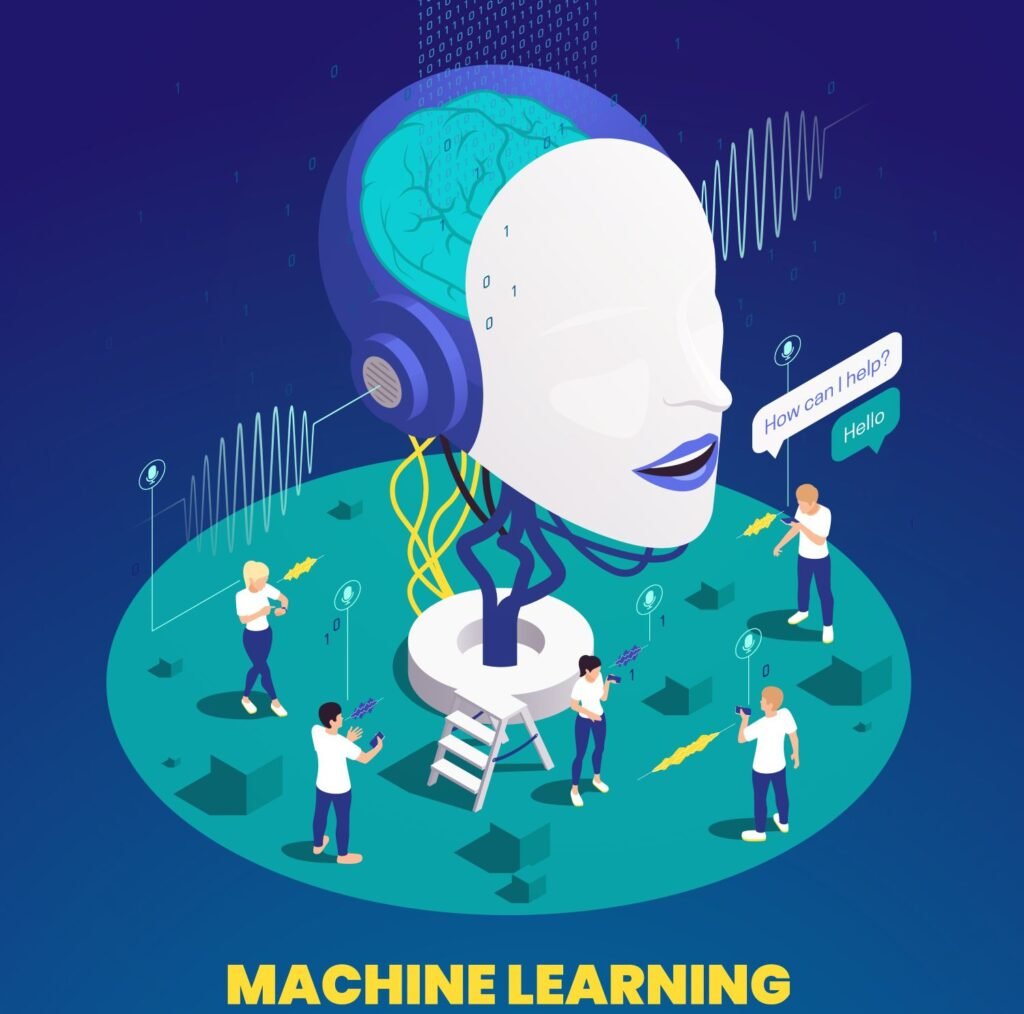
XGBoost: Extreme Gradient Boosting
XGBoost is a powerful library for boosting-based models.
Key Features:
- Parallel processing support
- Optimized gradient boosting algorithm
- Handles missing values efficiently
Example: Training an XGBoost Classifier
import xgboost as xgb
from sklearn.datasets import load_breast_cancer
from sklearn.model_selection import train_test_split
# Load data
cancer = load_breast_cancer()
X_train, X_test, y_train, y_test = train_test_split(cancer.data, cancer.target, test_size=0.2)
# Train model
model = xgb.XGBClassifier()
model.fit(X_train, y_train)
# Predict
predictions = model.predict(X_test)
print(predictions)
Natural Language Processing (NLP) Libraries
NLTK: The Classic NLP Library
NLTK is a comprehensive toolkit for NLP research and education.
Key Features:
- Tokenization, stemming, and lemmatization
- Named Entity Recognition (NER)
- Sentiment analysis and text classification
Example: Tokenization and POS Tagging with NLTK
import nltk
from nltk.tokenize import word_tokenize
from nltk import pos_tag
nltk.download('punkt')
nltk.download('averaged_perceptron_tagger')
text = "AI is transforming industries worldwide."
tokens = word_tokenize(text)
print("Tokens:", tokens)
print("POS Tags:", pos_tag(tokens))
spaCy: Fast & Efficient NLP
spaCy is designed for production-ready NLP applications.
Key Features:
- Pre-trained models for multiple languages
- Named Entity Recognition (NER) and dependency parsing
- Works well with deep learning frameworks
Example: Named Entity Recognition (NER) with spaCy
import spacy
nlp = spacy.load("en_core_web_sm")
doc = nlp("Elon Musk founded SpaceX in 2002.")
for ent in doc.ents:
print(ent.text, ent.label_)
Deep Learning Libraries
TensorFlow
TensorFlow is a leading deep learning framework developed by Google.
Key Features:
- Supports neural networks and deep learning models
- Works with CPUs and GPUs
- TensorBoard for visualization
Example: Training a Simple Neural Network
import tensorflow as tf
# Create a simple model
model = tf.keras.Sequential([
tf.keras.layers.Dense(64, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
# Compile model
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
print(model.summary())
PyTorch
PyTorch is an open-source deep learning framework developed by Facebook.
Key Features:
- Dynamic computation graphs
- Strong GPU acceleration
- Used for research and production
Example: Training a Neural Network with PyTorch
import torch
import torch.nn as nn
class SimpleNN(nn.Module):
def __init__(self):
super(SimpleNN, self).__init__()
self.fc1 = nn.Linear(10, 5)
self.fc2 = nn.Linear(5, 1)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = torch.sigmoid(self.fc2(x))
return x
model = SimpleNN()
print(model)
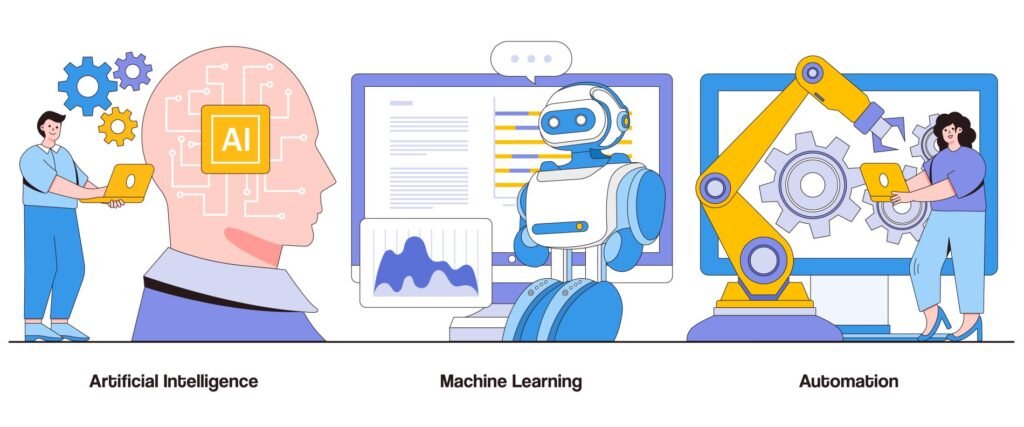
Reinforcement Learning (RL) Libraries
OpenAI Gym
OpenAI Gym is a standard environment for developing and testing reinforcement learning algorithms.
Key Features:
- Standardized RL environments
- Works with TensorFlow and PyTorch
- Supports Atari, robotics, and more
Example: Running an RL Environment
import gym
env = gym.make("CartPole-v1")
state = env.reset()
for _ in range(1000):
env.render()
action = env.action_space.sample()
state, reward, done, _ = env.step(action)
if done:
break
env.close()
Automated Machine Learning (AutoML) Libraries
Auto-sklearn
Auto-sklearn automates model selection and hyperparameter tuning.
Key Features:
- Optimizes models automatically
- Handles preprocessing
- Uses ensemble learning
Example: Using AutoML for Classification
import autosklearn.classification
from sklearn.datasets import load_digits
from sklearn.model_selection import train_test_split
X, y = load_digits(return_X_y=True)
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
automl = autosklearn.classification.AutoSklearnClassifier()
automl.fit(X_train, y_train)
print(automl.score(X_test, y_test))
WrapUP
Python offers a vast ecosystem of AI and ML libraries catering to different needs. From classical ML with Scikit-Learn to cutting-edge deep learning with TensorFlow and PyTorch, the choice depends on your specific use case. Whether you’re a beginner or an advanced AI practitioner, these libraries will help you build powerful AI applications efficiently.
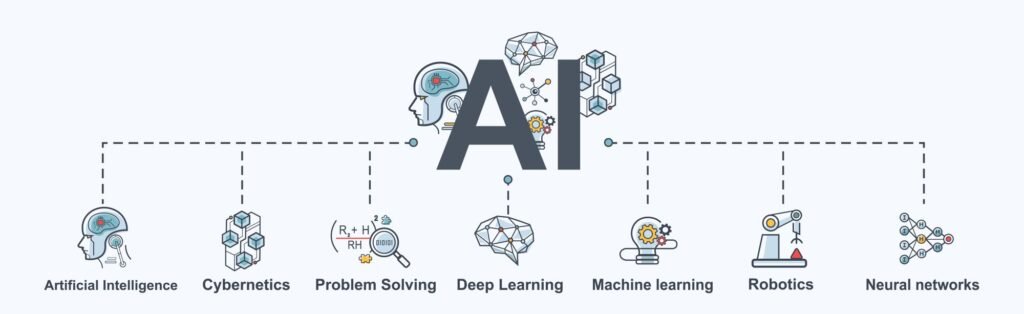
FAQs
What are the most important Python libraries for AI engineers?
The most essential Python libraries for AI engineers include:
Machine Learning: Scikit-Learn, XGBoost, LightGBM
Deep Learning: TensorFlow, PyTorch
Natural Language Processing (NLP): spaCy, NLTK, Transformers
Computer Vision (CV): OpenCV, PIL, TensorFlow/Keras for image processing
Reinforcement Learning: Stable-Baselines3, Gym
AutoML: Auto-sklearn, H2O.ai
Why is Python the preferred language for AI and Machine Learning?
Python is preferred due to:
Extensive Libraries & Frameworks (e.g., TensorFlow, PyTorch)
Easy-to-Read Syntax (enhances productivity)
Strong Community Support
Integration with Other Languages (C, C++, Java)
Scalability & Flexibility
Which Python library is best for building chatbots?
For chatbot development, you can use:
spaCy or NLTK for text processing
Transformers (by Hugging Face) for advanced NLP
Rasa for conversational AI
TensorFlow/Keras for deep learning models
How does Scikit-Learn differ from TensorFlow?
Scikit-Learn is used for traditional machine learning (e.g., decision trees, logistic regression), whereas TensorFlow is used for deep learning (e.g., neural networks).
What is XGBoost used for?
XGBoost is a gradient boosting algorithm that excels in structured/tabular data tasks, such as:
— Predicting customer churn
— Fraud detection
— House price prediction
How can I use OpenCV for AI applications?
OpenCV is great for image and video processing. Example: Face detection
What is AutoML, and which Python libraries support it?
AutoML automates the process of building ML models. Popular Python libraries for AutoML:
— Auto-sklearn (automated ML pipeline)
— H2O.ai (scalable ML automation)
— TPOT (genetic algorithms for ML selection)
What is the best Python library for reinforcement learning?
Stable-Baselines3 is widely used for RL. It provides implementations of algorithms like DQN, PPO, A2C.
What are some key use cases for NLP libraries?
NLP libraries help with:
Sentiment analysis (e.g., classifying positive/negative reviews)
Named Entity Recognition (NER) (e.g., extracting people’s names from text)
Chatbot development (e.g., automating customer service)
Text summarization (e.g., summarizing news articles)
How do AI engineers optimize ML model performance?
To improve model performance:
— Use feature engineering
— Tune hyperparameters (e.g., GridSearchCV for Scikit-Learn)
— Use ensemble learning (e.g., XGBoost, Random Forest)
— Reduce overfitting with regularization
What are some challenges AI engineers face when using Python libraries?
Model Interpretability: Hard to understand why a model made a decision
Computational Costs: Training deep learning models can be expensive
Data Quality Issues: Poor data leads to biased models
Library Compatibility: Some libraries don’t work well together
Which Python library is best for AI beginners?
For beginners, the best libraries are:
Scikit-Learn (easy ML models)
TensorFlow/Keras (simple deep learning)
spaCy (user-friendly NLP)
OpenCV (basic image processing)